Introduction
HTML5 has provided a great feature to validate forms but still it's not very helpful while handling it in JavaScript, and it has always been annoying to write separate codes for each form each time. Here is the JavaScript jrValidator to get rid of this problem. The set of JavaScript functions in jrValidator is light-weight, simple and very easy to use and modify the code. It has been written to meet the requirements of basic form validation.
LIVE DEMO
How to Add this Source to a Project?
- Download jrValidator src file from github
- Add jrValidator.min.css and jrValidator.min.js to your script where the form should be validated from the src folder:
-
<!--
<link href="src/jrValidator.min.css" rel="stylesheet">
-
<!--
<script href="src/jrValidator.min.js"></script>
- Now validate your form using the guidelines given below:
NOTE: Don't forget to add jquery library.
Validating a Form using jrValidator
There is a set of HTML attributes that should be included in form
tags to validate it. We will discuss these attributes later in this article.
Using jrValidateForm() Function
This function validates form and returns true
or false
:
var formValidated = jrValidateForm('form_id');
Example:
HTML:
<form id="form_id">
<!--
<input type="button" onclick="submitForm('form_id');">
</form>
JavaScript:
function submitForm(form_id){
if(jrValidateForm(form_id)){
}else{
}
}
Set the input as Compulsory Field
Use attribute required
in the compulsory inputs:
Example:
<input type="email" required>
<!--
<select type="gender" required>
<option>Female</option>
<option>Male</option>
<option>Other</option>
</select>
Using custom error message for Each Invalid Input
Use attribute data-validation-message
in the inputs you want to set custom error messages.
Example:
<input type="email" data-validation-message="This is my custom error message">
If you are happy with the default error messages, then don't add this attribute to the input:
Set accepted file formats for File Type Inputs
Use attribute data-accepted-file-format
in the file type inputs:
Example:
<input type="file" data-accepted-file-format="jpg, gif, png"
data-validation-message="This is my custom error message">
There is a default message box to show the error message (as given in the image below), but you can also get the message at your preferred location:
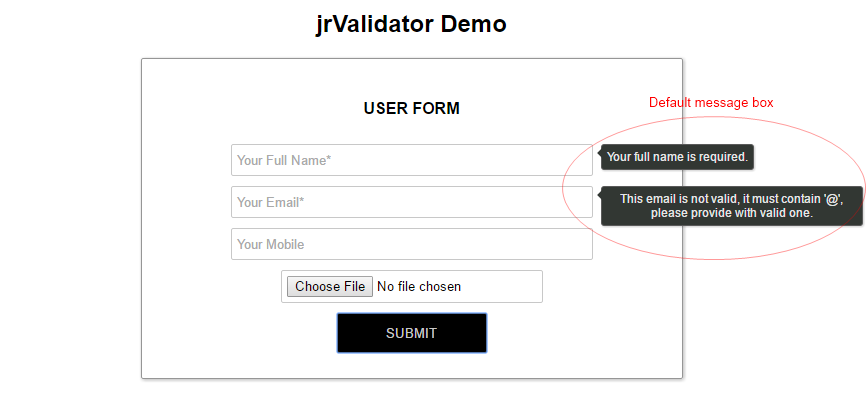
Common error message box for all the Inputs
Use attribute data-message-loc
in the form
tag:
Example:
<form type="email" data-message-loc="msg-box-id">
<!--
</form>
Note: Here 'msg-box-id
' is the id of common error message box.

Exclusive error message box for Each Input
Use attribute data-message-loc
in each input
tag:
Example:
<input data-message-loc="msg-box-id1">
<input data-message-loc="msg-box-id2">

Using data-custom-regex Attribute to Define the Custom Format for Inputs
You can simply add data-custom-regex
attribute to the input to set the format of input value according to your needs.
Example:
For example, if you want to take a 10 digit number from the user, you can simply write this code:
<input type="text" data-custom-regex="^[0-9]{10}$">
NOTE: Here, "^[0-9]{10}$
" is the regex for a 10 digit number.
Using data-password-pattern Attribute to Validate Passwords
There are 5 types of password patterns in jrValidator
, these are discussed below:
Type 1: Minimum 8 characters, at least 1 Alphabet and 1 Number:
Example: valid12345
<input type="password" data-password-pattern="1">
Type 2: Minimum 8 characters, at least 1 Alphabet, 1 Number and 1 Special Character:
Example: valid#12345
<input type="password" data-password-pattern="2">
Type 3 : Minimum 8 characters at least 1 Uppercase Alphabet, 1 Lowercase Alphabet and 1 Number:
Example: Valid12345
<input type="password" data-password-pattern="3">
Type 4 : Minimum 8 characters at least 1 Uppercase Alphabet, 1 Lowercase Alphabet, 1 Number and 1 Special Character:
Example: Valid@12345
<input type="password" data-password-pattern="4">
Type 5: Minimum 8 and Maximum 10 characters at least 1 Uppercase Alphabet, 1 Lowercase Alphabet, 1 Number and 1 Special Character:
Example: Valid@123
<input type="password" data-password-pattern="5">
Custom: Provide your own regex to validate password
Example: 1234587100
<input type="password" data-custom-regex="^[0-9]{10}$">
Validating Retype-Passwords
It can be done by simply adding two attributes data-password-pattern
and data-for-password-id
in the password type input:
<input type="password" name="re_password" data-password-pattern="retype"
data-for-password-id="user_password">
NOTE: Here, "user_password
" is the id of password type input which is to be matched with the retype
password.
Glance Over the Attributes Used
required
- used to specify that the input field must be filled by the user
data-validation-message
- Specify the custom error message
data-accepted-file-format
- Specify the file format to be accepted by file type input
data-message-loc
- Specify the location where error message will be displayed
data-custom-regex
- Specify the custom format of input (accept regex only)
data-password-pattern
- Specify the custom format of input (accepts the only regex)
data-for-password-id
- Specify the id of password to be matched with re-password