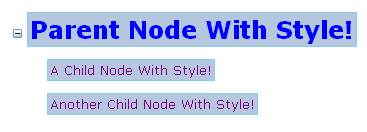
Introduction
This article will show how to extend the ASP.NET TreeView control to apply Cascading Style Sheets to tree nodes as well as add the functionality of storing custom attributes per node.
Background
There are lots of useful make-life-easy functionalities that the built-in ASP.NET TreeView
control lacks that are very noticeable especially when you are working with third party components. Functionalities like adding custom attributes or properties to a node other then the node text and Value, a built-in data binding supporting auto-binding from a single self-referencing table with ID -> Parent ID relation, and adding a custom style or template to nodes or globally to a tree view.
Attributes and node customization are addressed in this article, and hopefully I will address auto-binding in a later article or at least give it a try!
Customizing Tree Nodes
Since we are changing the way the System.Web.UI.WebControls.TreeNode
Text
property is being rendered, the first thing we need to do is to create a class that inherits TreeNode
and override the RenderPreText
and RenderPostText
methods. In the RenderPreText
method, we write out a Div
tag and add a class
attribute, setting its value with the cssClass
property.
protected override void RenderPreText(HtmlTextWriter writer)
{
writer.AddAttribute(HtmlTextWriterAttribute.Class, cssClass );
writer.RenderBeginTag(HtmlTextWriterTag.Div);
base.RenderPreText(writer);
}
In the RenderPostText
method, we end the Div
tag.
protected override void RenderPostText(HtmlTextWriter writer)
{
writer.RenderEndTag();
base.RenderPostText(writer);
}
Adding Custom Attributes
In a certain type of scenario, you many need to store data specific to nodes that the TreeNode
class does not provide. You can store any number of attributes in the collection as name/value pairs using the NameValueCollection
collection.
public NameValueCollection Attributes
{
get
{
return this._Attributes;
}
set
{
this._Attributes = value;
}
}
Using the Code
private CustomTreeNode CreateNode(string NodeText, string NodeStyle)
{
CustomTreeNode oNode = new CustomTreeNode();
oNode.Text = NodeText;
oNode.cssClass = NodeStyle;
oNode.Attributes["CustomAttribute1"] = "1";
oNode.Attributes["CustomAttribute2"] = "2";
oNode.Attributes["CustomAttribute3"] = "3";
return oNode;
}
Simple create an instance of the CustomTreeNode
class and set the cssClass
or Attributes
properties if applicable. I also provide a recursive function in the demo application that loops through the tree and displays node text and its relative attributes.
Points of Interest
I hope you find this article interesting and simple. I want to thank Danny Chen for discussing node customization which I found very useful in writing this article.