Here I'll try to explain a simple way to setup a gamepad to work in Unity3D.
First, we have to configure the editor(Edit->Project Settings->Input), by creating as many buttons as required. Create a name of your choice for each and in the value of Positive Button, use "joystick button 0" for first one, then, increase the number accordingly for the rest.
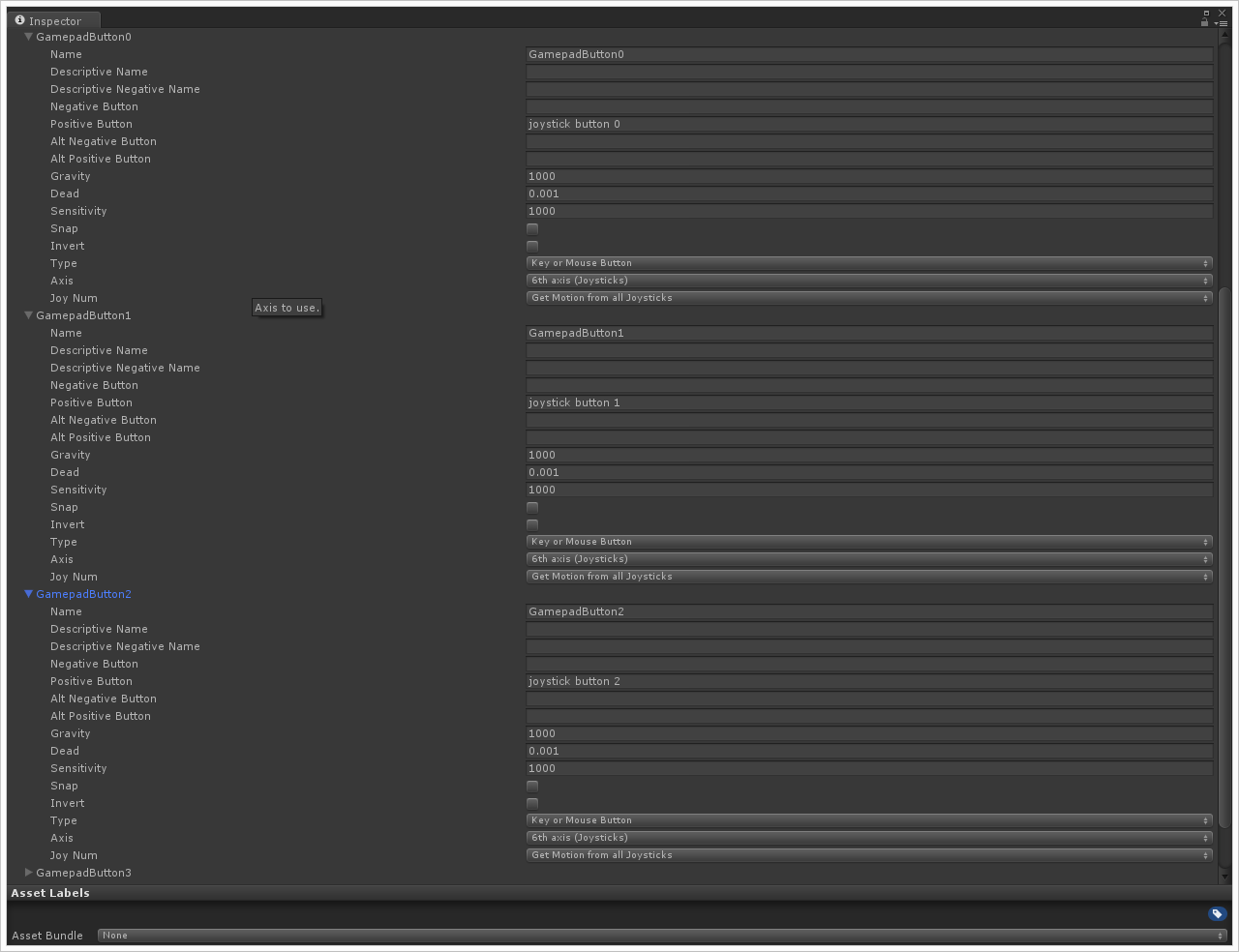
This will create an axis for each.
Then we create our script, for which we also have a Name
.
This Name
will later be set accordingly in the inspector.
The Actor
field in the script is just a shortcut to simulate click a button of your choice, not required, but certainly a shortcut for my own purposes.
[Serializable]
public class GamepadButton
{
public string Name;
public Button Actor;
public bool ButtonState
{
get
{
bool _buttonState =Input.GetButtonUp(this.Name);
if (_buttonState)
this.OnPressed();
return _buttonState;
}
}
private void OnPressed()
{
this.Actor.onClick.Invoke();
}
internal bool IsPressed()
{
return this.ButtonState;
}
}
[Serializable]
public class Gamepad
{
public GamepadButton Button0;
public GamepadButton Button1;
public GamepadButton Button2;
public GamepadButton Button3;
public GamepadButton Button4;
public GamepadButton Button5;
public GamepadButton Button6;
}
Then we add a Gamepad
type property to our player's script.
public Gamepad GamepadConfig;
which will allow us to configure it from the inspector.

Notice that on the Name
configuration, we had used the same names as when configuring the gamepad in the Project Settings Input.
In my personal situation, what I am doing is detecting which gamepad button was pressed and based on that, execute the respective action of the Actor button.
I hope you liked it and that it works for anybody.
As always, this is just a basic sample. I'm not an expert myself and there will always be much better ways to do things.
Feedback is always welcome.