Introduction
I developed this class because I needed a general class that will detect internally if a file is an archive, and then read the compressed files contained in the archive. For Zip and gz archives, I found the source code on CodeProject, in the articles of Hans Dietrich and Jonathan de Halleux.
I also implemented in the class the possibility of detecting & reading from tar files.
Using the Code
The class provides methods for: detecting and opening an archive, returning the number of files in an archive, and reading files from archives based on file position. In the header file, we have the methods and their description.
/***********************************************************************
Open a compressed file.
Parameters
pszCompressedFile - name of the file
Return values:
TRUE - file opened
FALSE - file could not be opened
(use GetErrorText() to find the error)\
Note:
The archive file must have one of these extensions:
- .zip
- .gz
- .tar.gz
- .tgz
***********************************************************************/
BOOL OpenArchive(IN const char* pszArchiveFile);
/***********************************************************************
Closes the archive, destroys all the internal objects
***********************************************************************/
void CloseArchive();
/***********************************************************************
Get number of files in the archive
Return values:
Number of files in the archive.
-1 if there are errors.
***********************************************************************/
int GetCompressedFilesCount();
/***********************************************************************
Get a buffer that contain the file in archive at a specific position
Params:
nFileIndex - file position in archive
pszFileBuffer - buffer containing the file data
nFileSize - file size
fIsFile - TRUE if this is a regular file,
FALSE otherwise (directory, link, etc)
szFileName - name of the file
Return values:
TRUE - file found and buffer filled correctly
FALSE - some error (use GetErrorText() to find the error)
Note:
All data buffers will be allocated/deleted internally in this class,
they should not be changed from outside !
***********************************************************************/
BOOL GetArchiveFile( IN int nFileIndex,
OUT char** pszFileBuffer,
OUT int& nFileSize,
OUT BOOL& fIsFile,
OUT CString& szFileName);
/***********************************************************************
Get last error if there were any problems
***********************************************************************/
CString GetErrorText();
BOOL OpenArchive(IN const char* pszArchiveFile);
How to Use
Include the DecompressLibrary
project in your workspace. Next, include the DecompressClass.h header file. Sample usage:
CDecompressClass testObj;
testObj.OpenArchive("archivefile.zip");
for (int i=0; i < testObj.GetCompressedFilesCount(); i++)
{
char* pszFileBuffer;
int nFileLength;
CString szFileName;
BOOL fIsFile;
testObj.GetArchiveFile(i,
&pszFileBuffer,
nFileLength,
fIsFile,
szFileName
);
}
History
- Version 1.0 - 2005 Nov 14, first implementation of the class
Demo App
You will find a test dialog used to test the class:
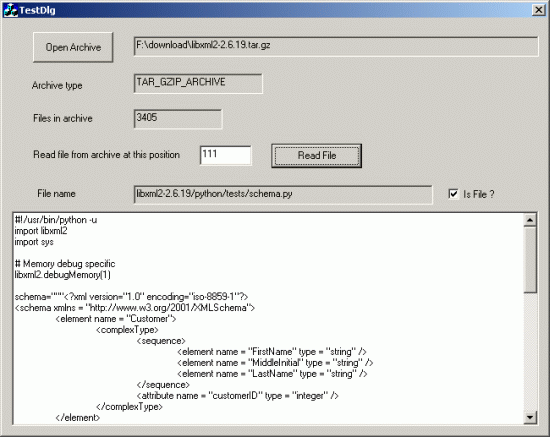
Usage
This software is created by Marius Daniel Ciorecan at https://elapseit.com and is released into the public domain. You are free to use it in any way you like. If you modify it or extend it, please consider posting new code here for everyone to share. This software is provided "as is" with no expressed or implied warranty. I accept no liability for any damage or loss of business that this software may cause.