Introduction
JQuery is an excellent JavaScript library to build modern user interactive websites. It's very easy to use jQuery in any web application. jQuery has a strong set of JavaScript UI controls, like date picker, tab panel etc.
I've written a small utility class to use the jQuery DatePicker
in ASP.NET. It's very simple to use this class. You just need to pass the Page
object and TextBox
of your page.
Screenshot
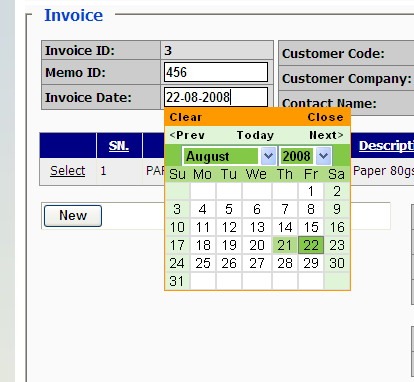
Using the code
Take a look at the the following utility class:
using System;
using System.Collections.Generic;
using System.Text;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace Util
{
public class JQueryUtils
{
public static void RegisterTextBoxForDatePicker(Page page,
params TextBox[] textBoxes)
{
RegisterTextBoxForDatePicker(page, "dd-mm-yy", textBoxes);
}
public static void RegisterTextBoxForDatePicker(Page page,
string format, params TextBox[] textBoxes)
{
bool allTextBoxNull = true;
foreach (TextBox textBox in textBoxes)
{
if (textBox != null) allTextBoxNull = false;
}
if (allTextBoxNull) return;
page.ClientScript.RegisterClientScriptInclude(page.GetType(),
"jquery", "JQuery/jquery.js");
page.ClientScript.RegisterClientScriptInclude(page.GetType(),
"jquery.ui.all", "JQuery/ui/jquery.ui.all.js");
page.ClientScript.RegisterClientScriptBlock(page.GetType(),
"datepickerCss",
"<link rel=\"stylesheet\" href=\"JQuery/themes/" +
"flora/flora.datepicker.css\" />");
StringBuilder sb = new StringBuilder();
sb.Append("$(document).ready(function() {");
foreach (TextBox textBox in textBoxes)
{
if (textBox != null)
{
sb.Append("$('#" + textBox.ClientID + "').datepicker(
{dateFormat: \"" + format + "\"});");
}
}
sb.Append("});");
page.ClientScript.RegisterClientScriptBlock(page.GetType(),
"jQueryScript", sb.ToString(), true);
}
}
}
The usage is very simple. If you have a TextBox
named MyDateTextBox
in your .aspx page, then you have to write the following line in the Page_Load
event to attach the TextBox
with jQuery:
protected void Page_Load(object sender, EventArgs e)
{
Util.JQueryUtils.RegisterTextBoxForDatePicker(Page, MyDateTextBox);
}
The second parameter takes variable arguments. So you can pass any number of TextBox
es to the function. There is also an overloaded function to take your desired date format.
You must download the jQuery library from the jQuery UI site http://ui.jquery.com/download.
You can just download the files for DatePicker
. But remember to rename the .js files in my JQueryUtils
class. You can see that I've written the jQuery core library JavaScript file name, .ui.all.js file, and the .css file for DatePicker
.
I've attached a sample project as well. Cheers!
My other articles