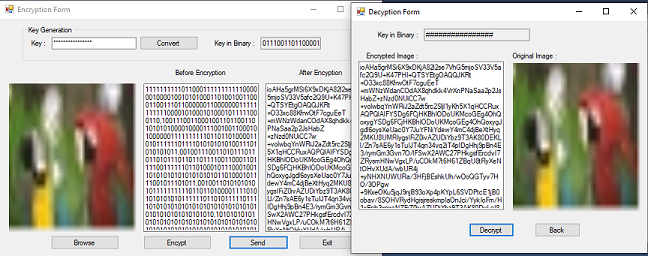
Introduction
This article explains two things:
- The working of
TripleDESCryptoServiceProvider()
cryptographic function - The conversion of Image to Byte and vice versa
Cryptography is a Greek word which means hidden. Cryptography in computers means secure and protected communication between the authorized ones. It was initially used by the army to exchange confidential information and earlier, it was used by kings to send secret messages. Modern cryptography aims at achieving the following goals:
- Confidentiality
- Integrity
- Authentication
- Non-repudiation
- Availability
There are three types of cryptography:
- Symmetric Cryptography
Single key/ Secret key/ Private Key - same key and algorithm used to encrypt/decrypt
- Asymmetric Cryptography
Public and Private keys/ two keys one for encryption and other for decryption in any order used
- Hybrid Cryptography
Combination of both above, adopts good qualities of both and discards weaknesses of both
Now we will understand symmetric cryptography by an example of encoding an image to cipher text using 128 bit key and sending to other user and then decoding using same key.
Understanding the Code
First, we generate a key using any characters or numbers or symbols, validate it as 128 bit and convert to binary.
if (keyBoxTxt.Text.Length == 16)
{
keyBinTxt.Text = StringToBinary(keyBoxTxt.Text);
uploadImg.Enabled = true;
}
else
MessageBox.Show("Key size is not appropriate, Please enter 16 characters!");
Length = 16 means 16 characters or symbols each of 8 bits so making 128 bits in total.
Here, a StringToBinary()
function is used which converts a string to binary.
public static string StringToBinary(string data)
{
StringBuilder sb = new StringBuilder();
foreach (char c in data.ToCharArray())
{
sb.Append(Convert.ToString(c, 2).PadLeft(8, '0'));
}
return sb.ToString();
}
Now to open image using browse button:
OpenFileDialog open = new OpenFileDialog();
open.Filter = "Image Files(*.jpg; *.jpeg; *.gif; *.bmp)|*.jpg; *.jpeg; *.gif; *.bmp";
if (open.ShowDialog() == DialogResult.OK)
{
pictureBox1.Image = new Bitmap(open.FileName);
pictureBox1.SizeMode = PictureBoxSizeMode.StretchImage;
imgpath = open.FileName;
encryptBtn.Enabled = true;
}
Reads image from FileDialog
and shows in a picture box.
Now the real working gets started and we start using TripleDESCryptoServiceProvider()
function:
byte[] bytimg = (Byte[])new ImageConverter().ConvertTo(pictureBox1.Image, typeof(Byte[]));
beforeEnc();
TripleDESCryptoServiceProvider tripleDES = new TripleDESCryptoServiceProvider();
tripleDES.KeySize = 128;
tripleDES.Key = UTF8Encoding.UTF8.GetBytes(keyBoxTxt.Text);
tripleDES.Mode = CipherMode.ECB;
tripleDES.Padding = PaddingMode.PKCS7;
ICryptoTransform cTransform = tripleDES.CreateEncryptor();
byte[] resultArray = cTransform.TransformFinalBlock(bytimg, 0, bytimg.Length);
tripleDES.Clear();
afterEncTxt.Text = Convert.ToBase64String(resultArray, 0, resultArray.Length);
Firstly, image is converted to byte using imageconverter
then by using self made function beforeEnc()
the bytes are converted to binary to string and shown in a multiline textbox.
Now a wrapper object for TripleDESCryptoServiceProvider()
function is created as tripleDES
. Then as we defined, our key size should be exactly 128 bits so it is set for tripleDES
too. After converting key into bytes, cipher algorithm is selected ECB.
Electronic Code Book(ECB)
It is an operation mode for creating block cipher, which means the bytes or bits will be encrypted using block cipher. Cipher is the encrypted plain text using any algorithm. There are two types of cipher:
- Stream cipher
- Block cipher
ECB is a block cipher algorithm which will convert the repeated plain text to same repeated cipher text. Means if "a
" means 0011
then every time when "a
" strikes, it will encode it as 0011
. So it's not good for small block sizes, that is why we use 128 bits block.
Public Key Cryptography Standards(PKCS) # 7
These are the de-facto standard formats for public key encryption by RSA Data Security Inc. It deals with Digital Signatures and Certificates. And PKCS #7 encrypts a message using a certificate's PK and decrypted only with that certificate's private key. Some extended features of PKCS #7 includes:
- It can encrypt using multiple certificates at the same time
- Recursive, means a digital envelop is wrapped in a digital envelop and further in another digital envelop and so on
- Time stamps for encrypted message and digital signatures
- Counter signatures and user defined characteristics
Then ICryptoTransform
defines the basic encryption operations and converts the encypted message to byte array and assigns to a textbox to compare the message before and after encryption.
Now encryption is done!
And the turn to Decrypt the message.
As both the Key and Encrypted message are sent together, so here is the code:
byte[] inputArray = Convert.FromBase64String(afterEncTxt.Text);
TripleDESCryptoServiceProvider tripleDES = new TripleDESCryptoServiceProvider();
tripleDES.KeySize = 128;
tripleDES.Key = UTF8Encoding.UTF8.GetBytes(binKeyTxt.Text);
tripleDES.Mode = CipherMode.ECB;
tripleDES.Padding = PaddingMode.PKCS7;
ICryptoTransform cTransform = tripleDES.CreateDecryptor();
byte[] resultArray =
cTransform.TransformFinalBlock(inputArray, 0, inputArray.Length);
tripleDES.Clear();
using (var ms = new MemoryStream(resultArray))
{
pictureBox1.Image = Image.FromStream(ms);
}
The same code is used to decrypt the message and the original images will be generated in bytes[]
. This time, we used ICryptoTransform
to create Decryptor()
. And then, using a memory stream converted bytes array to image.
Just remember one thing that cipher mode and padding mode should always be the same on both sides (Encryption/Decryption).
History
- 24th May, 2019: Initial version