Introduction
jQuery is a fast, concise, JavaScript library that simplifies how you traverse HTML documents, handle events, perform animations, and add AJAX interactions to your web pages. jQuery is designed to change the way that you write JavaScript.
In this article, I'll build a sliding JavaScript menu using jQuery.
Using the code
First, you must reference the jQuery library which can be downloaded form here. Then, you can make the menu HTML page as in the following code:
<html xmlns="http://www.w3.org/1999/xhtml" >
<head>
<title>JQuery Sample Menu</title>
<link rel="Stylesheet" type="text/css" href="styles/navigation.css" />
<script type="text/javascript" language="javascript"
src="scripts/jquery-1.2.3.js"></script>
<script type="text/javascript" language="javascript"
src="scripts/CustomMenu.js"></script>
</head>
<body>
<h2>This is a sample menu using JQuery</h2>
<h4>Try to click on the clickable items in the menu to see the animation</h4>
<ul class="menu">
<li>- Parent item with no children</li>
<li>
- Item 1 with children
<ul >
<li>Nested item 1</li>
<li>Nested item 2</li>
</ul>
</li>
<li>
- Item 2 with children
<ul >
<li>Nested item 1</li>
<li>Nested item 2</li>
<li>Nested item 3</li>
<li>Nested item 4</li>
</ul>
</li>
<li>
- Item 3 with children
<ul>
<li>Nested item 1</li>
<li>Nested item 2</li>
<li>Nested item 3</li></ul>
</li>
</ul>
</body>
</html>
Now, for the JavaScript magic! Just use the following JavaScript piece of code:
$(function()
{
$('li:has(ul)')
.click(function(event){
if (this == event.target) {
$('li:has(ul)').children().hide('slow');
$(this).children().animate({opacity:'toggle',height:'toggle'},'slow');
}
return false;
}
)
.css({cursor:'pointer'})
.children().hide();
}
);
Now you can have the sliding effect without so much JavaScript coding.
Menu at start:
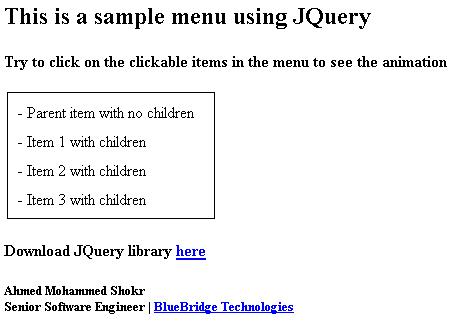
Menu in action:

Points of Interest
jQuery is a very useful JavaScript library, you should use it! I'll post about JQuery selectors and other operators soon.
I hope that helped.