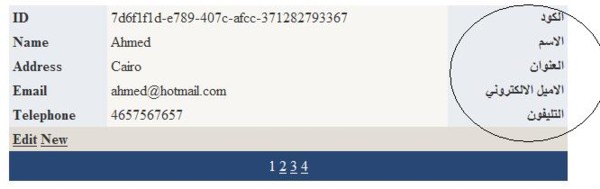
Introduction
After a long search about why DetailsView.FieldTemplate.FooterTemplate
is not rendered, I found out a way to generate it from scratch by modifying the HTML code that is generated by DotNet for the DetailsView
control. I will explain it in the next steps.
Step 1
<asp:DetailsView ID="DetailsView1" runat="server" AllowPaging="True"
AutoGenerateRows="False"
CellPadding="4" DataSourceID="ObjectDataSource1"
ForeColor="#333333" GridLines="None"Height="50px"
OnItemInserting="DetailsView1_ItemInserting"
OnItemUpdated="DetailsView1_ItemUpdated"
Width="50%">
<FooterStyle BackColor="#5D7B9D" Font-Bold="True" ForeColor="White" />
<CommandRowStyle BackColor="#E2DED6" Font-Bold="True" />
<EditRowStyle BackColor="#999999" />
<RowStyle BackColor="#F7F6F3" ForeColor="#333333" />
<PagerStyle BackColor="#284775" ForeColor="White" HorizontalAlign="Center" />
<Fields>
<asp:BoundField DataField="Guid" HeaderText="$ID$"
InsertVisible="False" ReadOnly="True" />
<asp:TemplateField HeaderText="$Ÿéèí§$" InsertVisible="False">
</asp:TemplateField>
<asp:BoundField DataField="Name" HeaderText="$Name$" />
<asp:TemplateField HeaderText="$ŸéŸ«ê$"></asp:TemplateField>
<asp:BoundField DataField="Address" HeaderText="$Address$" />
<asp:TemplateField HeaderText="$ŸéãëíŸë$"></asp:TemplateField>
<asp:BoundField DataField="Email" HeaderText="$Email$" />
<asp:TemplateField HeaderText="$ŸéŸêïé ŸéŸé袩íëï$"></asp:TemplateField>
<asp:BoundField DataField="Tel" HeaderText="$Telephone$" />
<asp:TemplateField HeaderText="$Ÿé¢éïåíë$"></asp:TemplateField>
<asp:CommandField ShowEditButton="True" ShowInsertButton="True" />
</Fields>
<FieldHeaderStyle BackColor="#E9ECF1" Font-Bold="True" />
<HeaderStyle BackColor="#5D7B9D" Font-Bold="True" ForeColor="White" />
<AlternatingRowStyle BackColor="White" ForeColor="#284775" />
</asp:DetailsView>
<asp:ObjectDataSource ID="ObjectDataSource1" runat="server"
DataObjectTypeName="Employee"
InsertMethod="Add" SelectMethod="getAllEmplyees"
TypeName="Employee" UpdateMethod="Update">
</asp:ObjectDataSource>
The code above illustrates that when putting the fields of detailsview
, I put them between two $
as if I have Field(Template Field,Bound Filed,.......)
called (Name)
. I put its headerText
property as $Name$
, and added a new Template column for the Footer and put it between two $
as in the previous example but by using another language that I wanted to use.
Step 2
public static void ApplyFooters(ref string str, DetailsView dv)
{
for (int i = 0; i < dv.Fields.Count - 1; i += 2)
{
FooterTemplate.SetFooter(ref str, dv.Fields[i].HeaderText,
dv.Fields[i + 1].HeaderText, dv.ClientID);
}
FooterTemplate.AdjustGrid(ref str, dv.ClientID);
}
This function takes the HTML code as a ref
string and takes the detailsview
control as a parameter. The function loops on detailsView
Control fields and uses the SetFooter
parameter to generate the footer.
private static void SetFooter(ref string str, string Header,
string Footer, string GridName)
{
int StartGridIndex = str.IndexOf(GridName);
if (StartGridIndex == -1) return;
int EndGridIndex = str.IndexOf("</table>", StartGridIndex);
int HeaderIndex = str.IndexOf(Header);
if (HeaderIndex == -1) return;
int StartIndex = str.IndexOf("</tr>", HeaderIndex);
if (StartIndex == -1 || (StartIndex < StartGridIndex ||
StartIndex > EndGridIndex)) return;
int EndIndex = str.IndexOf(">", StartIndex + 5);
string oHeader = Header.Replace("$", "");
string oFooter = Footer.Replace("$", "");
str = str.Remove(StartIndex, (EndIndex - StartIndex) + 1);
str = str.Remove(str.IndexOf(Footer) + Footer.Length + 5, 18);
str = str.Insert(str.IndexOf(Footer) - 1, " align= right");
str = str.Replace(Header, oHeader);
str = str.Replace(Footer, oFooter);
}
This function takes fields one by one to generate its Footer template by converting the rendered HTML code of the Template Column that I added in the DetailsView
Source Code.
private static void AdjustGrid(ref string str, string GridName)
{
int StartIndex = str.IndexOf(GridName);
if (StartIndex == -1) return;
int EndIndex = str.IndexOf("</table>", StartIndex);
int ColIndex = str.IndexOf("<td colspan=\"2\">", StartIndex);
if (ColIndex == -1) return;
if (ColIndex < EndIndex)
{
str = str.Remove(ColIndex, 16);
str = str.Insert(ColIndex, "<td colspan=\"3\">");
}
ColIndex = str.IndexOf("<td colspan=\"2\">", StartIndex);
if (ColIndex == -1) return;
if (ColIndex < EndIndex)
{
str = str.Remove(ColIndex, 16);
str = str.Insert(ColIndex, "<td colspan=\"3\">");
}
}
This function adjusts the general figure of detailsview
because of the problems that result from the modifications to the rendered HTML code.