Introduction
Animations always attract users to use the App usually , it's very important to incude some animation on your App
I will show you how to do it with XAML then With C#. Let's Start!
You can download the Full Project here
Using the code
At first we add the tag Storyboard in Page's Ressources. and you should give it a name.
<Page.Resources>
<Storyboard x:Name="storyboard1">
</Storyboard>
</Page.Resources>
After that we add DoubleAnimation
in animation.
Thera are many types of animations. I will show you 3 types. TranslateX or Y , Rotation and Opacity.
Part1 : Using XAML
1-Translate X
<DoubleAnimation
AutoReverse="True"
RepeatBehavior="forever"
Duration="0:0:2"
To="100.0"
Storyboard.TargetProperty="(UIElement.RenderTransform).(CompositeTransform.TranslateX)"
Storyboard.TargetName="rec2" />
Each animation has these properties :
AutoReverse
: if we want to return back the animation.
RepeatBehavior
: if want to keep the animation forever.
Duration
: specify the duration of the animation
Storyboard.TargetProperty
: specify the type of animation.
Storyboard.TargetName
: specify the name of the object that we want to animate.
2-Translate Y
just change the Storyboard.TargetProperty
:
Storyboard.TargetProperty="(UIElement.RenderTransform).(CompositeTransform.TranslateY)"
3-Translate X and Y at the same time
Just add 2 DoubleAnimation
with the same Storyboard.TargetName.
it means on the same object.
PS: You should add the tag CompositeTransform
on your object :
<Grid.RenderTransform>
<CompositeTransform/>
</Grid.RenderTransform>
4- Rotation
Changing as usual the Storyboard.TargetProperty :
Storyboard.TargetProperty="(UIElement.RenderTransform).(RotateTransform.Angle)"
Ps : you should add the tag RotateTransform
on your object :
<Grid.RenderTransform>
<RotateTransform/>
</Grid.RenderTransform>
5-Opacity
Storyboard.TargetProperty="Opacity"
Part2 : Using C#
Now we can do the same animation with a pure c# to do it dynamically. so here is the following Code , now you can understand it ;)
Storyboard storyboard1 = new Storyboard();
((UIElement)rec1).RenderTransform = (Transform)new TranslateTransform();
DoubleAnimation doubleAnimation1 = new DoubleAnimation();
doubleAnimation1.Duration = new Duration(new TimeSpan(0, 0, 2));
doubleAnimation1.To = 100;
doubleAnimation1.AutoReverse = true;
doubleAnimation1.RepeatBehavior = RepeatBehavior.Forever;
Storyboard.SetTarget((Timeline)doubleAnimation1, (DependencyObject)rec1.RenderTransform);
Storyboard.SetTargetProperty((Timeline)doubleAnimation1,"X");
((ICollection<Timeline>)storyboard1.Children).Add((Timeline)doubleAnimation1);
Ps : if you are working on window phone change :
Storyboard.SetTargetProperty((Timeline)doubleAnimation1,"X");
With :
Storyboard.SetTargetProperty((Timeline)doubleAnimation1, new PropertyPath("X"));
After we create our Storyboard , we need now to declenche it whenever we want :
storyboard1.Begin();
and Stopped as well :
storyboard1.Stop();
Sometimes we need to execute some codes just after the finish of the Animation se we declenche the Event Completed :
storyboard1.Completed += storyboard1_Completed;
void storyboard1_Completed(object sender, object e)
{
}
History
Don't forget to download it from here , it's very important to add it in your Apps , Good luck :)
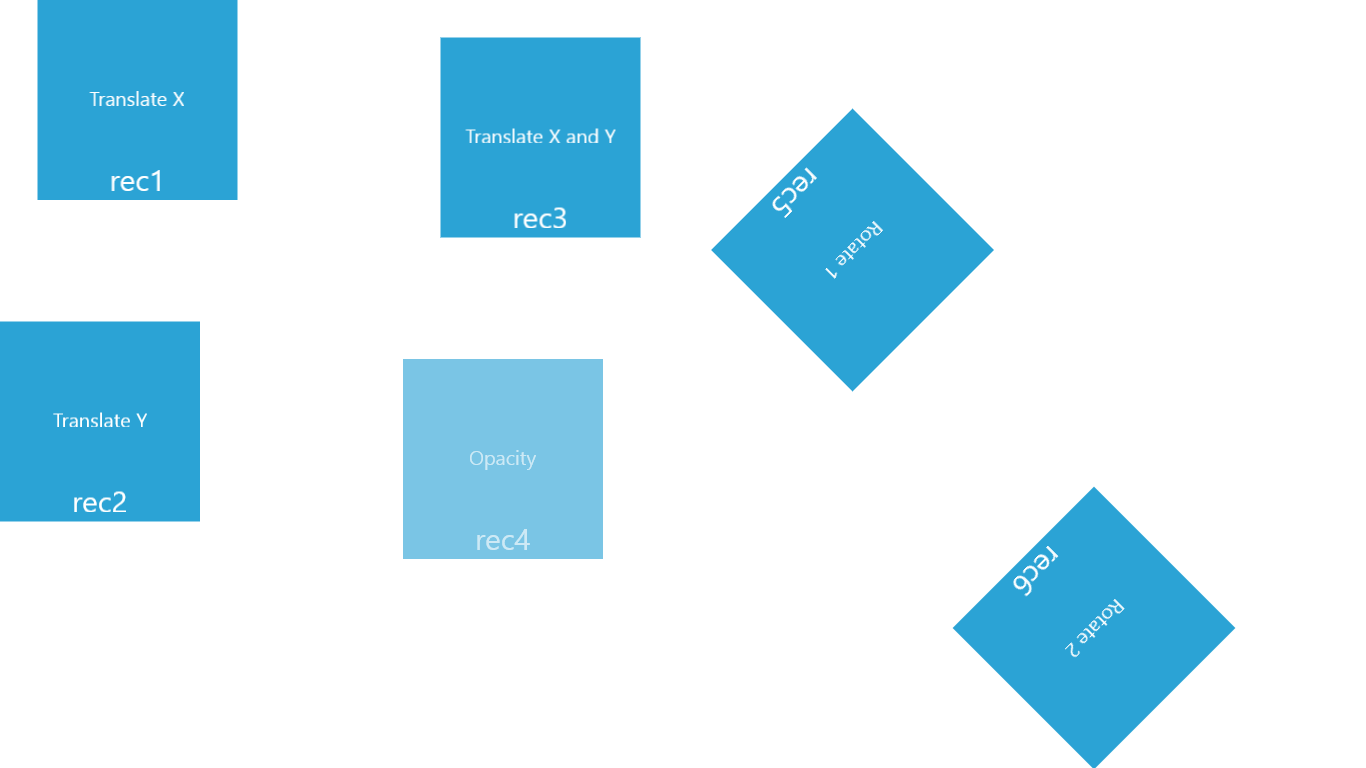