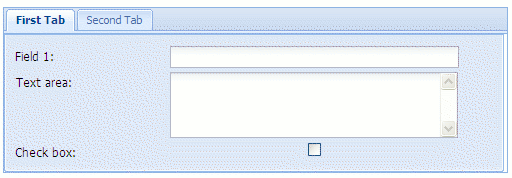
Introduction
ExtJS is a really cool JavaScript library for generating web GUIs. Everything works really nicely when all you want are simple forms, but it generally doesn't take long before you want to control the rendered JavaScript based on some server-based logic (i.e., only show these fields to "admins", or load values from a SQL database based on the selected ID and show them in the form). Pretty soon, you are wrapping the JavaScript in PHP control structures and embedded little snippets of PHP to set values. Then, what do you know, your code looks like unmaintainable rubbish. Not to mention that if you are an Emacs user (like me), it messes up all the nice syntax highlighting and auto-indenting. Hence, I decided to invest an hour or two in creating a PHP-wrapper that would allow me to write PHP but render ExtJS JavaScript. It turns out it is pretty simple, as my code will show.
Background
ExtJS code uses hierarchical JavaScript object structures where the objects are specialised by setting properties. A similar structure of objects can be specified in PHP, and now that PHP 5 supports the magic methods of __get
and __set
, that makes it is easy to set arbitrary properties of an object. This is the key to why the mapping ends up being simple.
Using the code
The included Zip file contains a file, demo.php, that generates the screenshot shown at the start of this article.
Ext objects are mapped to the PHP class ExtObject
. A few simple examples show the symmetry:
The PHP code:
$tab = new ExtObject('Ext.TabPanel');
$tab->renderTo = "tabs";
$tab->activeTab = 0;
$tab->autoHeight = true;
$tab->items = Array(
new ExtObject(null, Array('title'=>'First Tab',
'contentEl'=>'tab1',
'autoHeight'=>true
)
),
new ExtObject(null, Array('title'=>'Second Tab',
'contentEl'=>'tab2',
'autoHeight'=>true
)
)
);
Generates the JavaScript:
var tabs = new Ext.TabPanel({
renderTo: 'tabs',
activeTab: 0,
autoHeight: true,
items: [
{
title: 'First Tab',
contentEl: 'tab1',
autoHeight: true
},
{
title: 'Second Tab',
contentEl: 'tab2',
autoHeight: true
}
]
});
And, the following PHP code:
$tab1->items = Array(new ExtObject(null, Array('fieldLabel' => 'Field 1',
'value' => ''
)
),
new ExtObject('Ext.form.TextArea', Array('fieldLabel' => 'Text area',
'value'=> ''
)
),
new ExtObject('Ext.form.Checkbox', Array('fieldLabel' => 'Check box',
'value'=> true
)
)
);
Generates the following JavaScript:
var tab1 = new Ext.FormPanel({
labelWidth: 150,
url: 'part.submit.php',
frame: true,
bodyStyle: 'padding: 5px 5px 0',
width: 500,
defaults: {
width: 290
},
defaultType: 'textfield',
items: [
{
fieldLabel: 'Field 1',
value: ''
},
new Ext.form.TextArea({
fieldLabel: 'Text area',
value: ''
}),
new Ext.form.Checkbox({
fieldLabel: 'Check box',
value: true
})
]
});
ExtObject
also implements a method SetProperties(...)
that allows an associative array to be used to supply parameters. An example is shown below:
$tab1->SetProperties(Array('labelWidth' => 150,
'url' => 'part.submit.php',
'frame' => true,
'bodyStyle' => 'padding: 5px 5px 0',
'width' => 500,
'defaults' => new ExtObject(null, Array('width' => 290)),
'defaultType' => 'textfield'
)
);
A second object ExtPage
is supplied to generate all the surrounding HTML code that is required. It has three properties:
- The page title
- The JavaScript code to be included in the
<head>
tag - the bulk to the ExtJS code - Any HTML that should be included in the
<body>
tag
An example is shown below:
$page = new ExtPage('Demonstration');
$page->js = "
Ext.onReady(function(){
Ext.QuickTips.init();
";
$page->js .= $tab->render('tabs');
$page->js .= $tab1->render('tab1');
$page->js .= "tab1.render('tab1');
});
";
$page->body = "<div id='tabs'>
<div id='tab1' class='x-tab x-hide-display'></div>
<div id='tab2' class='x-tab x-hide-display'>A tab</div>
</div>
";
$page->render();
Points of interest
The crux of the code is the JSRender(...)
method in the ExObject
object. It descends the PHP object structure recursively to generate the equivalent JavaScript code. The static variable ExtObject::$indent
is used to provide reasonable indenting for the output JavaScript code to make it easier to follow.
History
- 3-Jun-08 PJC: Fixed the ExtJS link as per reader's comment - thanks admirm :-)