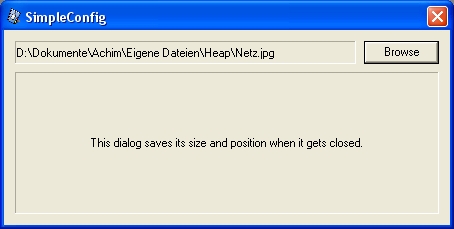
Introduction
To increase an application's usability it is often a good idea to deal with some persistent information. If someone doesn't want to hide these parameters in his Registry (maybe he often modifies these parameters manually), he could use a plain text file instead. The Config
class that is presented in this example, offers a complete solution to handle plain text files as config files.
Features
- common parameter handling
- scope names: different config values can have equal names.
- <scopeA:name> = <value>
- <scopeB:name> = <value>
- value lists: indices will be added automatically, if <name> specifies a value array.
- <name[0]> = <value>
- <name[1]> = <value>
- <name[2]> = <value>
The config file
This example deals with:
- the window's last size and position
- the filters of the
CFileDialog
- the index of the last used filter
- the directory of the last opened file

Using the code
Tip
If you include the Config.h header in your StdAfx.h, you will be able to access all your config values from any file that itself includes the StdAfx.h.
Example
Calling all necessary functions to obtain the values from a value list. (Doing this in just one function is a bit hypothetical.)
Config::initialize();
Config::import("MyApp.cfg");
vector<string> values;
const char* value = Config::getValue("Foo");
while(value)
{
values.push_back(value);
value = Config::getNextValue();
}
Config::export();
Config::depose();
Continuative stuff
The outright interface is a bit more extensive. Please have a look at the source code or the available documentation.
Other classes
ConfigValue
This class implements just some conversion constructors. Each constructor converts its passed value to const char*
. All implicit type conversion that is done for you is encoded in this class. Due to this class the Config
class only needs to provide one kind of setValue()
method.
Config::Implementation
You don't have to worry about this class. This class holds all algorithms and data structures that enable the provided functionality. I separated this class from the Config
class to be able to kick out all include
directives from the Config.h file.
History
Version 1.0
Added:
- Config handling
initialize()
depose()
import()
export()
enterScope()
leaveScope()
setValue()
addValue()
getValue()
getNextValue()
removeValue()
removeParameter()
- Implicit type conversion
ConfigValue(int)
ConfigValue(long)
ConfigValue(unsigned long)
ConfigValue(double)
ConfigValue(bool)
ConfigValue(char)
ConfigValue(char*)