Introduction
When I was reading the MSDN, I found an interesting topic on Windows services and FileSystemWatcher
. So I tried to write a Windows service which monitors the directory changes, writes an entry in the event log about the change, notifies the changes to the users by sending mail, and also converts the input XML file into a DataSet
.
This service is useful in scenarios where the clients upload the files (say XML files) on the server and the server will take care of processing the uploaded files and update them in the database if they are valid.
Building the Application
Start by creating a new Windows service project:
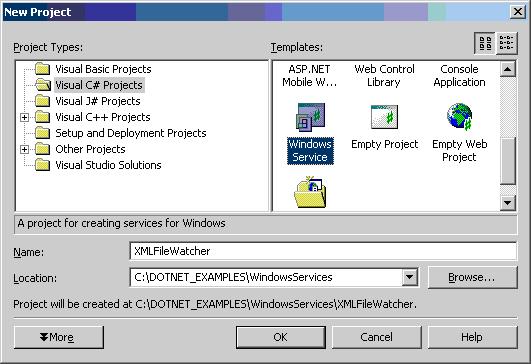
Change the service1.cs name to XMLWatcher.cs by editing the service1.cs properties in the Solution Explorer and also change service1 to XMLWatcher in the generated view code window.

This application uses FileSystemWatcher
to observe the directory changes and SmtpMail
to send the mails. To access FileSystemWatcher
and MailMessage
(in SmtpMail
), we need to add references to System.IO
and System.Web.dll to this project using Project->Add Reference. We should also add a reference to System.Data.dll to access ADO.NET objects.


Include System.IO
, System.Web.Mail
and System.Data.SqlClient
namespaces to this project.
using System.IO;
using System.Web.Mail;
using System.Data.SqlClient;

Now write the code to initialize the Event Log, FileSystemWatcher
and mail server in InitializEventLog()
, IntializeFileSystemWatcher()
and InitializeMailServer()
respectively, and call them from OnStart()
;
private void InitializEventLog()
{
if(!System.Diagnostics.EventLog.SourceExists("XMLWatcherSource"))
{
System.Diagnostics.EventLog.CreateEventSource("XMLWatcherSource","XMLWatcherLog");
}
el=new EventLog();
el.Log="XMLWatcherLog";
el.Source="XMLWatcherSource";
}
private void IntializeFileSystemWatcher()
{
fsWatcher=new System.IO.FileSystemWatcher("c:\\temp","*.xml");
fsWatcher.Changed += new FileSystemEventHandler(OnXMLFileChanged);
fsWatcher.Created += new FileSystemEventHandler(OnXMLFileCreated);
fsWatcher.EnableRaisingEvents = true;
}
private void InitializeMailServer()
{
mailMsg=new System.Web.Mail.MailMessage();
}
protected override void OnStart(string[] args)
{
InitializEventLog();
IntializeFileSystemWatcher();
InitializeMailServer();
}
Now provide Event Handlers for FileSystemWatcher
. These Event Handlers get called when a file is changed or created. Event Handlers will write the entry into Event Log and notify the required people by sending a mail and also converts the XML data into a DataSet
. This DataSet
can be used to update the database.
</summary>
private void OnXMLFileChanged(object source, FileSystemEventArgs e)
{
el.WriteEntry("XML File :" + e.FullPath + " changed");
SendMail(e.FullPath);
GetDataSetFromXML(e.FullPath);
}
private void OnXMLFileCreated(object source, FileSystemEventArgs e)
{
el.WriteEntry("XML File :" + e.FullPath + " created");
SendMail(e.FullPath);
GetDataSetFromXML(e.FullPath);
}
private void SendMail(string XMLFileName)
{
string fileName=XMLFileName;
mailMsg.From="admin@xxx.com";
mailMsg.To="dev@xxx.com";
mailMsg.Subject="New File Uploaded to the server ";
mailMsg.Body="XML File :" + fileName + " is uploaded ";
SmtpMail.Send(mailMsg);
}
private DataSet GetDataSetFromXML(string XMLFileName)
{
string uploadedXMLfile = XMLFileName;
DataSet ds = new DataSet();
System.IO.FileStream fsReadXml = new System.IO.FileStream
(uploadedXMLfile, System.IO.FileMode.Open);
try
{
ds.ReadXml(fsReadXml);
}
catch (Exception ex)
{
fsReadXml.Close();
el.WriteEntry("Error in readig XML File : "
+ uploadedXMLfile );
}
return ds;
}
We have done with the coding. Now this service needs to be installed under the services. To install this service, we need to add installers to this project.
To add installers to the project, open XMLWatcher.cs in design mode and right click on the form and click Add Installer.
Visual Studio will add ServiceProcessInsatller1
and ServiceInsatller1
to the project.

Right click on the ServiceProcessInsatller1
properties and change the Account to Local System to avoid user name, password entry while installing the service.

Now build the project. After build succeeds, open Visual Studio .NET 2003 Command Prompt. Using installutil command, install the service.

Now the service is installed under the Services. To start the service, go to Administrative Tools->Services, and look for XMLWatcherservice
and start the service.
Now the service is up and running. It's time to test the service.
To test the service, create an XML file in c:\temp. Service will monitor the directory change and write an entry in the Event Log under XMLWatcherLog. It will also send a mail to the required users about the directory change.
This application can be further extended to insert the DataSet
(which is retrieved from GetDataSetFromXML
) into the database.