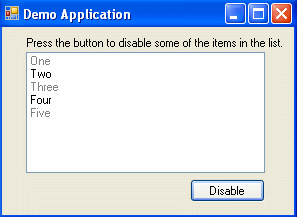
Introduction
This is a listbox User Control with an option for disabled items. The disabled item can be marked as disabled with a prefix that you choose, or by choosing a boolean column in the source database for the list DataSource
.
Background
I had some projects that I needed a listbox to display disabled item. The ListBox
that comes with VS does not expose this attribute for the listbox items. I ended up writing my own User Control with the ability to mark some items as disabled. This is great if you have an application that uses a database and has many users that update the data, and you want to mark an object as disabled if you can't use it any more, or if say, some one else is working on the same object at that time.
Using the code
The base for this solution is using the DrawMode.OwnerDrawFixed
attribute in the ListBox
control. We need to start by creating a UserControl project and inherit from ListBox
. Then, just add the following line inside the constructor of the control:
this.DrawMode = DrawMode.OwnerDrawFixed;
This means that we control how the listbox is drawn.
Next, we need to override the method OnDrawItem(DrawItemEventArgs e)
. This method does the drawing of the items inside the listbox control. Here, we choose if we want to draw the item as disabled or not.
This is the code for a disabled item base using a prefix for disabled items:
string item = this.Items[e.Index].ToString();
if (item.StartsWith(prefix))
{
item = item.Remove(0, prefix.Length);
myBrush = Brushes.Gray;
}
if (!item.StartsWith(prefix))
{
e.DrawBackground();
}
e.Graphics.DrawString(item, e.Font, myBrush, e.Bounds, StringFormat.GenericDefault);
Next, we want to enable selecting an item.
if ((e.State & DrawItemState.Selected) ==
DrawItemState.Selected && item.StartsWith(prefix))
{
this.SelectedIndex = -1;
this.Invalidate();
}
else if ((e.State & DrawItemState.Selected) == DrawItemState.Selected)
{
myBrush = Brushes.White;
e.Graphics.DrawString(item, e.Font, myBrush, e.Bounds,
StringFormat.GenericDefault);
e.DrawFocusRectangle();
}
else
{
if (!item.StartsWith(prefix))
e.DrawFocusRectangle();
}
Now, we must draw the item back into the listbox control:
base.OnDrawItem(e);
In the attached source code, you will find the code for connecting the disabled items to a column in a database.
That's it. Enjoy!
Points of interest
It is always fun to play with the built-in controls. Remember, you can do any thing you want (almost) in VS.