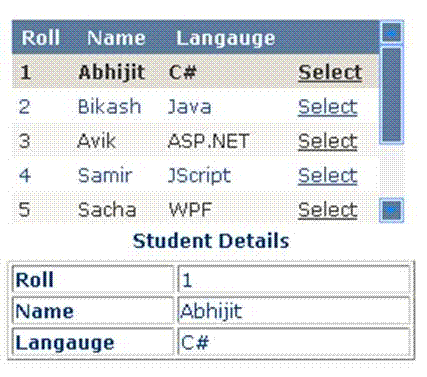
Introduction
For most kind of web development, we mostly need to show data inside a GridView
. Suppose we need to show 500 records inside
a GridView
and when the user selects a record, the details are displayed in the bottom or any where else in the page. Now for preventing postback
for such operations, generally we use the AJAX UpdatePanel
and put the GridView
inside the UpdatePanel
. This will resolve the problem
of postback, but then what happens? When you want to check record 100, you just scroll down the records and select the record for checking the details,
and now you get the records inside your details area, but look at the GridView
scroll position. Ohhhh...no, it should not be happening: it is back at the top
of the records. We have to now solve this problem at the time of partial postback of the page.
Problem Statement
Here I am going to describe the actual problem in detail:
Problem State | Description |

| I have a GridView with a scroll bar where I have 8 records. I can see 5 records at a time. For getting the other records, I have to scroll down.
I just select the first records and get the details in the details area. |

| No I want to see some other records. Let's say the 8th record. I just scroll down the scroll bar but I don't select
a record. Now check the scroll bar position and the student detail. |

| Now I select the 8th record. You will see in the details section that the detail of that records is showing, but check the scroll bar position. |
This is quite a frustrating scenario for the user. We have a good solution for that by handling the partial post back of the page.
The Solution
When we are using an UpdatePanel
, we need more control on the UpdatePanel
to solve this problem. For that, we need to use
the Sys.WebForms.PageRequestManager
class. This is used to manage the partial-page update by using client-side script. We do not need to create
an object of the PageRequestManager
class directly. For this solution, I have used two events. beginRequest
is raised before processing
of the asynchronous postback starts. Here I have just retrieved the Div
position of the scroll and stored it in a variable. endRequest
is raised
after an asynchronous postback is finished and control has been returned to the browser. Here I have just assigned the retrieved scroll position to the div
again.
For more info, click here.
Using the Code
I have written the following JavaScript code for handling the partial postback, which handles the Div
scroll position during the postback
of the GridView
inside the UpdatePanel
:
<script language="javascript" type="text/javascript">
var scrollTop;
Sys.WebForms.PageRequestManager.getInstance().add_beginRequest(BeginRequestHandler);
Sys.WebForms.PageRequestManager.getInstance().add_endRequest(EndRequestHandler);
Function BeginRequestHandler(sender, args)
{
var m = document.getElementById('divGrid');
scrollTop=m.scrollTop;
}
function EndRequestHandler(sender, args)
{
var m = document.getElementById('divGrid');
m.scrollTop = scrollTop;
}
</script>
Following is the code for the GridView
. The Gridview
should be placed inside an UpdatePanel
and a Div
control.
The Div
allow us to scroll the GridView
.
<asp:UpdatePanel ID="UpdatePanel1" runat="server">
<ContentTemplate>
<div id="divGrid" style="overflow: auto; height: 130px">
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False" CellPadding="4"
DataSourceID="SqlDataSource1" ForeColor="#333333" GridLines="None" Width="235px"
OnSelectedIndexChanged="GridView1_SelectedIndexChanged">
<FooterStyle BackColor="#5D7B9D" Font-Bold="True" ForeColor="White" />
<RowStyle BackColor="#F7F6F3" ForeColor="#333333" />
<HeaderStyle CssClass="HeaderFreez" />
<Columns>
<asp:BoundField DataField="Roll" HeaderText="Roll" SortExpression="Roll" />
<asp:BoundField DataField="Name" HeaderText="Name" SortExpression="Name" />
<asp:BoundField DataField="Langauge" HeaderText="Language" SortExpression="Langauge" />
<asp:CommandField ShowSelectButton="True" />
</Columns>
<PagerStyle BackColor="#284775" ForeColor="White" HorizontalAlign="Center" />
<SelectedRowStyle BackColor="#E2DED6" Font-Bold="True" ForeColor="#333333" />
<HeaderStyle BackColor="#5D7B9D" Font-Bold="True" ForeColor="White" />
<EditRowStyle BackColor="#999999" />
<AlternatingRowStyle BackColor="White" ForeColor="#284775" />
</asp:GridView>
</div>
<asp:SqlDataSource ID="SqlDataSource1" runat="server"
ConnectionString="<%$ ConnectionStrings:DotNetRnDDBConnectionString %>"
SelectCommand="SELECT * FROM [StudDetails]"></asp:SqlDataSource>
</ContentTemplate>
</asp:UpdatePanel>
Here is the CSS code which is used to freeze the Header
of the GridView
. Just add this class as a HeaderStyle
class
of the Gridview
. This will freeze the grid's header. We can use it in other cases where we need to freeze the header.
.HeaderFreez
{
position:relative ;
top:expression(this.offsetParent.scrollTop);
z-index: 10;
}
Reference
History