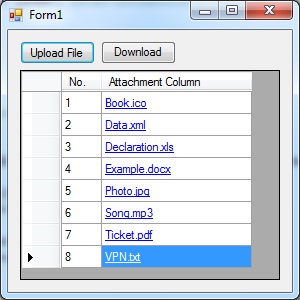
Introduction
This post helps to upload any file into a datagridview
cell and also download it from the datagridview
cell. In the attached project file, I have declared a model variable Dictionary<int, byte[]> _myAttachments
which stores the attachments as Byte
array, and key stores the rowIndex
.
Dictionary<int, byte[]> _myAttachments = new Dictionary<int, byte[]>();
Select the attachment cell and click on the ‘Upload File’ button. It will pop up a FileDialog
for the user to select the required file which is to be uploaded.
The following routine uploads the user selected file and shows the file name in the provided dataGridViewCell
.
private void UploadAttachment(DataGridViewCell dgvCell)
{
using (OpenFileDialog fileDialog = new OpenFileDialog())
{
fileDialog.CheckFileExists = true;
fileDialog.CheckPathExists = true;
fileDialog.Filter = "All Files|*.*";
fileDialog.Title = "Select a file";
fileDialog.Multiselect = false;
if (fileDialog.ShowDialog() == DialogResult.OK)
{
FileInfo fileInfo = new FileInfo(fileDialog.FileName);
byte[] binaryData = File.ReadAllBytes(fileDialog.FileName);
dataGridView1.Rows[dgvCell.RowIndex].Cells[1].Value = fileInfo.Name;
if (_myAttachments.ContainsKey(dgvCell.RowIndex))
_myAttachments[dgvCell.RowIndex] = binaryData;
else
_myAttachments.Add(dgvCell.RowIndex, binaryData);
}
}
}
The DownloadAttachment
routine can be called on cell double click or the ‘Download’ button click event.
private void DownloadAttachment(DataGridViewCell dgvCell)
{
string fileName = Convert.ToString(dgvCell.Value);
if (fileName == string.Empty)
return;
FileInfo fileInfo = new FileInfo(fileName);
string fileExtension = fileInfo.Extension;
byte[] byteData = null;
using (SaveFileDialog saveFileDialog1 = new SaveFileDialog())
{
saveFileDialog1.Filter = "Files (*" + fileExtension + ")|*" + fileExtension;
saveFileDialog1.Title = "Save File as";
saveFileDialog1.CheckPathExists = true;
saveFileDialog1.FileName = fileName;
if (saveFileDialog1.ShowDialog() == DialogResult.OK)
{
byteData = _myAttachments[dgvCell.RowIndex];
File.WriteAllBytes(saveFileDialog1.FileName, byteData);
}
}
}