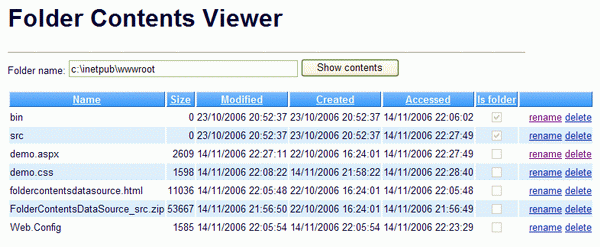
Introduction
In ASP.NET 2.0, accessing data in web pages is handled through data source controls. These are non-visual controls derived from DataSourceControl
. Common examples are the SqlDataSource
and the AccessDataSource
.
Some time ago, I wanted to make a simple file manager. For this, I needed to list the contents of a folder. I decided to create a custom data source control, so that I could use a GridView
control to display the data.
In combination with a GridView
, the control can not only show the contents of a folder, but it can also be used to rename or delete files or folders! Therefore, this can be a great control to create your own file manager.
The control can also be used to create a photo gallery with almost no code, as shown below:

The code is in VB.NET, but it's not difficult to get it running in C# or in another language.
License
The control is free and Open Source under the LGPL license.
Installation
Installing the control
You can install the control in the standard way:
- Create a "bin" folder inside the application root of your website (if it's not already there)
- Copy the assembly file foldercontentsdatasource.dll into the bin folder
You may want to add the control to the toolbox of your editor (Visual Studio or C# Builder). This will allow you to add the control to a page by dragging it. Follow the editor's procedure to add a control to the toolbox.
Using the control
Adding the control to your page
There are two ways to add the control to your page:
- If the control was installed on the toolbox, add a data control to the page first, such as a
GridView
, a DataList
, or a Repeater
. Next, use the Data Source Configuration Wizard to choose a data source. Make sure there's a copy of the evenlogdatasource.dll assembly in the bin folder. 
- Add the code manually. Add this line to the top of your page:
<%@ Register TagPrefix="rw"
Namespace="rw" Assembly="foldercontentsdatasourcecontrol" %>
Then, add a tag like this to your page:
<rw:FolderContentsDataSource
id="FolderContentsDataSource1" runat="server" >
</rw:FolderContentsDataSource>
Finally, add the data control (GridView
or DataList
) to the page, and connect it to the FolderContentsDataSource
.
Setting the folder
The control will show the contents of the folder that is set as a Parameter
. The name of the parameter is "Directory
".
<rw:FolderContentsDataSource ID="FolderContentsDataSource1" runat="server" >
<SelectParameters>
<asp:Parameter Name="Directory" Type="String" DefaultValue="c:\inetpub\wwwroot" />
</SelectParameters>
</rw:FolderContentsDataSource>
You can use any of the Parameter types, such as ControlParameter
or QueryStringParameter
, to connect the parameter value to an environment value. Use the Parameters setting in the Properties window. Please note that you only need a single parameter. This parameter is used for selection, deletion, and renaming of contents.
Demo pages
The control comes with two demo pages:
- demo.aspx shows a list of all the files and subfolders in a selected folder. This page uses a
GridView
control, and a TextBox
control for selecting a folder. The Directory
parameter is set through a ControlParameter
, which connects the TextBox
to the data source. - demogallery.aspx shows a gallery with all the pictures in a given folder. This page uses a
DataList
control bound to the FolderContentsDatasource
control. Use the RepeatColumns
property of the DataList
to control the number of pictures in a row.
Important: you need to run these pages with credentials that have read rights to the folder. For deleting or renaming files, you need the corresponding permissions. Use impersonation, or make sure the folder is accessible for the ASPNET account.
How it works
To build a data source control, I got some ideas from Nikhil Kothari's code at www.nikhilk.net/DataSourceControlsSummary.aspx. I built two main classes: FolderContentsDataSource
and FolderContentsDataSourceView
.
The code reading the folder contents is in the ExecuteSelect
method of the FolderContentsDataSourceView
class. This code generates a DataTable
with a list of entries in the given folder:
Protected Overrides Function ExecuteSelect(ByVal arguments _
As System.Web.UI.DataSourceSelectArguments) _
As System.Collections.IEnumerable
Dim dt As New DataTable()
dt.Columns.Add("Name", System.Type.GetType("System.String"))
dt.Columns.Add("Size", System.Type.GetType("System.Int64"))
dt.Columns.Add("Modified", System.Type.GetType("System.DateTime"))
dt.Columns.Add("Created", System.Type.GetType("System.DateTime"))
dt.Columns.Add("Accessed", System.Type.GetType("System.DateTime"))
dt.Columns.Add("IsFolder", System.Type.GetType("System.Boolean"))
Dim exc As Exception = Nothing
Dim dv As DataView = Nothing
Try
Dim objFolderContents As DirectoryInfo = _
New DirectoryInfo(_owner.GetSelectedDirectory())
Dim objEntries() As FileSystemInfo = _
objFolderContents.GetFileSystemInfos()
Dim objEntry As FileSystemInfo
For Each objEntry In objEntries
Dim dr As DataRow = dt.NewRow()
dr("Name") = objEntry.Name
If (objEntry.Attributes And FileAttributes.Directory) <> 0 Then
dr("Size") = 0
dr("IsFolder") = True
Else
dr("Size") = CType(objEntry, FileInfo).Length
dr("IsFolder") = False
End If
dr("Modified") = objEntry.LastWriteTime
dr("Created") = objEntry.CreationTime
dr("Accessed") = objEntry.LastAccessTime
dt.Rows.Add(dr)
Next
dv = New DataView(dt)
dv.Sort = arguments.SortExpression
Catch ex As Exception
exc = ex
End Try
Dim statusEventArgs As New FolderContentsDataSourceStatusEventArgs(exc)
OnSelected(statusEventArgs)
If (exc IsNot Nothing And Not statusEventArgs.ExceptionHandled) Then
Throw exc
End If
Return dv
End Function
Points of interest
Future
Here are some ideas for improvement:
If anyone decides to extend this control, or has any comments, bug reports, or questions, then it would be great to hear from you.