Introduction
This is a tutorial for developing a simple Web Browser App for a particular website in Android OS. To understand this tutorial, you will need to have some basic knowledge about programming in Java. The goal of this tutorial is to teach you how to make Loader Dialog Boxes and create Instances to load URL in your Android app.
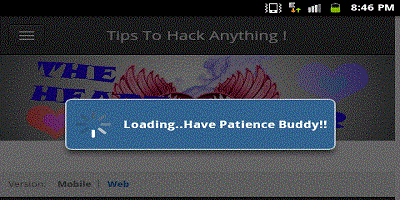
Background
To start developing your own Android apps, you need a SDK. I used Eclipse SDK.
Using the Code
Let's see what is in the code! I have pasted the full JAVA source code of MainActivity
here.
package com.androidexample.webview;
import android.app.Activity;
import android.app.ProgressDialog;
import android.os.Bundle;
import android.webkit.WebView;
import android.webkit.WebViewClient;
public class ShowWebView extends Activity {
private WebView webView;
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.show_web_view);
webView = (WebView) findViewById(R.id.webView1);
startWebView("http://www.hearthackertips.weebly.com");
}
private void startWebView(String url) {
webView.setWebViewClient(new WebViewClient() {
ProgressDialog progressDialog;
public boolean shouldOverrideUrlLoading(WebView view, String url) {
view.loadUrl(url);
return true;
}
public void onLoadResource (WebView view, String url) {
if (progressDialog == null) {
progressDialog = new ProgressDialog(ShowWebView.this);
progressDialog.setMessage("Loading..Have Patience Buddy!!");
progressDialog.show();
}
}
public void onPageFinished(WebView view, String url) {
try{
if (progressDialog.isShowing()) {
progressDialog.dismiss();
progressDialog = null;
}
}catch(Exception exception){
exception.printStackTrace();
}
}
});
webView.getSettings().setJavaScriptEnabled(true);
webView.loadUrl(url);
}
public void onBackPressed(WebView view, String url)
{
ProgressDialog progressDialog1;
if(webView.canGoBack())
{
webView.goBack();
}
else
{
super.onBackPressed();
}
}
}
I hope my code is self explanatory. I have written the purpose of most functions in the comments.
You can see that Android app developing isn't too hard. With some practice, you can develop your Android dream apps.
At the end of the tutorial, I would like to thank you for your attention. This is my first article and I would be really glad if you give me any feedback.
Points of Interest
- Previously some sites were unable to load. It was rectified by enabling JavaScript on webView.
- I didn't include any Exit Button. Instead I made a function to Respond to Back Button for step wise Exit from App.
- You can make mobile app for any site by replacing the URL in:
"startWebView("http:
History
- 15th April, 2014 - Article initiated