Introduction
This post will help you to search multiple cell values of the provided columns in a datagridview
.
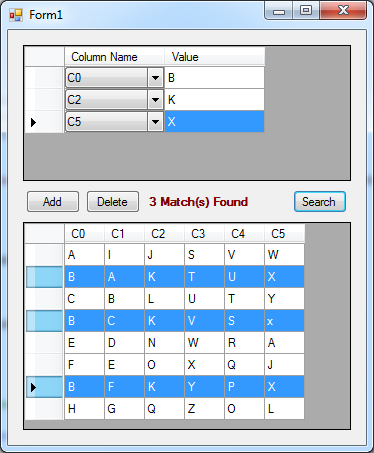
The Add/Delete button adds/deletes rows in the top datagridview
, were the user can enter the search criteria.
The bottom datagridview
is the grid in which we perform search.
The first column of the top datagridview
lists all the column names of the bottom datagridview
. The user has to enter the search value in the second column.
This property gets the search criteria from the top datagridview
:
private Dictionary<string, string> SearchList
{
get
{
Dictionary<string, string> searchList = new Dictionary<string, string>();
foreach (DataGridViewRow dgvRow in dataGridViewSearch.Rows)
{
string colName = Convert.ToString(dgvRow.Cells["ColumnName"].Value);
if (colName != string.Empty)
{
string val = Convert.ToString(dgvRow.Cells["Value"].Value);
searchList.Add(colName, val);
}
}
return searchList;
}
}
This routine performs the search operation and returns the matched datagridviewrow
s as list:
private List<DataGridViewRow> PerformSearch
(DataGridView dgView, Dictionary<string, string> searchList)
{
bool rowFound = false;
List<DataGridViewRow> dgvSearchResult = new List<DataGridViewRow>();
foreach (DataGridViewRow dgvRow in dgView.Rows)
{
foreach (string colName in searchList.Keys)
{
string val1 = Convert.ToString(dgvRow.Cells[colName].Value);
string val2 = Convert.ToString(searchList[colName]);
if (string.Compare(val1, val2, true) == 0)
rowFound = true;
else
{
rowFound = false;
break;
}
}
if (rowFound)
dgvSearchResult.Add( dgvRow);
}
return dgvSearchResult;
}
Search Button Click event is defined below:
private void btnSearch_Click(object sender, EventArgs e)
{
lblInfo.Text = string.Empty;
List<string> colNameList = new List<string>();
foreach (DataGridViewRow dgvRow in dataGridViewSearch.Rows)
if (!colNameList.Contains(Convert.ToString(dgvRow.Cells["ColumnName"].Value)))
colNameList.Add(Convert.ToString(dgvRow.Cells["ColumnName"].Value));
else
{
MessageBox.Show("Invalid Search criteria", "Search",
MessageBoxButtons.OK, MessageBoxIcon.Information);
return;
}
List<DataGridViewRow> resultRows = PerformSearch(dataGridView1, SearchList);
if (this.dataGridView1.SelectedCells.Count > 0)
foreach (DataGridViewCell dgvCell in this.dataGridView1.SelectedCells)
dgvCell.Selected = false;
if (resultRows.Count > 0)
{
foreach (DataGridViewRow dgvRow in resultRows)
dataGridView1.Rows[dgvRow.Index].Selected = true;
}
lblInfo.Text = resultRows.Count.ToString() + " Match(s) Found";
}
The attached source code is in VS 2010.
Thanks,
Balu