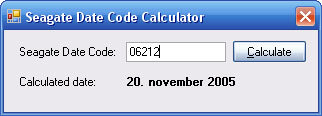
Introduction
The code described in this article helps users convert Seagate's (the hard drive manufacture) date code to a DateTime
value.
This article is based on this documentation.
Background
I had to check some hard drives for warranty, and found myself checking very old hard drives.
The code
Here is the actual code involved:
Class Variables
private DateTime datecalc;
private string _datecode;
Class Properties
public string DateCode
{
get { return _datecode; }
set
{
int _CodeYear = 0;
byte _CodeWeek = 0;
byte _CodeDayOfWeek = 0;
_datecode = value;
if (_datecode.Length == 5)
{
_CodeWeek = Convert.ToByte(_datecode.Substring(2, 2));
_CodeDayOfWeek = Convert.ToByte(_datecode.Substring(4));
}
else if (_datecode.Length == 4)
{
_CodeWeek = Convert.ToByte(_datecode.Substring(2, 1));
_CodeDayOfWeek = Convert.ToByte(_datecode.Substring(3));
}
if (_datecode.Length == 4 || _datecode.Length == 5)
{
_CodeWeek--;
_CodeDayOfWeek--;
_CodeYear = (2000 + Convert.ToInt32(_datecode.Substring(0, 2)) - 1);
datecalc = new DateTime(_CodeYear, 7, 1);
datecalc.AddDays(-1);
do { datecalc = datecalc.AddDays(1); }
while (datecalc.DayOfWeek != DayOfWeek.Saturday);
datecalc = datecalc.AddDays((_CodeWeek * 7) + _CodeDayOfWeek);
}
}
}
public DateTime CalculatedDate
{
get { return datecalc; }
}
Class Constructors
public SeagateDateCodeCalc()
{
}
public SeagateDateCodeCalc(string dateCode)
{
this.DateCode = dateCode;
}
History
This is my first submission here, so let me know if I am missing anything.