Introduction
In this article, I will explain how to manipulate text in the Clipboard. Here is how the main form in the sample application looks like:
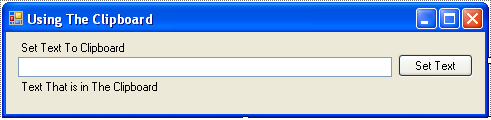
Adding Controls
First of all, create a new project (Windows Forms Application) and add two Label
s, then add a TextBox
(textClip
), and then another Label
(labelClip
) that will show what is in the Clipboard. Now, add a Timer
(timerRefresh
) that will refresh what is in the Clipboard to you automatically, and finally, add a Button
(buttonSet
).
The Code
First of all, define the interval of the Timer
. In this article, I will use 5000ms (5 sec.) as interval. Now, let's get into the code!
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
PasteData();
}
private void SetToClipboard(object text)
{
System.Windows.Forms.Clipboard.SetDataObject(text);
}
private void PasteData()
{
IDataObject ClipData = System.Windows.Forms.Clipboard.GetDataObject();
timerRefresh.Start();
if (ClipData.GetDataPresent(DataFormats.Text))
{
string s = System.Windows.Forms.Clipboard.GetData(DataFormats.Text).ToString();
labelClip.Text = s;
}
}
private void buttonSet_Click(object sender, EventArgs e)
{
SetToClipboard(textClip.Text);
}
private void timerRefresh_Tick(object sender, EventArgs e)
{
PasteData();
}
}
Explanation
PasteData()
: In the PasteData
, we have a IDataObject
called ClipData
that will store the Clipboard data object, then the Timer
starts. The if clause will see if the Clipboard has something; if it has, it will store the data in a string(s), for use later to change the text of labelClip
.timerRefresh_Tick(object sender, EventArgs e)
: In this function, every time the Timer
reaches the interval time (5 sec.), it will execute PasteData
.private void SetToClipboard(object text)
: Here, we will add a text that is in the object test
to the Clipboard, using SetDataObject
.private void buttonSet_Click(object sender, EventArgs e)
: When the user clicks in the buttonSet
button, it will use SetToClipboard
, using the text that is in testClip
as the variable.