Introduction
In a project, if you need to extract data from Excel, you have a lot of options irrespective of technology. But if you have a requirement to retrieve data from a MPP (Microsoft Project Plan) file, you have a limited number of options.
Firstly, you can use MPXJ (Microsoft Project Exchange) open source Library to retrieve data from MPP file. But the problem is that it is coded in Java and the .NET version just embeds a virtual Java processor and then calls the Java-native version. It involves performance overhead.
Secondly, you can use Interop library provided in .NET Using this library, you can access an MPP file but is very complex and is low-level. Programming directly against Interop has been an issue with developers.
We came up with a generalized library keeping in mind the structure and fields of a MPP file. It provides you a direct mapping with inbuilt fields of a MPP file in an exact manner. For example, tasks in a project may have subtasks, but when you directly read it using Interop, even subtasks will be considered as separate tasks. Then the essence of a MPP file gets lost and rather it looks like an Excel file.
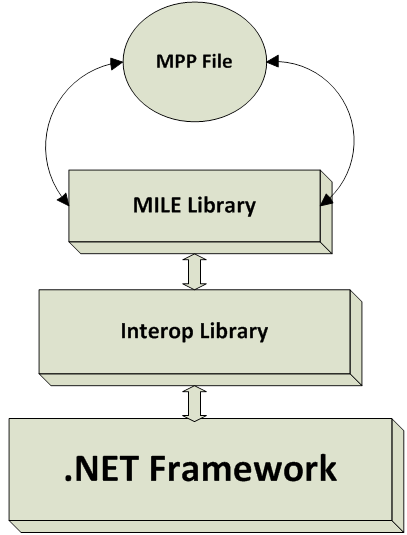
Fig 1.0 Structure of MILE
We have kept intact the extensibility of Interop library and allowed the user to access and create a MPP file in a generalized manner keeping the essence of a project file intact.

Fig 1.1 Class Specifications
Extensions
- Disconnected architecture
- LINQ extensibility
- Generate MPP file in a generalized manner
- Accessing data from project file in its essence
- Refinement- Child tasks
- Secure for web application
Prerequisites
Microsoft Project should be installed on your machine.
In order to use this library, you can use the following steps:
- Add reference of the provided DLL
- Import
Abhi.MILE
- Create a List object of type
MppTask
and a reference variable of type MPPProject
:
List<MppTask> lsMppTasks;
MPPProject project;
- Declare a
string
variable ‘path
’ to store the location of MPP file and store the path in it
String path;
path="Your path here";
- After storing the path, just instantiate an object of
MPPProject
class as per the class definition:
project = new MPPProject(path);
- Retrieve all tasks present in the mpp file through the object created in the last step.
Invoke
GetAllTasks
method and store all tasks present in a list variable created in
Step 3:
lsMppTasks = project.GetAllTasks();
- Now you have a project object that contains all Tasks, subtasks and resources present in the Mpp file. With the help of
List
variable, we can access subtasks and all other fields or properties of a particular task as shown below:
Firsttask.text=lsMpptasks[0].Name;
FirstChildOfFirstTask.text= lsMpptasks[0].ChildTasks[0].Name;
ChildTaskStartDate.Text = lsMpptasks [0].ChildTasks[0].StartDate.ToString();
SecondChildOfFirstTask.Text = lsMpptasks [0].ChildTasks[1].Name;
Now the lsMppTask
object can be used to access entire information present in MPP file with the help of methods as shown in the Class specification. In this way, library can be used for accessing all information present in a MPP file.
- Similarly, we can create a MPP File by creating
List
of MppTasks
and pass them into CreateMppFile
method of MppProject
class.
List<MppTask> lsMppTasks;
MppTask task1=new MppTask();
task1.Name="Task1";
task1.StartDate="30/09/2012";
task1.FinishDate="10/11/2012";
MppTask task2=new MppTask();
task2.Name="Task2";
task2.StartDate="12/09/2012";
task2.FinishDate="01/11/2012";
lsMppTasks.Add(task1);
lsMppTasks.Add(task2);
project .CreateMppFile(lsMppTasks,"D:/My_MppFile.mpp");
For all remaining properties and methods, kindly refer to the class specification given above.
Reference(s)