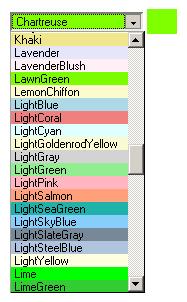
Introduction
This article demonstrates how to read system colors and to color-in each row of a drop down list. In this example, I will show:
- How to get a list of color names from the
System.Drawing.KnownColor
enumeration - How to exclude system environment colors, e.g. "Active Border"
- How to assign color to each row of the drop down list
Background
I was asked to make an admin tool where different types of appointments can be set. These appointments would be of unique color and the admin could change the color of the appointment type at any time.
I began to think of a drop down list where the name of the colors would be displayed and the background of that row would be of that color. With a view to that, I searched the web and couldn't find any solutions for a long time. Then I finally found a solution that seemed much more complex than needed, involving a database. So I tried to find an easier solution.
Using the code
I am taking a drop down list control named ddlMultiColor
to show the color names and color. I am using a <div>
tag, msgColor
, to show the color in a rectangular box.
<table>
<tr>
<td>
<asp:DropDownList ID ="ddlMultiColor"
OnSelectedIndexChanged="ddlMultiColor_OnSelectedIndexChanged"
runat="server" AutoPostBack="true">
</asp:DropDownList>
</td>
<td>
<div id="msgColor" runat="server">
</div>
</td>
</tr>
</table>
At the server side, we need the following namespaces imported for further coding.
using System;
using System.Web;
using System.Reflection;
using System.Drawing;
using System.Collections.Generic;
Let me show the Page.Load
event first. During this event, a drop down list is populated and color manipulation is performed.
protected void Page_Load(object sender, EventArgs e)
{
if (Page.IsPostBack == false)
{
populateDdlMultiColor();
colorManipulation();
}
}
Let us now go through the populateDdlMultiColor()
method.
private void populateDdlMultiColor()
{
ddlMultiColor.DataSource = finalColorList();
ddlMultiColor.DataBind();
}
Here goes the code for the finalColorList()
method.
private List finalColorList()
{
string[] allColors = Enum.GetNames(typeof(System.Drawing.KnownColor));
string[] systemEnvironmentColors =
new string[(
typeof(System.Drawing.SystemColors)).GetProperties().Length];
int index = 0;
foreach (MemberInfo member in (
typeof(System.Drawing.SystemColors)).GetProperties())
{
systemEnvironmentColors[index ++] = member.Name;
}
List finalColorList = new List();
foreach (string color in allColors)
{
if (Array.IndexOf(systemEnvironmentColors, color) < 0)
{
finalColorList.Add(color);
}
}
return finalColorList;
}
System.Drawing.KnownColor
is an enum that specifies the known system colors. I have filled the allColors
array with those system colors. In order to do this, I used one of the most basic enumeration features: the shared Enum.GetNames()
method. This method inspects an enumeration and provides an array of strings, one string for each value in the enumeration.
However, there lies a problem with this approach. It includes system environment colors -- e.g. "Active Border" -- in the array. In order to resolve the problem, I extracted the system environment color. I used the System.Reflection.MemberInfo
class, which obtains information about the attributes of a member and provides access to member metadata.
Here, I filled the systemEnvironmentColors
array with the properties of System.Drawing.SystemColors
. Then I created a generic list named finalColorList
in which I took only those colors that are in KnownColor
, but not in system environment color. The list, finalColorList
, is then bound to the drop down list named ddlMultiColor
. At this point, we have a drop down list full of color names. Let us manipulate those colors.
private void colorManipulation()
{
int row;
for (row = 0; row < ddlMultiColor.Items.Count - 1; row++)
{
ddlMultiColor.Items[row].Attributes.Add("style",
"background-color:" + ddlMultiColor.Items[row].Value);
}
ddlMultiColor.BackColor =
Color.FromName(ddlMultiColor.SelectedItem.Text);
}
Each row of the drop down list is assigned the style
attribute to the background-color
by the name of the color of that row. Then the background color of the drop down list is assigned by the selected color. At the OnSelectedIndexChanged
event of the dropdown list, the following code snippets are added so that the selected color name is highlighted and the <div>
tag named msgColor
can show the rectangular image of the selected color.
protected void ddlMultiColor_OnSelectedIndexChanged(object sender,
EventArgs e)
{
ddlMultiColor.BackColor = Color.FromName(ddlMultiColor.SelectedItem.Text);
colorManipulation();
ddlMultiColor.Items.FindByValue(ddlMultiColor.SelectedValue).Selected =
true;
msgColor.Attributes.Add("style", "background:" +
ddlMultiColor.SelectedItem.Value + ";width:30px;height:25px;");
}
Points of interest
So, we have learned how to get colors from System.Drawing
, exclude system environment colors from those, bind the list to a drop down list and assign colors to each row according to the name of the color. That's it! Happy coding!!
History