Introduction
The aim of our project ‘ServerZilla
’ is to encourage transfer files between remote host and FTP server. And this project manages files in both remote host and FTP server. Currently, remote administrator uses TFTP (Trivial File Transferring Protocol) to connect remote host to FTP server. To execute TFTP, the administrator needs to spawn a shell using back connect or SSH (secured shell ).This application is based on Command Line Interface (CLI) and has several commands to encourage transfer files between remote host and FTP server which is very difficult for a normal user. Hence by doing this project, reduce this problem. This project uses a web interface which will be user friendly.
Objectives
- Enable the administrator to connect the web server with FTP server.
- Provide impressive and user-friendly interface.
- Save time of administrator.
- Transfer files between web server and FTP server.
- Enable administrator to browse the files in web server and FTP server.
- Enable administrator to manage the files in web server and FTP server.
Using the Code
The project used technologies like:
- PHP
- JavaScript
- CSS3
- jQuery
- bootstrap
- bootbox, etc
Let's start with FTP functions used in the program.
To connect to FTP server, the function ftp_connection()
is used. The syntax of ftp_connect()
is:
$conn = ftp_connect("hostname");
The variable $conn
is the handler to connection to the FTP server. Later, it is used as reference to the connection.
The next function is to login to FTP server. The function ftp_login()
is used to login to FTP server. The syntax is:
$login = ftp_login($conn, $usr, $pass);
The $usr
is variable in which we have stored the username of user
and $pass
is the variable in which we have stored password of user.
To change the current working directory of FTP server, the function ftp_chdir()
is used. The syntax is:
ftp_chdir($conn, $fpath);
The next function of FTP client is upload file to FTP server. To upload files to FTP server, the function ftp_put()
is used. The syntax is:
ftp_put($conn, $rFile, $lFile, FTP_BINARY);
The variable $rFile
stores the name of remote file, $lFile
stores the name of local file and FTP_BINAY
is the mode of operation.
The next operation of FTP client is download file. To download files to web server, the function ftp_get()
is used. The syntax is:
ftp_get($conn, $lFile, $rFile, FTP_BINARY);
The variable $lFile
stores the name of local file, $rFile
stores the name of remote file and FTP_BINARY
is the mode of operation.
The renaming of files is another function of FTP client. To rename a file on FTP host, the function ftp_rename()
is used. The syntax is:
ftp_rename($conn, $oldname, $newname);
I hope you understand what the variables are used for.
The deletion of file is another function of FTP client. To delete files, the function ftp_delete()
is used. The syntax is:
ftp_delete($conn, $file);
Deleting directory is the hard part because to delete a directory, the directory must be empty. So we use a recursive function to delete directory. The function code is:
function deleteFDir($conn, $path)
{
$list = getfiles(ftp_pwd($conn).'/'.$path);
foreach($list as $item)
{
if($item['type']=='Directory')
{
deleteFDir($conn, $path.'/'.$item['name']);
}
else {
ftp_delete($conn, $path.'/'.$item['name']);
}
}
if(ftp_rmdir($conn, $path))
{
return true;
}
else {
return false;
}
}
To create a new directory in FTP server, the function ftp_mkdir()
is used. The syntax is:
ftp_mkdir($conn, $dir);
I hope you understand all functions used to develop the program. You can check the program source code for further understanding.
Screenshots
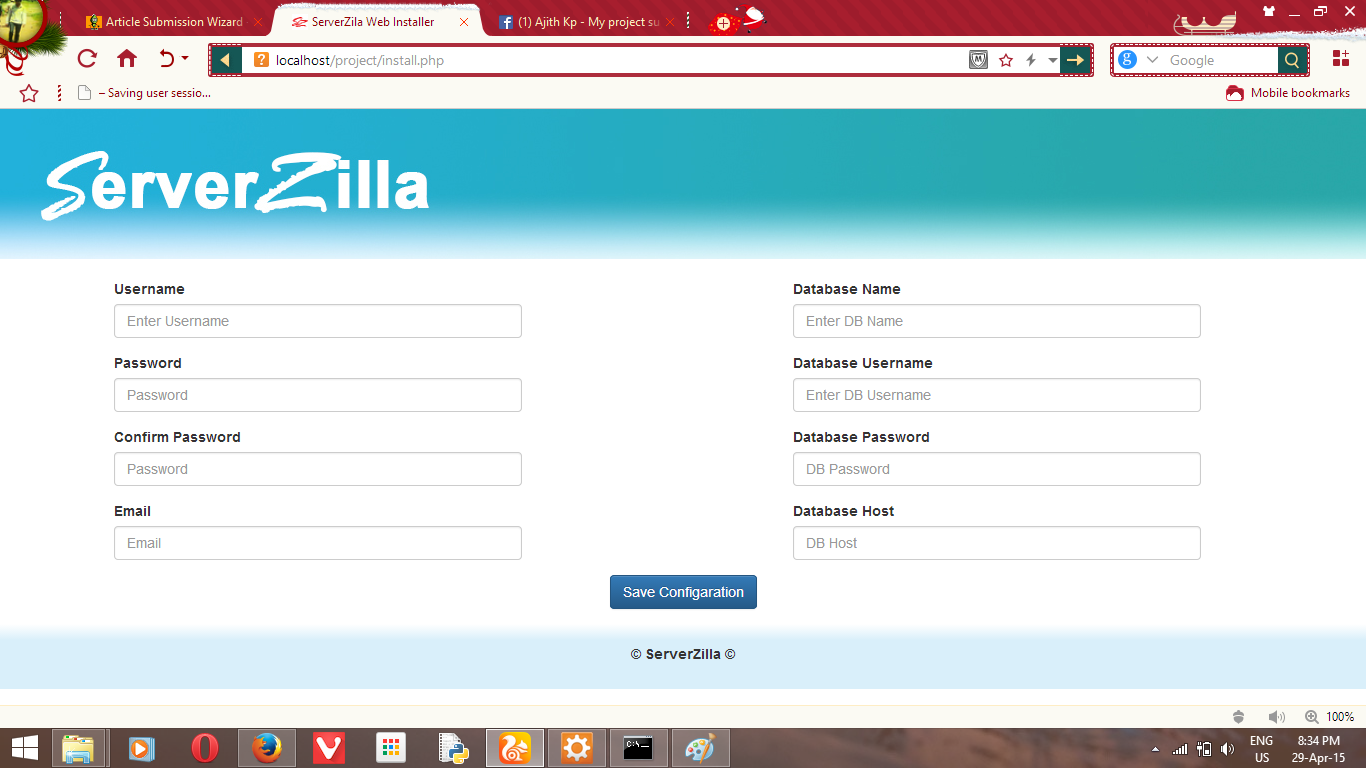




Hope you like the program...