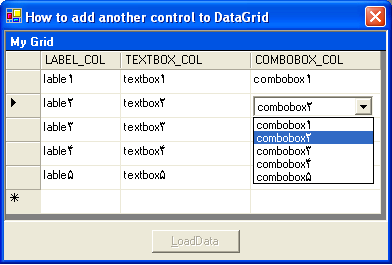
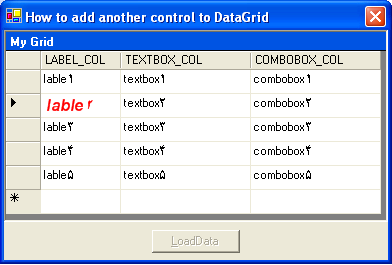
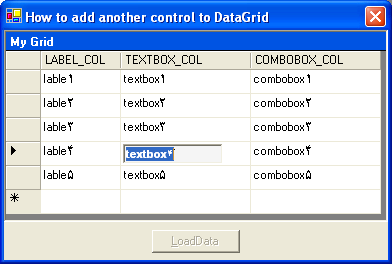
Introduction
I always wanted to know how to use controls such as TextBox
, ComboBox
, RadioButton
, Button
etc. in a DataGrid
cell. You can also fire their events easily. Nowadays it's very simple to do this! You must create a DataGridTableStyle
and add a DataGridTextboxColumn
s to it. After that, you can add each control you want to the DataGridTextboxColumn
s.
TextBox txtBox = new TextBox();
datagridTextBoxColumn.TextBox.Controls.Add( txtBox );
Now, let me describe my sample. First, I design a DataTable
and fill my DataGrid
with it:
private DataSet ds = new DataSet("myDs");
private DataTable dt = new DataTable("myDT");
private void FillData()
{
ds.Tables.Add(dt);
DataColumn dc = new DataColumn("Label_Col" ,
System.Type.GetType("System.String"));
dt.Columns.Add(dc);
dc = new DataColumn("TextBox_Col" ,
System.Type.GetType("System.String"));
dt.Columns.Add(dc);
dc = new DataColumn("ComboBox_Col",
System.Type.GetType("System.String"));
dt.Columns.Add(dc);
DataRow dr;
for(int i=1; i<=5; i++)
{
dr = dt.NewRow();
dr["Label_Col"] = "lable"+i.ToString();
dr["TextBox_Col"] = "textbox"+i.ToString();
dr["ComboBox_Col"] = "combobox"+i.ToString();
dt.Rows.Add(dr);
}
}
After you load data in your DataGrid
and design its DataGridTableStyle
, you must write this code in the MouseUp
event of DataGrid
:
hitTestGrid = dataGrid.HitTest(e.X, e.Y);
if(hitTestGrid != null)
{
switch(hitTestGrid.Column)
{
case 0:
dataGridLable.TextBox.Controls.Add( lblControl );
lblControl.Text =
dataGrid[dataGrid.CurrentRowIndex , 0].ToString();
break;
case 1:
dataGridTextBox.TextBox.Controls.Add( txtControl );
txtControl.Text =
dataGrid[dataGrid.CurrentRowIndex , 1].ToString();
txtControl.Focus();
break;
case 2:
dataGridComboBox.TextBox.Controls.Add( cboControl );
for(int i=0; i<CBOCONTROL.ITEMS.COUNT; pre }< } break;
cboControl.SelectedIndex="i;" 2].ToString()) ,
dataGrid[dataGrid.CurrentRowIndex if(
cboControl.Items[i].ToString()="=" { i++)>
You can initialize your controls, which will be added to the DataGrid
, and fire their events:
private void InitializeControls()
{
lblControl.Cursor = Cursors.Hand;
lblControl.ForeColor = Color.Red;
lblControl.Font = new Font("Arial", 12,
FontStyle.Bold | FontStyle.Italic);
txtControl.Cursor = Cursors.Hand;
txtControl.BackColor = Color.WhiteSmoke;
txtControl.ForeColor = Color.DarkSlateBlue;
txtControl.Font = new Font("Arial", 8, FontStyle.Bold);
txtControl.TextChanged+=new EventHandler(txtTextChanged);
for(int i=1; i<=5; i++)
cboControl.Items.Add("combobox"+i.ToString());
cboControl.Cursor = Cursors.Hand;
cboControl.DropDownStyle = ComboBoxStyle.DropDownList;
cboControl.SelectedIndexChanged+=
new EventHandler(cboSelectedIndexChanged);
}