Introduction
This library contains simple ways to create a pie chart with basic interaction and labels.
Steps to Implement
- Calculate the percentage of the values.
- We can find sweep angle with percentage.
- Add the current pie slice sweep angle with previous pie slice end angle.
- Create new pie slice view with start angle and sweep angle (Make sure to apply a different color for each pie splice).
Code
Iterate all the points and calculate the angle and add pie slice to the layout.
total = 0;
for (int i = 0; i < data.length; i++) {
total += data[i];
}
float startAngle = 0, sweepAngle;
for (int i = 0; i < data.length; i++) {
sweepAngle = data[i] * (360f / total);
PieSlice pieSlice = new PieSlice(getContext(), this);
pieSlice.startAngele = startAngle;
pieSlice.sweepAngle = sweepAngle;
addView(pieSlice);
pieSlices.add(pieSlice);
pieSlice.paint.setColor(palette[i % 6]);
startAngle += sweepAngle;
}
Draw pie slice with the above created points.
canvas.drawArc(oval, startAngele, sweepAngle, true, paint);
float angle = (startAngele + (sweepAngle / 2)) * D_TO_R;
canvas.save();
if (label != null) {
labelPaint.getTextBounds(label, 0, label.length(), labelRect);
float x = oval.centerX() + (float) Math.cos(angle) * (oval.width() / 4 + labelRect.width() / 2);
float y = oval.centerY() + (float) Math.sin(angle) * (oval.height() / 4 + labelRect.width() / 2);
x -= labelRect.width() / 2;
canvas.rotate(startAngele + (sweepAngle / 2), x + labelRect.exactCenterX(), y + labelRect.exactCenterY());
canvas.drawText(label, x, y, labelPaint);
}
canvas.restore();
Output
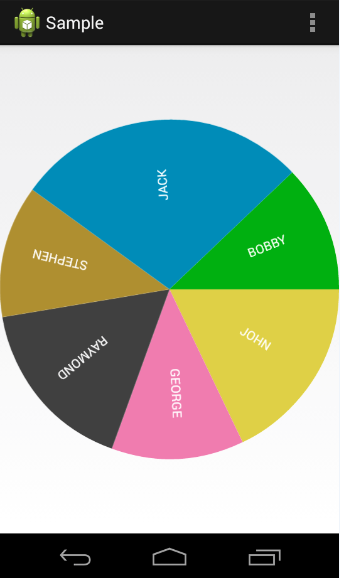
Steps to Run Sample
Android Studio
- Unzip the PieChart.zip folder.
- Create new project in Android studio.
- File --> Import module, import Library and sample module from extracted folder.
- Add the Library module dependency to Sample module.
Eclipse
Indirect solution:
- Create new project with two modules.
- Replace all the required files from extracted PieChart folder.