Revisit Episode 1: Click here
Introduction
We'll cover the following topics in this article:
- AngularJs Module
- Creating Module
- AngularJs Controller
- About Scope
- Creating Controller
- Scope behavior
- ng-repeat Directive with filters example
AngularJs Module
- A detachable and independent code component
- A (logical) container or collection of various key parts/components like directives, filters, controllers, services and more
- Does auto-bootstrapping of application
- Gives better code management and loose coupling
- Used to reasonable breakdown of large size code for better readability
- Used to avoid declaration of objects at global namespace
- Also, plays an important role in dependency injection
- Can be defined inline or be kept in a separate JavaScript (.js) file
How to Define a Module?
Mandatory: All AngularJs modules or any other third party modules need to be registered.
angular.module()
– Use this predefined function for creating a module
Syntax
Without dependency
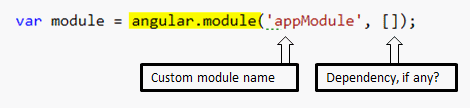
In the above syntax, currently there is no dependency but you can mention the dependencies if there will be any.
With dependencies

Note
- You can create single or multiple modules in an application based on application requirement.
- One module can be assigned single or multiple relative controllers.
- Dependent modules can also be appended to any module.
AngularJs Controller
- Is a Constructor function, a JavaScript object
- Holds the scope (shared object) of function
- Exposes function behavior that View can use
- Detachable independent component
- Mediator between Model and View (Model<==> Controller <==> View)
- Contains business logic
- Can be created using
ng-controller
directive
What About Scope?
- Include properties for controller
- Include functions for controller
- Glue between a controller and a single view
- Expressions also get evaluated against scope object
- Inherit functionality from
$rootScope
(we'll learn about this shortly)
Points to Remember
- Be careful while using scope
- Scope is not a model but a way to access model
- Refrain from changing scope directly from view - advice
- Keep scope as read-only inside view - advice
- Keep scope write-only inside controller - advice
How to Create Controller?
Syntax
moduleName.controller("controllerName", function($scope){
define variables/properties and assign values
});
Example: Single module and single controller

Example: Single module and multiple controllers
appModule.controller('personalInfoController', function ($scope) {
$scope.StaffName = 'Abhishek Maitrey';
$scope.Experience = '12 Years';
$scope.ContactNumber = '9990-123-456';
$scope.Email = 'support@domain.com';
});
appModule.controller('educationInfoController', function ($scope) {
$scope.LastQualification = 'MCA';
$scope.Stream = 'Computer Science';
$scope.YearOfPassing = '2000';
$scope.Grade = 'A';
});
Views to Use the Controllers
<div ng-controller="personalInfoController">
<p>
Personal Information<br />
--------------------------
</p>
Staff Name :<strong> {{StaffName}}</strong><br />
Relevant Experience :<strong> {{Experience}}</strong><br />
Contact Number : <strong>{{ContactNumber}}</strong><br />
Email : <strong>{{Email}}</strong>
</div>
<div ng-controller="educationInfoController">
<p>
Educational Information<br />
------------------------------
</p>
<!--
<!--
Last Qualification :<strong><span
ng-bind="LastQualification"></span></strong><br />
Stream :<strong> <span ng-bind="Stream"></span></strong><br />
Year of Passing : <strong>{{YearOfPassing}}</strong><br />
Grade : <strong>{{Grade}}</strong>
</div>
Output
About the staff
Personal Information
--------------------------
Staff Name : Abhishek Maitrey
Relevant Experience : 12 Years
Contact Number : 9990-123-456
Email : support@domain.com
Educational Information
------------------------------
Last Qualification :MCA
Stream : Computer Science
Year of Passing : 2000
Grade : A
Scope Behavior and Access Limit
You must have noticed the $scope
object has been defined in each controller. Every controller must have its own $scope
object and this object will have limitation to its respective controller.
For example: If you'll try to use $scope.LastQualification
in <div ng-controller="personalInfoController">
, you should not get the desired output against LastQualification
variable because it was defined under educationInfoController
controller.
ngRepeat Directive with Filter
ng-repeat
- a looping construct ng-repeat
- iterates the collection filter
- transforms the model data filter
- appropriate representation to the view

Output
Example of Array, ng-Repeat directive, and filter
- ABHISHEK from noida - India
- JACK from new jersy - USA
- PHILIS from london - UK
- THIU from bangkok - Thailand
Source code is available with this article.
Next Episodes
Episode 3: Few more directives with examples, creating Custom Directives with example
There are 15 episides in all and the contents are under development.