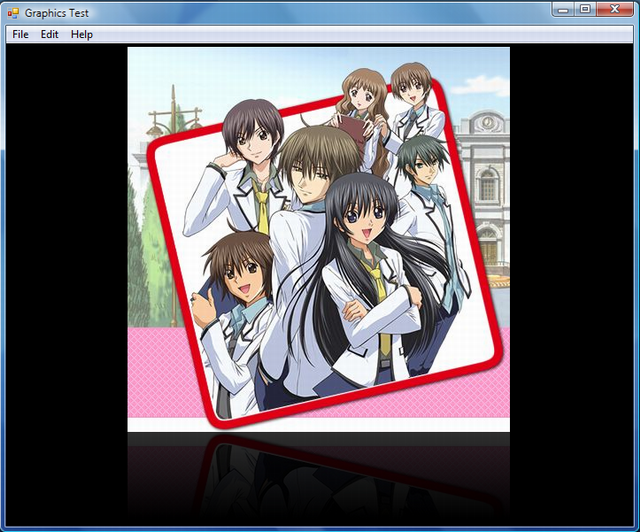
Introduction
This source code will teach you how to create a simple GDI+ Glass Reflection effect in C#.NET.
The Codes
Make sure to include these namespaces:
System.Drawing;
System.Drawing.Drawing2D;
System.Drawing.Imaging;
Here's the DrawImage
code. It gets the image from the pictureBox1
, then creates a reflection.
private static Image DrawReflection(Image img,Color toBG)
{
int height = img.Height + 100;
Bitmap bmp = new Bitmap(img.Width, height, PixelFormat.Format64bppPArgb);
Brush brsh = new LinearGradientBrush(new Rectangle(0, 0, img.Width + 10,
height), Color.Transparent, toBG, LinearGradientMode.Vertical);
bmp.SetResolution(img.HorizontalResolution, img.VerticalResolution);
using(Graphics grfx = Graphics.FromImage(bmp))
{
Bitmap bm = (Bitmap)img;
grfx.DrawImage(bm, 0, 0, img.Width, img.Height);
Bitmap bm1 = (Bitmap)img;
bm1.RotateFlip(RotateFlipType.Rotate180FlipX);
grfx.DrawImage(bm1, 0, img.Height);
Rectangle rt = new Rectangle(0, img.Height, img.Width, 100);
grfx.FillRectangle(brsh, rt);
}
return bmp;
}
Now after creating this function, use it to change the image of the picturebox
through a click event or other events.
private void controlname_click(Object sender, EventArgs e)
{
pictureBox1.Image = DrawReflection(pictureBox1.Image, Color.Black);
}
This code changes the current pictureBox1
's image to the image generated by the DrawReflection
.
How Does It Work?
This is the result if the image is not painted with gradient.

Explanation for the gradient:

The flipped image below the original image has a fading effect because of the gradient we painted on it. The starting color is Transparent
. We can see the image below it. And as it goes down, it changes to color black, which is the color of the form, so the lower portion of the image blends with the background color, creating a fading effect.
What Problems Does This Solution Solve?
I made this solution so that beginner coders could better understand the Image Reflection in C#.NET. It is made in the Main Form's class.
How Does This Help Someone?
This helps better understanding of the usage of the System.Drawing
classes and functions.
History
- 6/03/09 - Initial release (this source code)
- 9/01/24 - Release to public domain