Introduction
as I said in previous tips , Pictures are the most used tools by developers , this time I will show you how to crop
image in different size to do a puzzle or to add a beautiful animation in the picture.
Using the code
The first thing you must do , is to download the WriteableBitmapExt here or you can download it from nuget on your visual studio , this dll allow you to use the Crop
To Crop Image , you must create a WriteableBitmap
image
WriteableBitmap image = new WriteableBitmap(1, 1);
image = await WriteableBitmapExtensions.FromContent(image, new Uri("ms-appx:///Assets/cat.jpg", UriKind.RelativeOrAbsolute));
Then you call the Function Cropper that takes two arguments , The image and the size :
public static List<WriteableBitmap> Cropper(WriteableBitmap <code>mainImage</code>, int <code>size</code>)
{
int num1 = <code>mainImage</code>.PixelWidth;
int x = Math.Abs((<code>mainImage</code>.PixelHeight - <code>mainImage</code>.PixelWidth) / 2);
int y = Math.Abs((<code>mainImage</code>.PixelHeight - <code>mainImage</code>.PixelWidth) / 2);
List<WriteableBitmap> list = new List<WriteableBitmap>();
if (<code>mainImage</code>.PixelWidth > <code>mainImage</code>.PixelHeight)
{
num1 = <code>mainImage</code>.PixelHeight;
y = 0;
}
else
x = 0;
WriteableBitmap bmp = WriteableBitmapExtensions.Crop(<code>mainImage</code>, x, y, num1, num1);
int num2 = (int)Math.Sqrt((double)<code>size</code>);
int num3 = num1 / num2;
for (int index1 = 0; index1 < num2; ++index1)
{
for (int index2 = 0; index2 < num2; ++index2)
{
WriteableBitmap writeableBitmap = WriteableBitmapExtensions.Crop(bmp, index2 * num3, index1 * num3, num3, num3);
list.Add(writeableBitmap);
}
}
return list;
}
This function return as well a List of WriteableBitmap.
After that you bind this List to the number of images that you create it on the XAML :
for (int index = 0; index <= 16 - 1; ++index)
{
(this.GridMain.FindName("P_4_" + index.ToString()) as Image).Source = (ImageSource)wplist[(int)Convert.ToInt16(this.pictureArray[index])];
}
History
If you are Interesting in this sample you can Download it and take the rest of the project. hope you enjoy and like it :)
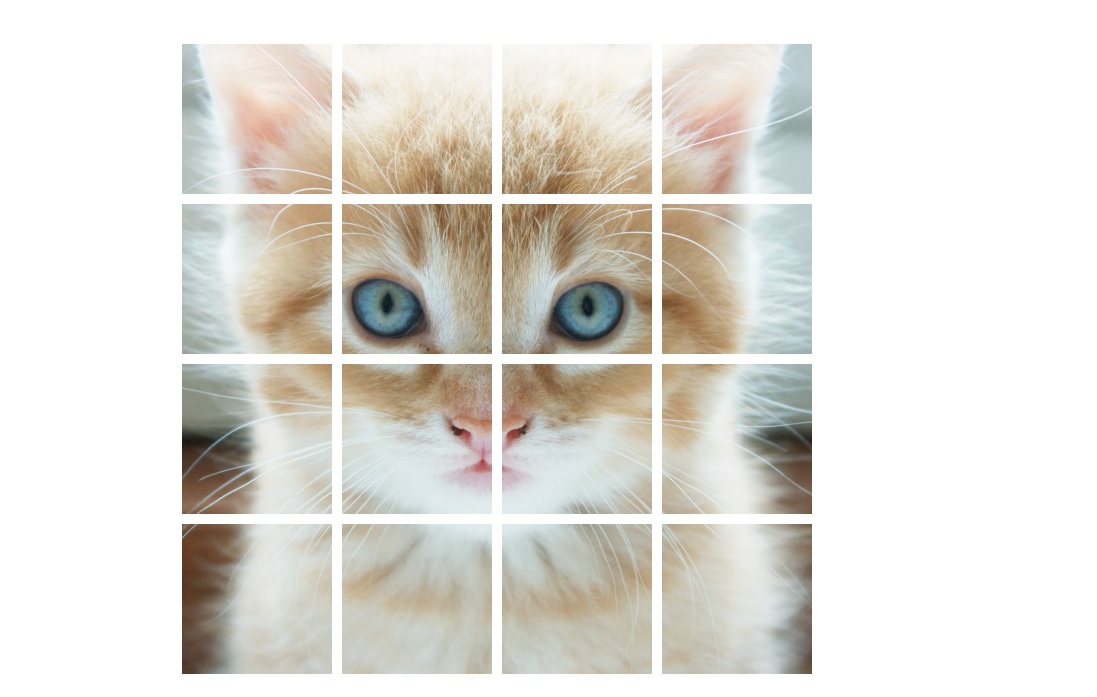