Introduction
My first article ever, in any community. A progress bar is something that definitely any developer will need at some point. I've read some other articles about the same topic here but found them unnecessarily complicated, both for beginners and also for the more experient. If you are a beginner, you don't need to understand the code right away. You only need to know how to instantiate a class and update its properties as your task progresses. If you are experient you'll understand the code (and maybe enhance it) but will not need to worry about fiddling with it (hopefully).
Background
Before any code, I think it's useful to list some characteristics.
A screenshot of the demo project:
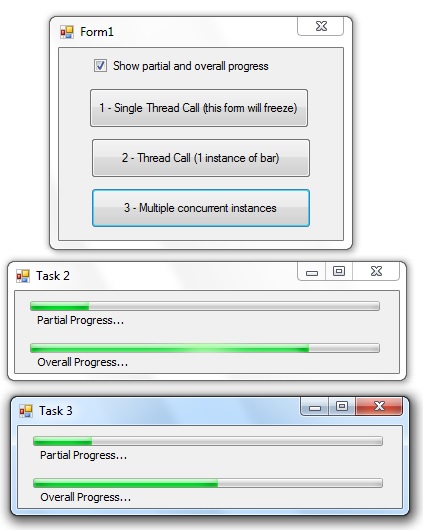
Using the code
The main class is too long to be shown here so instead of pasting it, it's better to describe its properties.
WindowTitle As String
OverallProgressValue As Int32 (1 to 100)
OverallProgressText As String (shown beneath the overall progress bar)
PartialProgressValue As Int32 (If omitted, the partial progress bar isn't shown)
PartialProgressText As String
CallerThreadSet As Threading.Thread
(WriteOnly, optional. The thread that called this class/thread. Use this if you want
the progress bar window to close itself when the caller thread ends)
TimeOut As Int32
(Optional, default = 60. Time out in seconds without receiving any progress updates. User is asked whether or not to close the window)
This class creates a form which will have a timer that updates the controls at every tick.
![Image 2]()
Using the Class
The example below from the demo project uses the class to show the progress of a 10-second loop.
The milliseconds update the partial bar.
Each second updates the overall bar.
Private Sub Task_2()
Dim pb As New Progress_Bar
pb.WindowTitle = "Task 2"
pb.TimeOut = 60
pb.CallerThreadSet = Threading.Thread.CurrentThread
Dim dt As Date = Now
Do While DateDiff(DateInterval.Second, dt, Now) < 10
If Me.CheckBox1.Checked = True Then
pb.PartialProgressText = "Partial Progress..."
If Now.Millisecond < 100 Then
pb.PartialProgressValue = 1
Else
pb.PartialProgressValue = ((Now.Millisecond / 999) * 100)
End If
pb.OverallProgressText = "Overall Progress..."
pb.OverallProgressValue = ((DateDiff(DateInterval.Second, dt, Now) / 10) * 100)
Else
pb.OverallProgressText = "Overall Progress..."
pb.OverallProgressValue = ((DateDiff(DateInterval.Second, dt, Now) / 10) * 100)
End If
Threading.Thread.Sleep(1)
Loop
If Me.CheckBox1.Checked = True Then pb.PartialProgressValue = 100
pb.OverallProgressValue = ((DateDiff(DateInterval.Second, dt, Now) / 10) * 100)
End Sub
Points of Interest
You may also find useful the elements of multi threading and control creation at runtime .