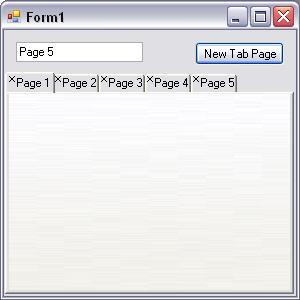
Introduction
I was writing an application that uses a TabControl
. I needed to give my user the ability to close tab pages dynamically, so I was looking for a tab control that has a close sign on every page, but could not find one. So I started developing one. The result of this development is the following class: TabControlEx
.
Using the code
To use the the tab control, I perform the following action:
- Add a regular
TabControl
to my form.
- Edit the Designer file (if your form name is
Form1
, you need to edit the Form1.Designer.cs file).
You need to change the type of the tab control from "System.Windows.Forms.TabControl
" to "Yoramo.GuiLib.TabControlEx
". You also need to change the instantiation statement (in the "InitializeComponent
" method) to create the correct type of tab control.
The TabControlEx Code
TabControlEx
derives from System.Windows.Forms.TabControl
. It implements the OnDrawItem
to draw the 'x' sign on the page (Close sign) and the OnMouseClick
to figure out if the close sign has been clicked. In the constructor, I have changed the DrawMode
to TabDrawMode.OwnerDrawFixed
to activate the OnDrawItem
. In order to enable the application to refuse closing a tab, or to provide an event to enable saving data, I have defined an event called PreRemoveTabPage
.
using System;
using System.Windows.Forms;
using System.Drawing;
namespace Yoramo.GuiLib
{
public delegate bool PreRemoveTab(int indx);
public class TabControlEx : TabControl
{
public TabControlEx()
: base()
{
PreRemoveTabPage = null;
this.DrawMode = TabDrawMode.OwnerDrawFixed;
}
public PreRemoveTab PreRemoveTabPage;
protected override void OnDrawItem(DrawItemEventArgs e)
{
Rectangle r = e.Bounds;
r = GetTabRect(e.Index);
r.Offset(2, 2);
r.Width = 5;
r.Height = 5;
Brush b = new SolidBrush(Color.Black);
Pen p = new Pen(b);
e.Graphics.DrawLine(p, r.X, r.Y, r.X + r.Width, r.Y + r.Height);
e.Graphics.DrawLine(p, r.X + r.Width, r.Y, r.X, r.Y + r.Height);
string titel = this.TabPages[e.Index].Text;
Font f = this.Font;
e.Graphics.DrawString(titel, f, b, new PointF(r.X + 5, r.Y));
}
protected override void OnMouseClick(MouseEventArgs e)
{
Point p = e.Location;
for (int i = 0; i < TabCount; i++)
{
Rectangle r = GetTabRect(i);
r.Offset(2, 2);
r.Width = 5;
r.Height = 5;
if (r.Contains(p))
{
CloseTab(i);
}
}
}
private void CloseTab(int i)
{
if (PreRemoveTabPage != null)
{
bool closeIt = PreRemoveTabPage(i);
if (!closeIt)
return;
}
TabPages.Remove(TabPages[i]);
}
}
}