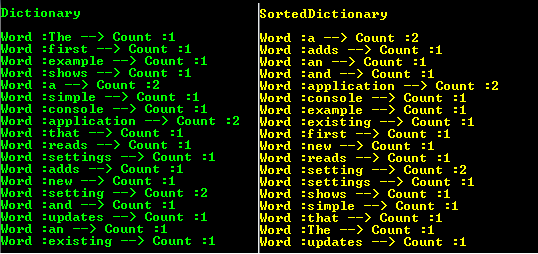
Introduction
This tip demonstrates the usage of strategy design pattern. We display two different implementations of IDictionary<K,T>
based on Strategy.
Background
Strategy is a behavioral design pattern that involves removing an algorithm from its host class and putting it
in a separate class. There may be different algorithms (strategies) that are applicable for
a given problem. If the algorithms are all kept in the host, messy code with lots of conditional
statements will result. The Strategy pattern enables a client to choose which
algorithm to use from a family of algorithms and gives it a simple way to access it.
Using the Code
Our family of algorithms, in our current example, is two different implementations of IDictionary<K,T>
. First Algorithm will return a simple Dictionary<K,T>
, whereas the second one will return a SortedDictionary<K,T>
.
public interface IDictionaryStrategy
{
IDictionary<string, int> GetProcessedText(string text);
}
public class DictionaryStrategy : IDictionaryStrategy
{
public IDictionary<string, int> GetProcessedText(string text)
{
string[] words = text.Split(' ', ';', ',', '.');
var dictionary = new Dictionary<string, int>();
int count = 1;
foreach (var item in words.Where(x => x != string.Empty))
{
int value=0;
if(!dictionary.TryGetValue(item,out value))
{
count = 0;
}
dictionary[item] = ++count;
}
return dictionary;
}
}
public class SortedDictionaryStrategy : IDictionaryStrategy
{
public IDictionary<string, int> GetProcessedText(string text)
{
string[] words = text.Split(' ', ';', ',', '.');
var dictionary = new SortedDictionary<string, int>(new CaseInsensitiveComparer());
int count = 1;
foreach (var item in words.Where(x=>x!=string.Empty))
{
int value = 0;
if (!dictionary.TryGetValue(item, out value))
{
count = 0;
}
dictionary[item] = ++count;
}
return dictionary;
}
class CaseInsensitiveComparer : IComparer<string>
{
public int Compare(string s1, string s2)
{
return string.Compare(s1, s2, true);
}
}
}
Points of Interest
Big fan of GoF!