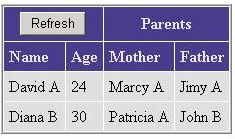
Introduction
This article explains how to add an extra header item to a standard ASP.NET DataGrid
.
Using the code
The code is pretty simple. The grid is built using a DataGridTable
object. All we have to do is to add a DataGridItem
to the Rows
collection in the CreateControlHierarchy
method of our custom grid. Now I'll let the code talk:
Protected Overrides Sub CreateControlHierarchy(ByVal useDataSource As Boolean)
MyBase.CreateControlHierarchy(useDataSource)
If Not ShowExtraHeader Then Return
If Controls.Count > 0 Then
Dim rows As TableRowCollection = DirectCast(Controls(0), Object).Rows
Dim extraHeaderRow As DataGridItem = CreateItem(-1, -1, ListItemType.Header)
extraHeaderRow.ID = "extraheader"
Dim extraHeaderRowArgs As New DataGridItemEventArgs(extraHeaderRow)
OnItemCreated(extraHeaderRowArgs)
rows.AddAt(0, extraHeaderRow)
End If
End Sub
And let's see how we could use this code in a real scenario:
Private Sub g_ItemCreated(ByVal sender As Object, ByVal e _
As System.Web.UI.WebControls.DataGridItemEventArgs) Handles g.ItemCreated
If e.Item.ItemType = ListItemType.Header Then
If e.Item.ID = "extraheader" Then
Dim refreshCell As New TableCell
refreshCell.ColumnSpan = 2
refreshCell.HorizontalAlign = HorizontalAlign.Center
Dim btnRefresh As New Button
btnRefresh.Text = "Refresh"
refreshCell.Controls.Add(btnRefresh)
AddHandler btnRefresh.Click, AddressOf btnRefresh_Click
Dim parentsCell As New TableCell
parentsCell.ColumnSpan = 2
parentsCell.HorizontalAlign = HorizontalAlign.Center
parentsCell.Text = "Parents"
e.Item.Cells.Add(refreshCell)
e.Item.Cells.Add(parentsCell)
End If
End If
End Sub
That's all! Check the demo project to see all the bits.
History
- Dec. 10, 2005 - Version 1.0.