Introduction
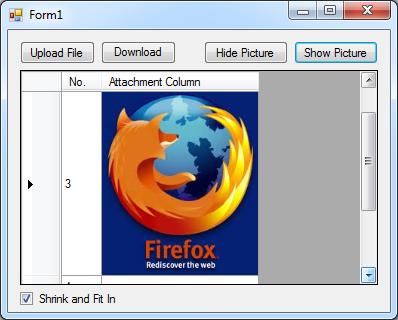
Image 1
This post is regarding how to show and hide Image within the DataGridViewCell
s. The user can also opt to view the image within the available display area.
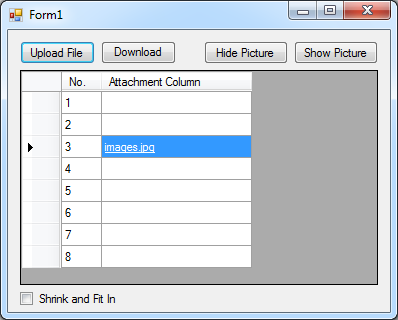
Image 2
The following routine helps to show the image in the cell. The Image in the cell is saved as bytearray which is converted back to image, then replaces the DataGridViewTextboxCell
with an DataGridViewImageCell
where the image cell is loaded with the image.
private void ShowPicture(DataGridView myGrid, DataGridViewCell dgvCell, bool fillIn)
{
if (!_myAttachments.ContainsKey(dgvCell.RowIndex) ||
_myAttachments[dgvCell.RowIndex] == null)
return;
byte[] byteData = _myAttachments[dgvCell.RowIndex];
Image returnImage = null;
try
{
MemoryStream ms = new MemoryStream(byteData);
returnImage = Image.FromStream(ms, false, true);
}
catch
{
MessageBox.Show("Not an Image File", "Show Picture",
MessageBoxButtons.OK, MessageBoxIcon.Warning);
return;
}
DataGridViewImageCell dgImageCol = new DataGridViewImageCell();
if (fillIn)
returnImage =ResizeImageToFitInsideTheGrid(returnImage, myGrid);
dgImageCol.Value = returnImage;
if (myGrid.Columns[dgvCell.ColumnIndex].Width < returnImage.Width)
myGrid.Columns[dgvCell.ColumnIndex].Width = returnImage.Width;
if (myGrid.Rows[dgvCell.RowIndex].Height < returnImage.Height)
myGrid.Rows[dgvCell.RowIndex].Height = returnImage.Height;
myGrid[dgvCell.ColumnIndex, dgvCell.RowIndex] = dgImageCol;
}
Hide the Picture routine, replace the DataGridViewImageCell
with a DataGridViewTextboxCell
and load the file name in it.
private void HidePicture(DataGridView myGrid, DataGridViewCell dgvCell)
{
Type tp = dgvCell.GetType();
if (tp.Name == "DataGridViewImageCell")
{
DataGridViewTextBoxCell dgTxtCol = new DataGridViewTextBoxCell();
dgTxtCol.Value = _myAttachmentFileNames[dgvCell.RowIndex];
myGrid[dgvCell.ColumnIndex, dgvCell.RowIndex] = dgTxtCol;
myGrid.AutoSizeRowsMode = DataGridViewAutoSizeRowsMode.AllCells;
}
}
Resizing the Image
- Image with large
Width
& Height
- Image with large
Width
- Image with large
Height
- Image with small
Width
private Image ResizeImageToFitInsideTheGrid(Image returnImage, DataGridView myGrid)
{
int dgvDisplayHeight = Convert.ToInt32
((myGrid.DisplayRectangle.Height - myGrid.ColumnHeadersHeight) * .95);
int dgvDisplayWidth = Convert.ToInt32
((myGrid.DisplayRectangle.Width - myGrid.RowHeadersWidth) * .95);
if (returnImage.Width >= dgvDisplayWidth && returnImage.Height >= dgvDisplayHeight)
{
int h = Convert.ToInt32(dgvDisplayHeight);
int w = Convert.ToInt32(h * (Convert.ToDouble(returnImage.Width) /
Convert.ToDouble(returnImage.Height)));
if (w > dgvDisplayWidth)
{
w = Convert.ToInt32(dgvDisplayWidth);
h = Convert.ToInt32(w * (Convert.ToDouble(returnImage.Height) /
Convert.ToDouble(returnImage.Width)));
}
returnImage = ResizeImage(returnImage, w, h);
}
else if (returnImage.Width <= dgvDisplayWidth && returnImage.Height >=
dgvDisplayHeight)
{
int h = Convert.ToInt32(dgvDisplayHeight);
int w = Convert.ToInt32(h * (Convert.ToDouble(returnImage.Width) /
Convert.ToDouble(returnImage.Height)));
returnImage = ResizeImage(returnImage, w, h);
}
else if (returnImage.Width >= dgvDisplayWidth &&
returnImage.Height <= dgvDisplayHeight)
{
int w = Convert.ToInt32(dgvDisplayWidth);
int h = Convert.ToInt32(w *
(Convert.ToDouble(returnImage.Height) / Convert.ToDouble(returnImage.Width)));
returnImage = ResizeImage(returnImage, w, h);
}
return returnImage;
}
private Image ResizeImage(Image actualImage, int width, int height)
{
Bitmap bitmap = new Bitmap(width, height);
using (Graphics graphics = Graphics.FromImage((Image)bitmap))
graphics.DrawImage(actualImage, 0, 0, width, height);
return (Image)bitmap;
}