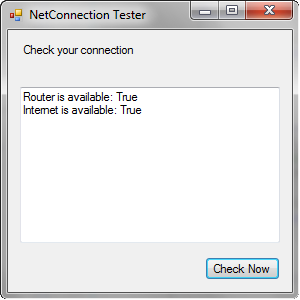
Introduction
This article will show how to use my netUtility (short for NetworkUtility) library to check Internet connections, listen for Internet connections and other methods as finding router,
check if a webpage are available and a Ping method.
Requires .NET 3.0 or above.
Background
I created this as a result of a project I have, I needed a way to check Internet connections and I seached the web and found many different ways to solve the problem.
There were so many that I decided to create a class library file that solves the problem for me and I can be re-used in other projects.
Using the code
Before you get started you need to place the netUtility.dll (in the source code above) in your project folder. In your solution you need to add the netUtility.dll as a reference (browse). Then you import it by type the following:
using netUtility;
Thereafter you are able to create an instance of NetworkUtility class:
NetworkUtility netUtil = new NetworkUtility();
The NetworkUtility has the following methods:
netUtil.AddressExistOrAvailable(string address);
netUtil.CheckInternetConnection();
netUtil.GetAddressHeaders(string address);
netUtil.Ping(string address);
netUtil.CheckAvailability(bool checkInternet);
The following are the Properties of NetworkUtility
netUtil.ErrorMessage;
netUtil.RouterInfo;
The following are the Events of the NetworkUtility
netUtil.RouterAvailableEvent += new RouterAvailableHandler(netUtil_RouterAvailableEvent);
The following will show how to check for a router and print its information, check Internet connection and at last Ping google.
NetworkUtility netUtil = new NetworkUtility(); <br />
Console.WriteLine("Router connection: " + netUtil.CheckRouterConnection());
if(netUtil.CheckRouterConnection)
{
string[] routerInfo = netUtil.RouterInfo;
for(int i = 0; i < routerInfo.Length; i++)
{
Console.WriteLine(routerInfo[i]);
}
Console.WriteLine("\nInternet connection: " + netUtil.CheckInternetConnection());
if(netUtil.CheckInternetConnection)
{
Console.WriteLine("n\Ping webaddress: " + netUtil.Ping("http://www.google.com"));
try {
string[] pingInfo = netUtil.PingInfo;
for(int i = 0; i < pingInfo.Length; i++)
{
Console.WriteLine(pingInfo[i]);
}
} catch (Exception) {
Console.WriteLine(netUtil.ErrorMessage);
}
}
}
The following are the code in the testApp(console application) that prints out all data:
using System;
using System.Collections.Generic;
using System.Text;
using netUtility;
namespace testApp
{
class Program
{
public static void Main(string[] args)
{
NetworkUtility netUtil = new NetworkUtility();
Console.WriteLine("Router connection: " +
netUtil.CheckRouterConnection());
string[] routerInfo = netUtil.RouterInfo;
for(int i = 0; i < routerInfo.Length; i++)
{
Console.WriteLine(routerInfo[i]);
}
Console.WriteLine("Internet connection: " +
netUtil.CheckInternetConnection());
Console.WriteLine();
Console.WriteLine("Ping IP: " +
netUtil.Ping("209.85.137.99"));
try {
string[] pingIpInfo = netUtil.PingInfo;
for(int i = 0; i < pingIpInfo.Length; i++)
{
Console.WriteLine(pingIpInfo[i]);
}
} catch (Exception) {
Console.WriteLine(netUtil.ErrorMessage);
}
Console.WriteLine();
Console.WriteLine("Ping webaddress: " +
netUtil.Ping("http://www.google.com"));
try {
string[] pingInfo = netUtil.PingInfo;
for(int i = 0; i < pingInfo.Length; i++)
{
Console.WriteLine(pingInfo[i]);
}
} catch (Exception) {
Console.WriteLine(netUtil.ErrorMessage);
}
Console.WriteLine();
Console.WriteLine("The address exist: " +
netUtil.AddressExistOrAvailable("http://www.google.com"));
if(netUtil.ErrorMessage != null)
{
Console.WriteLine(netUtil.ErrorMessage);
}
Console.WriteLine();
try {
string[] getHeaders = netUtil.GetAddressHeaders("http://www.google.com");
for(int i = 0; i < getHeaders.Length; i++)
{
Console.WriteLine(getHeaders[i]);
}
} catch (Exception) {
Console.WriteLine(netUtil.ErrorMessage);
}
Console.Write("Press any key to continue . . . ");
Console.ReadKey(true);
}
}
}

To setup a listener, you will need the following code:
private NetworkUtility net_utility = new NetworkUtility();
net_utility.InternetAvailableEvent += new NetworkUtility.InternetAvailableHandler(InternetAvailable);
private void RouterAvailable(object sender, RouterAvailableEventArgs e)
{
if (sender is NetworkUtility)
{
NetworkUtility n_utility = (NetworkUtility)sender;
Print("Router" ,e.IsAvailable);
}
}
private void InternetAvailable(object sender, InternetAvailableEventArgs e)
{
if (sender is NetworkUtility)
{
NetworkUtility n_utility = (NetworkUtility)sender;
Print("Internet", e.IsAvailable);
}
}
net_utility.CheckAvailability(true);
Below you will have the whole code for the NetConnection Tester.
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Windows.Forms;
using netUtility;
namespace NetConnection_Tester
{
public partial class MainForm : Form
{
private NetworkUtility net_utility = new NetworkUtility();
public MainForm()
{
InitializeComponent();
}
void Button1Click(object sender, EventArgs e)
{
net_utility.CheckAvailability(true);
}
private void RouterAvailable(object sender, RouterAvailableEventArgs e)
{
if (sender is NetworkUtility)
{
NetworkUtility n_utility = (NetworkUtility)sender;
Print("Router" ,e.IsAvailable);
}
}
private void InternetAvailable(object sender, InternetAvailableEventArgs e)
{
if (sender is NetworkUtility)
{
NetworkUtility n_utility = (NetworkUtility)sender;
Print("Internet", e.IsAvailable);
}
}
private void Print(string data, bool status)
{
textBox1.AppendText(string.Format("{0} is available: {1}\n", data, status));
}
void MainFormLoad(object sender, EventArgs e)
{
net_utility.RouterAvailableEvent +=
new NetworkUtility.RouterAvailableHandler(RouterAvailable);
net_utility.InternetAvailableEvent +=
new NetworkUtility.InternetAvailableHandler(InternetAvailable);
}
}
}
Points of Interest
This library file has been useful to me, I saved basicly 20-30 lines of code by building this. I needed just a few lines to check everything I needed for my project.
History
Article updated (2012-02-21)
Article updated (2012-02-20)
Latest version 1.0.0.0