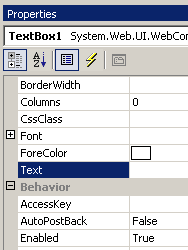
Introduction
When creating a control you will normally start with the lowest common denominator base control that provides you with some base functionality. Sometimes however the control you start with may present properties to the PropertyGrid
that you do not wish to expose in your new control in the Designer as they may no longer be relevant. Here we explain two ways that you can hide these properties which you may find useful.
Hiding properties from the PropertyGrid
To hide properties from the PropertyGrid
is simple all you need to use is the BrowsableAttribute
and set its value to false
.
[Browsable(false)]
public int AnIntegerProperty
{
get
{
...
}
set
{
...
}
}
So, to hide an inherited property all you need to do is encapsulate that property and add the attribute.
[Browsable(false)]
public override string Text
{
get
{
...
}
set
{
...
}
}
Sometimes you find that you may need to add the virtual
or new
keyword depending on the circumstances. Now this is an easy approach, however all you are doing is adding needless code in order to get the PropertyGrid
to display what you want it to display.
An alternative approach
When you create a control it is a common practice to associate a designer with the control and using a designer allows you to separate the design-time functionality of your control from the run-time. A designer has an override called PreFilterProperties
that can be used to add extra attributes to the collection that has been extracted by the PropertyGrid
.
using System.ComponentModel;
[ToolboxData("<{0}:MyControl runat=server></{0}:MyControl>"),
Designer(typeof(MyControlDesigner))]
public class MyControl : ...
{
...
}
public class MyControlDesigner : ...
{
...
protected override void PreFilterProperties(
IDictionary properties)
{
base.PreFilterProperties (properties);
string[] propertiesToHide =
{"MyProperty", "ErrorMessage"};
foreach(string propname in propertiesToHide)
{
prop =
(PropertyDescriptor)properties[propname];
if(prop!=null)
{
AttributeCollection runtimeAttributes =
prop.Attributes;
Attribute[] attrs =
new Attribute[runtimeAttributes.Count + 1];
runtimeAttributes.CopyTo(attrs, 0);
attrs[runtimeAttributes.Count] =
new BrowsableAttribute(false);
prop =
TypeDescriptor.CreateProperty(this.GetType(),
propname, prop.PropertyType,attrs);
properties[propname] = prop;
}
}
}
}
By adding the names of the properties you wish to hide to the collection propertiesToHide
, you now have a quick and easy way of hiding properties without writing lots of needless code. With a little extra effort you can add this code to a base designer class and add it to all your controls where required.
Known issues
It has been noted that some properties when manipulated in this way may cause issues when the control is being edited. If you have an issue then you can use the first solution in order to hide the property, an example is the Text
property when using a control based on the BaseValidator
. It is still being investigated if it is always the Text
property or if some other issue is causing the misbehavior.
Comments
Please take the time to vote for this article and/or to comment about it on the boards below. All suggestions for improvements will be considered.
History
- 22nd August, 2005 - v1
- 25th August, 2005 - v1.1 - added known issues.