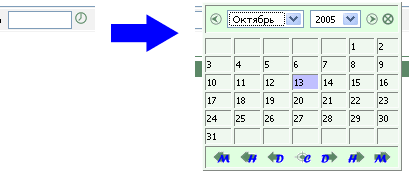
Introduction
Here is the sample code for a DatePicker
in JavaScript. It can be used on HTML pages or as an ASP control - like I use it (see demo files).
Here is the code snippet of the JS code (part of the "render
" function):
DatePicker.prototype.render = function()
{
var oT1, oTR1, oTD1, oTH1;
var oT2, oTR2, oTD2;
this.oSpan = document.getElementById(this.name);
this.oSpan.appendChild(oT1 = document.createElement("table"));
oT1.width = "200";
oT1.border = 1;
oTR1 = oT1.insertRow(oT1.rows.length);
oTD1 = oTR1.insertCell(oTR1.cells.length);
oTD1.colSpan = 7;
oTD1.className = 'DatePickerHdr';
oT2 = document.createElement("table");
oT2.width = "100%";
oTD1.appendChild(oT2);
oT2.border = 0;
oTR2 = oT2.insertRow(oT2.rows.length);
oTD2 = oTR2.insertCell(oTR2.cells.length);
oTD2.title = this.texts.prevMonth;
oTD2.onclick = function() { this.oDatePicker.onPrev(); }
oTD2.oDatePicker = this;
oTD2.innerHTML = "<img src="images/datepicker/prev.gif">";
oTD2.className = 'DatePickerHdrBtn';
oTD2 = oTR2.insertCell(oTR2.cells.length);
oTD2.title = this.texts.monthTitle;
this.oMonth = document.createElement("select");
oTD2.appendChild(this.oMonth);
this.oMonth.oDatePicker = this;
this.oMonth.onchange = this.oMonth.onkeyup =
function() { this.oDatePicker.onMonth(); }
for(var i = 0; i < 12; i++)
{
this.oMonth.add(new Option(this.texts.months[i], i),undefined);
}
this.oMonth.className = 'DatePickerHdrBtn';
oTD2 = oTR2.insertCell(oTR2.cells.length);
oTD2.title = this.texts.yearTitle;
this.oYear = document.createElement("select");
oTD2.appendChild(this.oYear);
this.oYear.oDatePicker = this;
this.oYear.onchange = this.oYear.onkeyup =
function() { this.oDatePicker.onYear(); }
I did use Russian language and Russian date format because the need of the picker was for a Russian site. But the language and format the picker uses can be changed very simply - just change the array values and the "fill
" method:
DatePicker.prototype.fill = function()
{
this.clear();
var nRow = 0;
var d = new Date(this.dt.getTime());
var m = d.getMonth();
for ( d.setDate(1); d.getMonth() == m;
d.setTime(d.getTime() + 86400000) ) {
var nCol = d.getDay();
if(nCol == 0) nCol = 7;
nCol = nCol-1;
this.aCells[nRow][nCol].innerHTML = d.getDate();
if ( d.getDate() == this.dt.getDate() ) {
this.aCells[nRow][nCol].className =
'DatePickerBtnSelect';
}
if ( nCol == 6 ) nRow++;
}
this.oMonth.value = m;
this.oYear.value = this.dt.getFullYear();
}
That is all. I do really think the control is very useful.