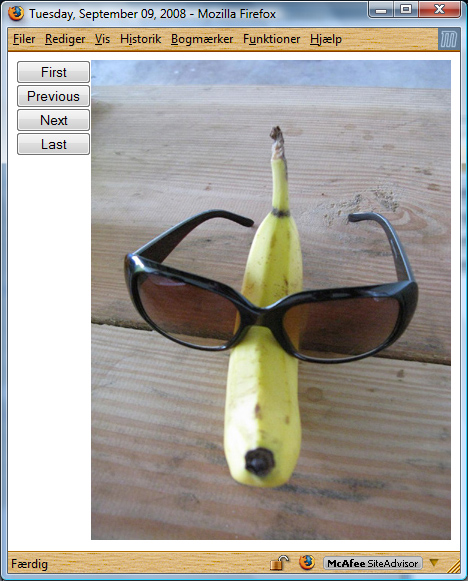
Introduction
I have written an image browser page with ASP.NET, using ASP.NET AJAX to retrieve information about the images on the server. Images are displayed using a single ASPX page and a folder with JPG files. The code-behind is written in C#. No database is involved.
Background
My goal was to show my family some photos on a web page, and hopefully spending less than a few hours accomplishing that. I wanted to do so without using a database, without titles, without descriptions, without tags, etc. However, I included the shooting date of the photo as part of the file name, since it provided a way to retain some information without using a database.
Using the code
Code-behind (C#)
I first created an ASPX web form, and in the code-behind, wrote a static web method to return information about the images on the server:
[WebMethod]
public static Image[] GetImages()
{
string imageFolderVirtualPath = "~/Photos/";
string imageFolderPath = HttpContext.Current.Server.MapPath(imageFolderVirtualPath);
List<image> images = new List<image>();
DirectoryInfo diImages = new DirectoryInfo(imageFolderPath);
foreach (FileInfo fiImage in diImages.GetFiles("*.jpg"))
{
string fileName = fiImage.Name;
string date = string.Empty;
int year = 0;
int month = 0;
int day = 0;
if (fileName.Length > 9 && Int32.TryParse(fileName.Substring(0, 4), out year)
&& Int32.TryParse(fileName.Substring(5, 2), out month)
&& Int32.TryParse(fileName.Substring(8, 2), out day))
{
date = new DateTime(year, month, day).ToLongDateString();
}
images.Add(new Image
{
Date = date,
VirtualPath = CombineVirtualPaths(imageFolderVirtualPath, fileName)
});
}
return images.ToArray();
}
A helper method combines the virtual paths:
private static string CombineVirtualPaths(string virtualPath1, string virtualPath2)
{
return string.Format("{0}/{1}", virtualPath1.Trim('~', '/'), virtualPath2.Trim('/'));
}
A helper class provides information about an image:
public class Image
{
public string VirtualPath { get; set; }
public string Date { get; set; }
}
HTML/ASPX markup
In the *.aspx file, I have the following markup:
<body onload="GetImages();">
<form id="form1" runat="server">
<asp:ScriptManager id="sm1" runat="server"
EnablePageMethods="true" EnableScriptGlobalization="true">
</asp:ScriptManager>
<div>
<div id="divButtons" style="float: left;">
</div>
<div id="divImage" style="float: left;">
</div>
</div>
</form>
</body>
JavaScript
On page load, a JavaScript method is fired that retrieves info from the page method:
var images;
var currentImageIndex = 0;
function GetImages() {
PageMethods.GetImages(GetImagesCompleted);
}
function GetImagesCompleted(result) {
images = result;
ShowImage();
}
function ShowImage() {
var currentImage = images[currentImageIndex];
var date = currentImage.Date;
var imgImage = "<img id='imgImage' alt='" + date +
"' title='" + date + "' src='" +
currentImage.VirtualPath "' />";
var dp = document.getElementById("divImage");
dp.innerHTML = imgImage;
document.title = date;
ShowButtons();
}
A separate JavaScript method displays the appropriate navigation buttons:
function ShowButtons() {
var first = "First";
var previous = "Previous";
var next = "Next";
var last = "Last";
if (Sys.CultureInfo.CurrentCulture.name.toLowerCase().startsWith("da")) {
first = "Første";
previous = "Forrige";
next = "Næste";
last = "Sidste";
}
var button1 = "<div><input type='button' style='width: 75px;'";
var btnFirst = button1 + " value='" + first +
"' onclick='ShowFirstImage();'";
var btnPrevious = button1 + " value='" + previous +
"' onclick='ShowPreviousImage();'";
var btnNext = button1 + " value='" + next +
"' onclick='ShowNextImage();'";
var btnLast = button1 + " value='" + last +
"' onclick='ShowLastImage();'";
if (currentImageIndex == 0) {
btnFirst += " disabled='disabled'";
btnPrevious += " disabled='disabled'";
}
if (currentImageIndex == images.length - 1) {
btnNext += " disabled='disabled'";
btnLast += " disabled='disabled'";
}
var button2 = " /></div>";
btnFirst += button2;
btnPrevious += button2;
btnNext += button2;
btnLast += button2;
var db1 = document.getElementById("divButtons");
db1.innerHTML = btnFirst + btnPrevious + btnNext + btnLast;
}
function ShowFirstImage() {
currentImageIndex = 0;
ShowImage();
}
function ShowPreviousImage() {
if (currentImageIndex > 0) {
currentImageIndex -= 1;
ShowImage();
}
}
function ShowNextImage() {
if (currentImageIndex < images.length - 1) {
currentImageIndex += 1;
ShowImage();
}
}
function ShowLastImage() {
currentImageIndex = images.length - 1;
ShowImage();
}
Points of interest
- A useful application built in a single web form and a folder with images.
- The
EnableScriptGlobalization
feature on the ScriptManager
and the ability to extract language information in JavaScript using Sys.CultureInfo.CurrentCulture.name
.