Introduction
MSXML, a window component shipped with Windows / Office and other Microsoft products, exists in all Windows platforms.
But there are different versions of MSXML, from 2.6 to 6.0, which causes a lot of problems.
This is a wrapping class library for MSXML 3.0/4.0/5.0/6.0
consisting of resolving the above problem -- you don't need to worry about the available MSXML version on different machines any more.
Also it provides an easy-to-use
interface -- each node in the XML is considered an object and you can use some code like below to locate a node.
xml.GetRoot()->GetChild(_T("a"))->GetChild(_T("b"))->GetChild(_T("c"))
Sample Code
Please find the sample code in the demo project.
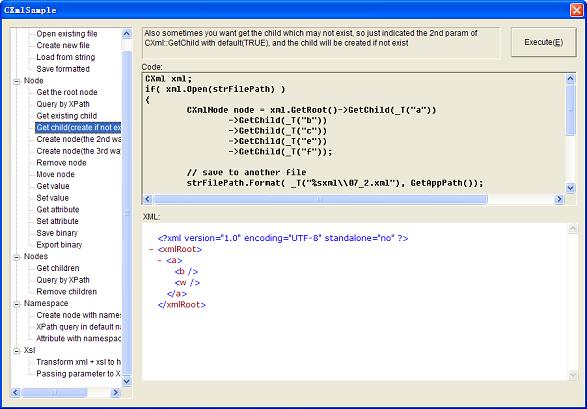
Using the Code
Copy all the files in the /CXml/*.* directory and add them into your project.
#include "Xml.h"
using namespace JWXml;
Here, I have added a namespace for the classes, you can change it as you like.
MSXML Version
The class will try to choose the version of MSXML in the following order:
hr = (hr == S_OK) ? hr : m_pDoc.CreateInstance( __uuidof(MSXML2::DOMDocument60) );
hr = (hr == S_OK) ? hr : m_pDoc.CreateInstance( __uuidof(MSXML2::DOMDocument30) );
hr = (hr == S_OK) ? hr : m_pDoc.CreateInstance( __uuidof(MSXML2::DOMDocument50) );
hr = (hr == S_OK) ? hr : m_pDoc.CreateInstance( __uuidof(MSXML2::DOMDocument40) );
hr = (hr == S_OK) ? hr : m_pDoc.CreateInstance( __uuidof(MSXML2::DOMDocument26) );
hr = (hr == S_OK) ? hr : m_pDoc.CreateInstance( __uuidof(MSXML2::DOMDocument) );
Classes Overview
CXml
class CXml
{
friend class CXsl;
public:
CXml(void);
~CXml(void);
protected:
MSXML2::IXMLDOMDocument2Ptr m_pDoc;
CString m_strFilePath;
MSXML_VERSION m_emVersion;
std::map< CString, CString> m_mpNamespace;
BOOL CreateInstance(void);
public:
BOOL Open(LPCTSTR lpszXmlFilePath);
BOOL Create( LPCTSTR lpszRootName = _T("xmlRoot")
, LPCTSTR lpszPrefix = _T("")
, LPCTSTR lpszNamespaceURI = _T("")
);
BOOL LoadXml(LPCTSTR lpszXmlContent);
BOOL Save(LPCTSTR lpszFilePath);
BOOL SaveWithFormatted(LPCTSTR lpszFilePath = NULL,
LPCTSTR lpszEncoding = _T("UTF-8"));
void Close(void);
CString GetXmlFile(void) const;
CString Base64Encode( LPBYTE pBuf, ULONG ulSize);
BOOL Base64Decode( CString strIn, LPBYTE pBuf, LONG & lSize);
void AddSelectionNamespace( LPCTSTR lpszPrefix, LPCTSTR lpszURI);
CXmlNodePtr GetRoot(void);
CXmlNodePtr SelectSingleNode(LPCTSTR lpszPath);
CXmlNodesPtr SelectNodes(LPCTSTR lpszPath);
CXmlNodePtr CreateNode(LPCTSTR lpszName
, LPCTSTR lpszPrefix = _T("")
, LPCTSTR lpszNamespaceURI = _T("")
);
MSXML_VERSION GetVersion(void) const;
};
CXmlNode
class CXmlNode
{
friend class CXml;
friend class CXmlNode;
friend class CXmlNodes;
protected:
MSXML2::IXMLDOMNodePtr m_pNode;
CXmlNode( MSXML2::IXMLDOMNodePtr pNode);
BOOL _GetValue(CString & strValue) const;
BOOL _SetValue(CString & strValue) const;
BOOL _GetAttribute( CString & strName, CString & strValue) const;
BOOL _SetAttribute( CString & strName IN
, CString & strValue IN
, CString & strPrefix IN
, CString & strNamespaceURI IN
) const;
public:
CXmlNode(void);
CXmlNode(const CXmlNode & refNode IN);
CXmlNode(const CXmlNodePtr pNode IN);
~CXmlNode(void);
CXmlNodePtr operator = (CXmlNodePtr pNode);
CXmlNode & operator = (const CXmlNode & refNode);
BOOL IsNull(void) const; CString GetName(void) const; CXmlNode & Detach(void); void Release(void);
CXmlNodePtr GetChild( CString strName, BOOL bBuildIfNotExist = TRUE);
CXmlNodePtr NewChild( CString strName );
CXmlNodePtr GetParent(void);
CXmlNodesPtr GetChildren();
void AttachChild( CXmlNodePtr & pChildNode);
void AttachChild( CXmlNode & refChildNode);
BOOL HasChildren(void);
BOOL RemoveChildren(void);
CString GetAttribute( CString strName, LPCTSTR lpszDefault = NULL) const;
bool GetAttribute( CString strName, bool bDefault) const;
int GetAttribute( CString strName, int nDefault) const;
long GetAttribute( CString strName, long lDefault) const;
__int64 GetAttribute( CString strName, __int64 llDefault) const;
float GetAttribute( CString strName, float fDefault) const;
double GetAttribute( CString strName, double dDefault) const;
DWORD GetAttribute( CString strName, DWORD dwDefault) const;
BOOL SetAttribute( CString strName, LPCTSTR lpszValue ,
CString strPrefix = _T(""), CString strNamespaceURI = _T(""));
BOOL SetAttribute( CString strName, bool bValue ,
CString strPrefix = _T(""), CString strNamespaceURI = _T(""));
BOOL SetAttribute( CString strName, int nValue ,
CString strPrefix = _T(""), CString strNamespaceURI = _T(""));
BOOL SetAttribute( CString strName, long lValue ,
CString strPrefix = _T(""), CString strNamespaceURI = _T(""));
BOOL SetAttribute( CString strName, __int64 llValue ,
CString strPrefix = _T(""), CString strNamespaceURI = _T(""));
BOOL SetAttribute( CString strName, float fValue ,
CString strPrefix = _T(""), CString strNamespaceURI = _T(""));
BOOL SetAttribute( CString strName, double dValue ,
CString strPrefix = _T(""), CString strNamespaceURI = _T(""));
BOOL SetAttribute( CString strName, DWORD dwValue ,
CString strPrefix = _T(""), CString strNamespaceURI = _T(""));
BOOL RemoveAttribute( CString strName );
CString GetValue( LPCTSTR lpszDefault = NULL ) const;
bool GetValue( bool bDefault ) const;
int GetValue( int nDefault) const;
long GetValue( long lDefault) const;
__int64 GetValue( __int64 llDefault) const;
float GetValue( float fDefault) const;
double GetValue( double dDefault) const;
DWORD GetValue( DWORD dwDefault) const;
BOOL SetValue( LPCTSTR lpszValue );
BOOL SetValue( bool bValue );
BOOL SetValue( int nValue );
BOOL SetValue( long lValue );
BOOL SetValue( __int64 llValue );
BOOL SetValue( float fValue );
BOOL SetValue( double dValue );
BOOL SetValue( DWORD dwValue );
CXmlNodePtr SelectSingleNode(LPCTSTR lpszPath);
CXmlNodesPtr SelectNodes(LPCTSTR lpszPath);
CString GetOuterXml(void) const;
CString GetInnerXml(void) const;
};
CXmlNodes
class CXmlNodes
{
friend class CXml;
friend class CXmlNode;
friend class CXmlNodes;
public:
~CXmlNodes(void);
CXmlNodes(void);
CXmlNodes( const CXmlNodes & refNodes );
CXmlNodes( CXmlNodesPtr pNodes );
CXmlNodesPtr operator = (CXmlNodesPtr pNodes);
CXmlNodes & operator = (const CXmlNodes & refNodes);
CXmlNodePtr operator[] ( LONG lIndex );
CXmlNodePtr operator[] ( LPCTSTR lpszName );
LONG GetCount(void);
void Release(void);
CXmlNodePtr GetItem( LONG nIndex );
CXmlNodePtr GetItem( LPCTSTR lpszName );
protected:
CXmlNodes(MSXML2::IXMLDOMNodeListPtr pNodeList);
MSXML2::IXMLDOMNodeListPtr m_pNodeList;
};
CXsl
class CXsl
{
public:
CXsl(void);
~CXsl(void);
BOOL Open(LPCTSTR lpszXslFilePath);
void Close(void);
BOOL TransformToFile( CXml & objXml, LPCTSTR lpszFilePath);
BOOL AddParameter( LPCTSTR lpszParamName, LPCTSTR lpszParamValue,
LPCTSTR lpszNamespaceURI = NULL);
protected:
MSXML2::IXSLTemplatePtr m_pIXSLTemplate;
MSXML2::IXMLDOMDocument2Ptr m_pStyleSheet;
MSXML2::IXSLProcessorPtr m_pIXSLProcessor;
};
History
- v2.0
- v2.1
- Added
LoadXml
method - Added
GetVersion
method - Added const for
GetXXX
methods - Defined
ASSERT
as ATLASSERT
for ATL - Defined
TRACE
as ATLTRACE
for ATL
- V2.2
- Added the parameter
lpszRootName
for CXml::Open
- Removed
CXml::GetLastError
- Added
CXml::AddNamespace
- Added two new overrides for
CXml::CreateNode
with namespace support
- V3.0
- Added another copy constructor for
CXmlNode
and CXmlNodes
- Added
const
modifier for some variables - Added
CXmlNode::GetInnerXml
- Added
CXmlNode::GetOuterXml
- Added
CXml::Create
- Changed the MSXML version for
Create
to 6.0 -> 3.0 -> 5.0 -> 4.0 - Added namespace support for attributes
- Added a new class named
CXsl
- V3.1
- Add method
CXml::SaveWithFormatted
(Thanks to roel_) - Reuse
CXml::SaveStreamToFile
in CXsl::TransformToFile
- Add
CXsl::AddParameter
to allow passing parameters to XSLT - Use
std::tr1::shared_ptr
if exists instead of std::auto_ptr
- Change namespace from
Generic
to JWXml
- V3.2
- Bug fix for the
Create
method usage in demo. - Upgrade to VS2010