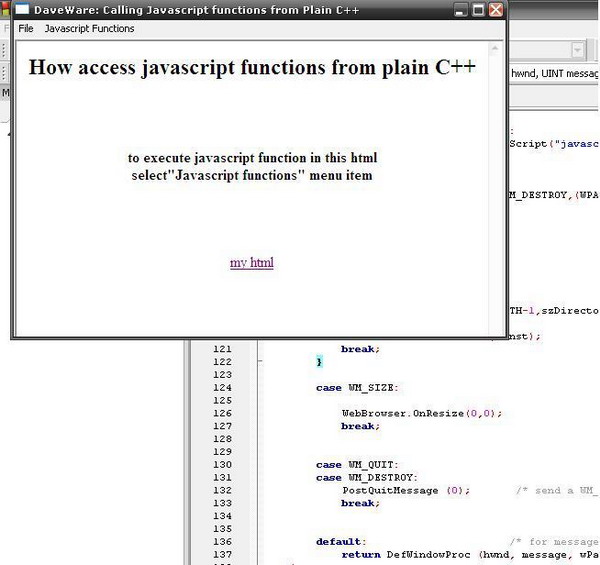
Introduction
How can we execute JavaScript functions from C++, a question I asked a long time ago. But, recently, when I needed an interface between a C++ code and a JavaScript function within an HTML page, I decided to code in search of an answer to that question.
Using the code
For this solution, we have a file called "daveWare.html" located in the same directory/path as the *.exe of this program. The JavaScript function in this html page is:
.........
<script type="text/javascript">
function message(messagestring)
{
alert(messagestring);
}
</script>
........
The solution, just one of many solutions, is to interface the HTML page/document using a COM/ATL interface, the IWebBrowser2
interface within the dWebCtrl
class.
With this interface and its method "Navigate
", we can navigate URLs and execute JavaScript (using javascript:) codes in the URLs, as follows:
void dWebCtrl::ExecuteScript(char* Script)
{
BSTR bstrScript;
BSTR bufferScript;
static DWORD size=0;
char nameInput[256]={'\0'};
memset(nameInput,'\0',sizeof(nameInput)-1);
wsprintf(nameInput,"javascript:%s",Script);
size = MultiByteToWideChar(CP_ACP, 0, nameInput, -1, 0, 0);
if (!(bufferScript =
(wchar_t *)GlobalAlloc(GMEM_FIXED, sizeof(wchar_t)*size)))
return;
MultiByteToWideChar(CP_ACP, 0, nameInput, -1, bufferScript, size);
bstrScript = SysAllocString(bufferScript);
SysFreeString(bufferScript);
pIwb->Navigate(bstrScript, &vEmpty, &vEmpty, &vEmpty, &vEmpty);
SysFreeString(bstrScript);
return;
}
That's it. In our main process, we can access any JavaScript function and pass any parameter to it from our C++ code:
LRESULT CALLBACK WindowProcedure (HWND hwnd, UINT message,
WPARAM wParam, LPARAM lParam)
{
switch (message)
{
case WM_COMMAND:
switch LOWORD(wParam)
{
case IDM_COMMAND_ALERT:
WebBrowser.ExecuteScript("javascript:message('David is the best');");
break;
case IDM_FILE_EXIT:
SendMessage(hwnd,WM_DESTROY,(WPARAM)0,(LPARAM)0);
break;
}
break;
case WM_CREATE:
{
GetCurrentDirectory(MAX_PATH-1,szDirectorioTrabajo);
WebBrowser.Iniciar(hwnd,hInst);
break;
}
case WM_SIZE:
WebBrowser.OnResize(0,0);
break;
case WM_QUIT:
case WM_DESTROY:
PostQuitMessage (0);
break;
default:
return DefWindowProc (hwnd, message, wParam, lParam);
}
return 0;
}
Now, you can interface your C++ code with any JavaScript function located in any HTML page (you must know how the JavaScript function works, of course). My next step, access JavaScript "var
" defined variables from C++. Any advice?
Best regards to all, and good luck with this stuff. My e-mail address. My URL.