Introduction
This trick shows how you can use ImageButton
control as AdRotator
without using xml or database integration to rotate the picture of the advertisement.
Background
Using AdRotator
control we need to create xml file or database with ImageUrl, NavigateUrl, AlternateText, Impression tags/fields ,instead we can directly use ImageButton
control as Adrotator
to rotate advertisements and navigate the user to specified page once he/she clicks on ImageButton.
Using the code
- Create new website project in visual studio by selecting ASP.NET empty website template.
- Create Images folder in the project and store some images in the folder and rename each one of them as follows
If we want to display the shopping website image in ImageButton then rename that image as websitename.jpg
For example-If we want to display image which shows clothings available at filpkart.com then rename that image as flipkart.jpg
- Now Add new webform to the project and name it as
Default.aspx
and in form
tag write the following code
<asp:ScriptManager ID="sm1" runat="server"></asp:ScriptManager>
<div align="center">
<asp:UpdatePanel runat="server">
<ContentTemplate>
<asp:ImageButton ID="img" Height="300" Width="350" OnClientClick="return confirm('Are you sure, you want to leave the page?');" OnClick="img_Click" runat="server" />
</ContentTemplate>
<Triggers>
<asp:AsyncPostBackTrigger ControlID="timer1" EventName="Tick" />
</Triggers>
</asp:UpdatePanel>
</div>
<asp:Timer ID="timer1" runat="server" OnTick="timer1_tick" Interval="2000"></asp:Timer>
In above source view ImageButton
control is enclosed in update panel
to avoid full postback of the page. Timer
is used to rotate the image after 2 seconds by using AsyncPostback
trigger of update panel
. - Now in code behind write the following code.
using System.IO;
public partial class Adrotatorwithimgtag : System.Web.UI.Page
{
static List<string> filename=new List<string> ();
static List<string> url = new List<string>();
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
DirectoryInfo info = new DirectoryInfo(Server.MapPath("~/images/"));
foreach (var item in info.GetFiles())
{
filename.Add(item.Name);
}
foreach (var item in info.GetFiles())
{
url.Add(item.Name.Substring(0,item.Name.Length-4));
}
}
}
protected void timer1_tick(object sender, EventArgs e)
{
Random r = new Random();
int i = r.Next(0, filename.Count);
img.ImageUrl = "~/images/" + filename[i];
ViewState["x"] = "http://www."+url[i]+".com";
img.ToolTip = "http://www." + url[i] + ".com";
}
protected void img_Click(object sender, ImageClickEventArgs e)
{
Response.Redirect(ViewState["x"].ToString(),true);
}
}
- We are using
DirectoryInfo
class of System.IO
namespace so import System.IO
namespace. To create object of DirectoryInfo
class provide path of the images folder as specified. - Using
foreach
loop and GetFiles()
method of DirectoryInfo
class we are storing complete name of the image file in filename
object and name of the image file without .jpg extension in url
object using Name
property of DirectoryInfo
class. - In
timer1_tick
event we are using Random
class to generate random number between 0 and total count of images which are present in images folder and assign that random number to int variable i
to access the data which are present in filename
and url
generics by using index.
- Run the project to check output as follows:
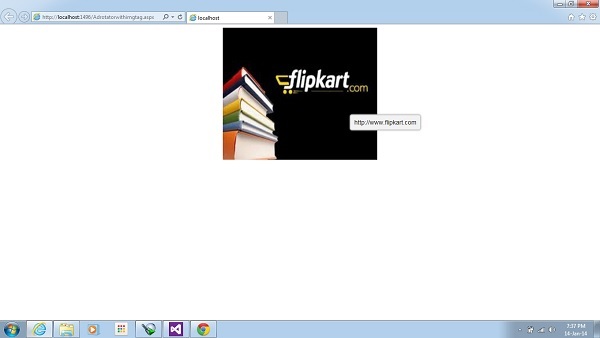
Points of Interest
Using ajax changing the behavior of ImageButton control and use it as Adrotator control.