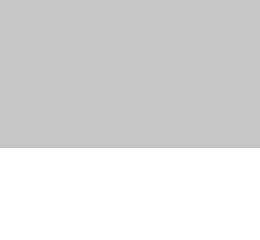
Introduction
I’m going to introduce here a JavaScript Context Menu control. It is a cross-browser JavaScript class that runs on various browsers except Opera as Opera doesn’t support the oncontextmenu
event.
Background
Around one and a half years ago, I had created a context menu to display on a GridView
control to perform some actions in a project. That project was IE based. So, the context menu was IE specific, and not cross-browser compatible. Also, it was a mixture of JavaScript and HTML: I had created its layout using the HTML table
and div
elements and displayed it using JavaScript. I wanted to develop it as a cross-browser JavaScript class. So, I started its development, and after spending some time on the Internet and books, I succeeded.
Constructor
The constructor of the context menu class takes an argument of the type Object Literal. The definition of the Object Literal argument for this control is given below:
var Arguments = {
Base: _Base,
Width: _Width,
FontColor: _FontColor,
HoverFontColor: _HoverFontColor,
HoverBackgroundColor: _HoverBackgroundColor,
HoverBorderColor: _HoverBorderColor,
OnClickEventListener: _OnClickEventListener
};
var Arguments = {
Base: document.getElementById('div'),
Width: 200,
FontColor: black,
HoverFontColor: white,
HoverBackgroundColor: blue,
HoverBorderColor: orange,
OnClickEventListener: ClickEventHandler
};
You can assign each property of the Object Literal argument to null
. In this case, each property will acquire its default value as:
var Arguments = {
Base: null,
Width: null,
FontColor: null,
HoverFontColor: null,
HoverBackgroundColor: null,
HoverBorderColor: null,
OnClickEventListener: null
};
Methods
The context menu control has the following public methods:
AddItem(ImagePath, ItemText, IsDisabled, CommandName)
- Used to add a context menu item.
It takes four arguments:
ImagePath
: Path of the item image. ItemText
: The item text. IsDisabled
: Indicates whether the item is to be disabled or not. CommandName
: The command name of the item.
AddSeparatorItem()
: Used to add a separator item. Display(e)
: Used to display the context menu. Hide()
: Used to hide the context menu. Dispose()
: Used to destroy the context menu. GetTotalItems()
: Used to get the count of total items including the separator items.
Properties
The context menu control has only a public property. It only displays the current version of the context menu control. It does nothing else.
Version
: Displays the current version.
Events
The context menu control has only one event – The Click
event that fires when items other than the separator items are clicked.
Click
: Fires when an item is clicked.
The local anonymous method that responds to the Click
event (i.e., the event handler) has the following signature:
var EventHandlerName = function(Sender, EventArgs)
{
...
}
where Sender
is the reference of the element that raises the click
event (i.e., the tr
element) and EventArgs
is the Object Literal that contains the necessary information regarding Click
event. The EventArgs
Object Literal has the following definition:
var EventArgs = {
CommandName: _CommandName,
Text: _Text,
IsDisabled: _IsDisabled,
ImageUrl: _ImageUrl
};
Note that the Click
event handler is a C# style event handler.
Using the Control
Add a reference to the ContextMenu.js file in your web page, as:
<script type="text/javascript" src="JS/ContextMenu.js"></script>
Create a div
element on the web page, as:
<div id="div" style="width: 925px; height: 300px; background-color: silver;">
</div>
Now, create a script
tag in the head
section of the web page and add the following code in the window.onload
event:
<script type="text/javascript">
var oCustomContextMenu = null;
var oBase = null;
window.onload = function()
{
oBase = document.getElementById('div');
var Arguments = {
Base: oBase,
Width: 200,
FontColor: null,
HoverFontColor: null,
HoverBackgroundColor: null,
HoverBorderColor: null,
ClickEventListener: OnClick
};
oCustomContextMenu = new CustomContextMenu(Arguments);
oCustomContextMenu.AddItem('Images/ei0019-48.gif', 'Add', false, 'Add');
oCustomContextMenu.AddItem('Images/save.png', 'Save', true, 'Save');
oCustomContextMenu.AddSeparatorItem();
oCustomContextMenu.AddItem('Images/ei0020-48.gif', 'Update', false, 'Update');
oCustomContextMenu.AddSeparatorItem();
oCustomContextMenu.AddItem(null, 'Cancel', false, 'Cancel');
}
</script>
First, get the reference of the Base
object and create an Arguments
Object Literal with the necessary properties. After that, instantiate a context menu object using the new
keyword and add the context menu items. Don’t forget the Click
event wire up in the Arguments
Object Literal:
ClickEventListener: OnClick
Now, create a Click
event handler as a local anonymous method:
var OnClick = function(Sender, EventArgs)
{
…
oCustomContextMenu.Hide();
}
var OnClick = function(Sender, EventArgs)
{
switch(EventArgs.CommandName)
{
case 'Add':
alert('Text: ' + EventArgs.Text);
alert('IsDisabled: ' + EventArgs.IsDisabled);
alert('ImageUrl: ' + EventArgs.ImageUrl);
break;
case 'Save':
alert('Text: ' + EventArgs.Text);
alert('IsDisabled: ' + EventArgs.IsDisabled);
alert('ImageUrl: ' + EventArgs.ImageUrl);
break;
case 'Update':
alert('Text: ' + EventArgs.Text);
alert('IsDisabled: ' + EventArgs.IsDisabled);
alert('ImageUrl: ' + EventArgs.ImageUrl);
break;
case 'Cancel':
alert('Text: ' + EventArgs.Text);
alert('IsDisabled: ' + EventArgs.IsDisabled);
alert('ImageUrl: ' + EventArgs.ImageUrl);
break;
}
oCustomContextMenu.Hide();
}
This method will get called when you click on any item of the context menu. Don’t forget to invoke the Hide
method at last in the event handler.
Now, attach the oncontextmenu
event on the div
that has been created earlier:
< … oncontextmenu="javascript:return oCustomContextMenu.Display(event);" … >
Invoke the Dispose
method in the window.onunload
event in order to destroy the context menu object:
window.onunload = function(){ oCustomContextMenu.Dispose(); }
Conclusion
So this is my approach. I was working for a long time to create C# like event handlers for JavaScript classes and finally, I’ve done it. Please let me know of any bugs and suggestions to improve this context menu control.
Browser Compatibility
I have tested this control on various browsers and it works fine except on Opera as Opera doesn’t support the oncontextmenu
event. If any one has information regarding simulating the oncontextmenu
event on Opera, kindly let me know. The following are the supported browsers:
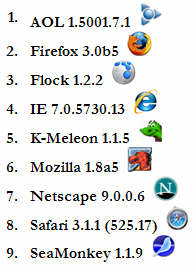