Other projects you will need to download
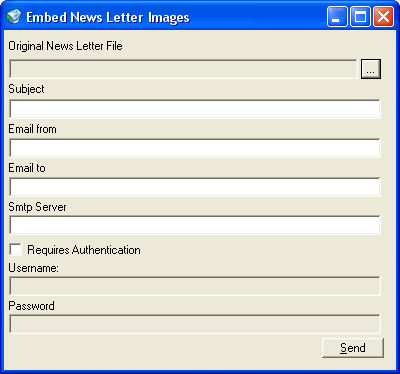
Introduction
How to embed images into an email; short and sweet, but this is all this does.
Background
My sister wanted to send out an email newsletter and wanted to embed images. So, she asked a friend and they sent her some classic ASP code. Then she asked me to make it work. Yeah, right! I fired up VS 2003 and got a hold of MIL HTML Parser and DotNetOpenMail. Within an hour, I had a small little app that could take a webpage, add all the needed images, and change the img
tags to point to the content IDs of the images attached to the email and send it off to an email address.
Using the code
This code is really straightforward. Just select an HTML file that you have created, add a subject, a "from" email address, a "to" email address and the SMTP server name or IP address, and then click Send. But what does the code do? Well, you can't get much simpler than this. The code goes off and gets all the img
elements.
GetImageNodes(document.Nodes);
private void GetImageNodes(HtmlNodeCollection nodes)
{
foreach (HtmlNode node in nodes)
{
HtmlElement element = node as HtmlElement;
if (element != null)
{
if (element.Name.ToLower() == "img")
{
imageNodes.Add(element);
}
if (element.Nodes.Count > 0)
{
GetImageNodes(element.Nodes);
}
}
}
}
Then all the needed images are attached and the src
attributes of the img
elements are set to the content ID of the attached images.
foreach (HtmlElement element in imageNodes)
{
FileInfo imageFileInfo = new FileInfo(Path.Combine(
fileInfo.DirectoryName,
element.Attributes["src"].Value));
string contentId =
imageFileInfo.Name.Replace(imageFileInfo.Extension,
string.Empty);
if (!images.ContainsKey(element.Attributes["src"].Value))
{
images.Add(
element.Attributes["src"].Value, imageFileInfo.FullName);
FileAttachment relatedFileAttachment =
new FileAttachment(imageFileInfo, contentId);
if ((imageFileInfo.Extension == ".jpg") ||
(imageFileInfo.Extension == ".jpeg"))
{
relatedFileAttachment.ContentType = "image/jpeg";
}
else if (imageFileInfo.Extension == ".gif")
{
relatedFileAttachment.ContentType = "image/gif";
}
emailMessage.AddRelatedAttachment(relatedFileAttachment);
}
element.Attributes["src"].Value = string.Format("cid:{0}", contentId);
}
And then the changed HTML is added to the email message and sent off.
emailMessage.HtmlPart = new HtmlAttachment(document.HTML);
emailMessage.Send(new SmtpServer(this.SmtpServerTextBox.Text));
Points of interest
I just slapped this code together in an hour. In fact, writing the article added another 30 minutes. So, this is not code to look to for best practices. I just wanted to embed images into an email with a minimum of hassle and I achieved that goal with this. I hope others will find it useful.
History
- 5/6/2007 - Version 2.0
- 27/9/2005 - Version 1.0 - Also happens to be my birthday! :D