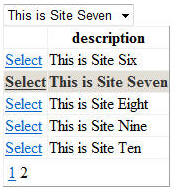
Introduction
When using declarative data binding, you will sometimes run into a situation where you need to programmatically set the selection on a GridView
control. The problem you may encounter is that the GridView
control can only be set by its row index value. Usually, you only possess the key value.
This article demonstrates using an Extension Method to allow you to programmatically set the selected value on a GridView
using the key value on the GridView
.
The Code
In this example, the dropdown control and the GridView
control are bound to the same data source. When the dropdown control is changed, this line is called:
GridView1.SetRowValueValueByKey(DropDownList1.SelectedValue);
This calls the SetRowValueValueByKey
Extension Method to set the selected value on the GridView
. Below is the complete code for the code-behind:
using System;
using System.Collections;
using System.Configuration;
using System.Data;
using System.Linq;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.HtmlControls;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Xml.Linq;
namespace UsingDataKeys
{
public partial class GridViewSelectionUsingDataKeys : System.Web.UI.Page
{
protected void DropDownList1_SelectedIndexChanged(object sender, EventArgs e)
{
GridView1.SetRowValueValueByKey(DropDownList1.SelectedValue);
}
}
public static class GridViewExtensions
{
public static void SetRowValueValueByKey(this GridView GridView,
string DataKeyValue)
{
int intSelectedIndex = 0;
int intPageIndex = 0;
int intGridViewPages = GridView.PageCount;
for (int intPage = 0; intPage < intGridViewPages; intPage++)
{
GridView.PageIndex = intPage;
GridView.DataBind();
for (int i = 0; i < GridView.DataKeys.Count; i++)
{
if (Convert.ToString(GridView.DataKeys[i].Value) == DataKeyValue)
{
intSelectedIndex = i;
intPageIndex = intPage;
break;
}
}
}
GridView.PageIndex = intPageIndex;
GridView.SelectedIndex = intSelectedIndex;
GridView.DataBind();
}
}
}
Points of Interest
- You must have the
DataValueField
set on the GridView
for this to work. - Currently, this code does not handle composite keys; however, it can be modified to do so.