Convert 10112 to base 10.
1 x 20 = 1
1 x 21 = 2
0 x 22 = 0
1 x 23 = 8
1 + 2 + 0 + 8 = 11
Answer: 10112 = 1110
Does this look familiar? I learned this during my college days, but I didn’t understand how can we use it. Until… I code.
static void Main(string[] args)
{
Console.WriteLine((7 | 3).ToString());
Console.WriteLine((7 & 3).ToString());
Console.Read();
}
Try to run this code in Visual Studio, and you will have this:
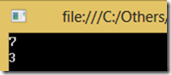
Let’s start the math resolution now.
7 = 1112 or [22 + 21 + 20 = 7] or [4 + 2 + 1 =7]
3 = 112 or [21 + 20 = 3] or [2 + 1 = 3]
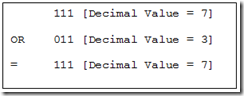
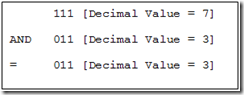
Remembered? logical operator. 1 AND 1 = 1, 1 AND 0 = 0, 1 OR 1 = 1, 1 OR 0 =1…etc.
Let’s start the code now.
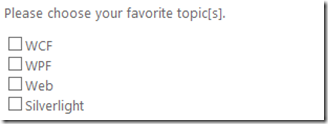
Let’s say, we have a survey requirement. See how we can store multiple selections in a field by using bitwise.
public enum Favorites
{
WCF = 1,
WPF = 2,
Web = 4,
Silverlight = 8,
None = 0
}
First, we create an Enum
class with predefined value for each selection.
<asp:UpdatePanel ID="UpdatePanel1″ runat="server">
<ContentTemplate>
<p>
Please choose your favorite topic[s].
</p>
<asp:CheckBox ID="WCF" runat="server" Text="WCF" />
<br />
<asp:CheckBox ID="WPF" runat="server" Text="WPF" />
<br />
<asp:CheckBox ID="Web" runat="server" Text="Web" />
<br />
<asp:CheckBox ID="Silverlight" runat="server"
Text="Silverlight" />
<p>
<asp:Label ID="Label2″ runat="server" Text="Value"
AssociatedControlID="DecimalValueText_Get"></asp:Label>
<asp:TextBox ID="DecimalValueText_Get" runat="server"></asp:TextBox>
<asp:Button ID="GetValue" runat="server"
Text="Get Value" OnClick="GetValue_Click" />
</p>
<p>
<asp:Label ID="Label1″ runat="server" Text="Value"
AssociatedControlID="DecimalValueText_Set"></asp:Label>
<asp:TextBox ID="DecimalValueText_Set" runat="server"
Text="0″></asp:TextBox>
<asp:Button ID="SetValue" runat="server"
Text="Set Value" OnClick="SetValue_Click" />
</p>
</ContentTemplate>
</asp:UpdatePanel>
private Int32 Retrieve()
{
Int32 result = 0;
result = WCF.Checked ? (result | Favorites.WCF.GetHashCode()) : result;
result = WPF.Checked ? (result | Favorites.WPF.GetHashCode()) : result;
result = Web.Checked ? (result | Favorites.Web.GetHashCode()) : result;
result = Silverlight.Checked ? (result | Favorites.Silverlight.GetHashCode()) : result;
return result;
}
Then, follow by a function to construct an integer value according to the selection.
private void Display()
{
Int32 favorites = int.Parse(DecimalValueText_Set.Text);
WCF.Checked = ((favorites & Favorites.WCF.GetHashCode()) == Favorites.WCF.GetHashCode());
WPF.Checked = ((favorites & Favorites.WPF.GetHashCode()) == Favorites.WPF.GetHashCode());
Web.Checked = ((favorites & Favorites.Web.GetHashCode()) == Favorites.Web.GetHashCode());
Silverlight.Checked =
((favorites & Favorites.Silverlight.GetHashCode()) == Favorites.Silverlight.GetHashCode());
}
To make the solution more complete, we create another function to make the selection according to a defined value.
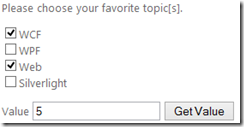
Run the project, now you can select multiple items and click “GetValue
” to get the result.
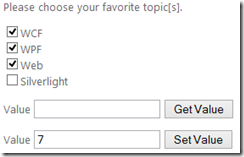
Or, key in a result and let the program make the selection by clicking on “SetValue
” button.
