Introduction
This is my first article ever, so go easy on me. This is an article about a method of saving application settings to the permanent storage I use, and it is proven to me to be useful and easy to maintain and to add new settings members.
Background
The background to this was that I had problems changing app.config and web.config settings files to save some simple settings of my Win/web/mobile applications. So, I wrote my own class to save the application settings, and it stuck to me (even though I learned to change the app.config).
Using the code
Using the code should be easy. All classes needed are contained in a file clsSettings.cs which you include in your project. The next thing you should do is change the class namespace to match your default namespace, as it makes it easier to use.
About the clsSettings class
The class has two methods, Save
and Load
, which save and load the clsSettings
object to/from a desired path. It also has private members and public properties which are added/changed to fit your needs.
The clsSetting
class has two members which are classes of their own. One is called clsApplication
and the other Paths
. These two, with their members, will be saved when the Save
method is called.
[Serializable]
public class clsSettings
{
#region private Fields
private clsApplication appRelated = new clsApplication();
private Paths paths = new Paths();
#endregion
#region Public properties
public Paths Paths
{
get
{
return paths;
}
set
{
paths = value;
}
}
public clsApplication AppRelated
{
get
{
return appRelated;
}
set
{
appRelated = value;
}
}
#endregion
#region Methods: Save, Load
public void Save(string fileName)
{
XmlSerializer mySerializer = new XmlSerializer(typeof(clsSettings));
StreamWriter myWriter = new StreamWriter(fileName);
mySerializer.Serialize(myWriter, this);
myWriter.Close();
}
public clsSettings Load(string fileName)
{
XmlSerializer mySerializer = new XmlSerializer(typeof(clsSettings));
FileStream myFileStream = new FileStream(fileName, FileMode.Open);
clsSettings pos = (clsSettings)mySerializer.Deserialize(myFileStream);
myFileStream.Close();
return pos;
}
#endregion
}
You can add members to any of these classes and they will be saved/loaded as well.
The clsApplication
member class in the demo looks like this:
public class clsApplication
{
#region private fields
private string version = "";
private string lsStrIDLeft = "";
private string lsStrIDRight = "";
#endregion
#region public properties
public string LsStrIDLeft
{
get { return lsStrIDLeft; }
set { lsStrIDLeft = value; }
}
public string LsStrIDRight
{
get { return lsStrIDRight; }
set { lsStrIDRight = value; }
}
public string Version
{
get { return version; }
set { version = value; }
}
#endregion
}
The way to add new members is that you add a private member like:
private string version = "";
and then click the right button on the version and:
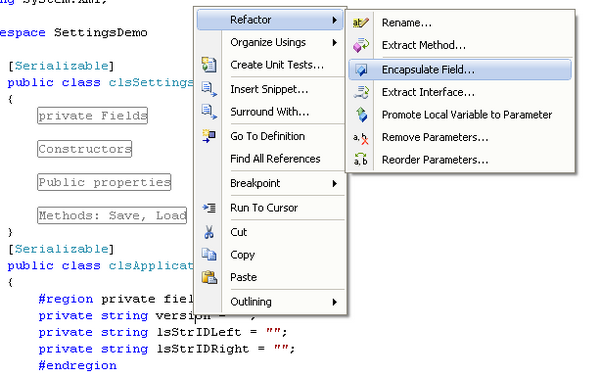
This will generate the public property which will get/set the value of the private member.

The way I use this clsSetting
class is that I add a public static member of the Program
class, so I can access the setting from any other class or form in my application. Also, I add a public static method to the Program
class which returns the path where the settings will be saved/loaded, like this:
static class Program
{
public static clsSettings sets = new clsSettings();
public static string GetSettingPath()
{
return Application.StartupPath + "settings.xml";
}
.
.
.
and access it from anywhere, for example, like this:
this.Text += " " + Program.sets.AppRelated.Version;
To load the settings, call the Load method of the clsSettings
instance; for example, like this:
Program.sets = Program.sets.Load(Program.GetSettingPath());
To save the settings, call the Save method of the clsSettings
instance, like this:
Program.sets.Save(Program.GetSettingPath());
Points of interest
You can use this class to save application settings, like database paths, database addresses, various IP addresses, last selected values of list boxes, grids, combos, last open forms, web service addresses, and such..