Introduction
This property grid control has been developed in Visual Studio C# 2008. This user control is similar to the standard Microsoft .NET 2.0 PropertyGrid
control with several additional features:
- Gets/sets any property item at runtime (e.g. change property’s help text)
- New property items (
Date
and Time
, Date
, Time
, File
, Directory
, ProgressBar
, ...) - Multi-language support
- Image Preview
- Date calendar
- Numeric interval automatic validation
- Customizable Boolean type (yes/no, true/false, ..)
- Customizable engineering unit for numeric values
- Use .NET standard dialog box, text title, new buttons (show text, apply), ...
This control is fully customizable at runtime. Property items can be added and changed at any time.
Background
The main problem of the Microsoft .NET 2.0 standard Property Grid Control is that it is not easy to control at run-time. Using this control is possible, for example, to change the language at runtime or to change a property that normally is read-only using the standard control.
Using the Code
To run the example project:
- Extract all files from ZIP into a folder
- Run Visual Studio C #2008 and select the command "File" > "Open project"
- Select the file "\Test\Test_mbrPropertyGrid\Test_mbrPropertyGrid.sln"
- Select the command "Build" > "Rebuild solution"
- Press the [F5] keyboard button to run the program
To build your new test project:
- Run Visual Studio C #2008 and create a new project (standard Windows form application)
- Add a reference to the .NET DLL "mbrPropertyGrid.dll"
- Right-click in toolbox windows and select the command "Choose Items..."
- Select the browse button and select the .NET DLL "mbrPropertyGrid.dll"
- Drag and drop the new
PropertyGrid
icon from the toolbox to your project form to add the control to your project - Set the control properties to customize it
- Write code to add/manage property items
To logically group the various item objects, at first, add a category item:
mbrPropertyGrid.PropertyItemCategory catItem;
catItem = new mbrPropertyGrid.PropertyItemCategory("Main Category");
PropertyGrid1.CategoryAdd("CatMain", catItem);
To add property items to a category use the .ItemAdd
method like the following example :
mbrPropertyGrid.PropertyItemInt32 intItem;
intItem = new mbrPropertyGrid.PropertyItemInt32("Line02 - Age (Int32)", 0);
intItem.SetValidationRange(0, 120, 1);
intItem.SetHelpCaptionText("Age", "Tell me your age (valid range : 0..120)");
PropertyGrid1.ItemAdd("CatMain", "MyAge", intItem);
mbrPropertyGrid.PropertyItemString strItem;
strItem = new mbrPropertyGrid.PropertyItemString
("Line01 - Name (String)", "Jak", "Jak Smith");
strItem.HelpCaption = "Name";
strItem.HelpText = "Tell me your name...";
PropertyGrid1.ItemAdd("CatMain", "YourName", strItem);
To disable a property item, set the item property .Enabled
to false
like in the following example:
strItem = new mbrPropertyGrid.PropertyItemString
("Line03 - Job (String)", "A software developer");
strItem.HelpText = "Tell me about your job";
strItem.Enabled = false;
PropertyGrid1.ItemAdd("CatMain", "K03", strItem);
When you have completed the addition of item objects to force the complete control repaint, run the .RefreshControl(true)
method like in the following code:
PropertyGrid1.RefreshControl(true);
A quick screen shot control preview. Please note that the standard dialog box will be shown in the same language of the operating system.
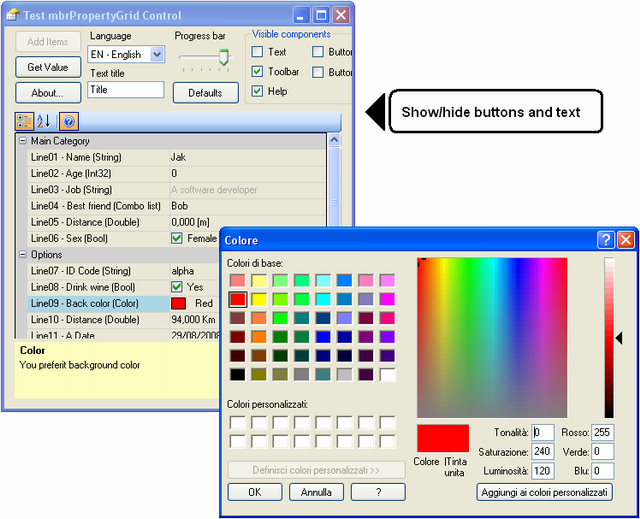












Points of Interest
To change the language at runtime, simply change the following properties of the property items: Text
, HelpCaption
, HelpText
(and Description
for a Directory Item).
History
- Version 1.0.0.0 - 28/08/2008: This is the first version. Some points have to be accommodated. For example, there is a known bug on a video refresh of the
.Text
property. I suggest, at the moment, to disable the Text
property view by setting .TextVisible = false;
About Me
My name is Massimiliano Brugnerotto and I'm an Italian C# software developer. I believe that open source software is a useful resource and with this article, I will give my contribution to this site that on many occasions gave me interesting development solutions.