Introduction
This simple tip is for WPF beginners level. This will help to understand the use of RotateTransform
by which a simple clock can be created. Just changing the Angle
in the RotateTransform
for each line (hours, minute and seconds hand), a simple clock is made.
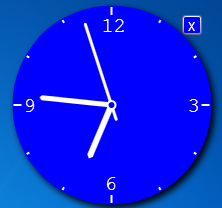
Background
This requires a simple understanding of XAML usage. Also, a basic knowledge in C# is enough as we are not going to add any complex items.
Using the Code
XAML Part
Let's look at the XAML part first. First, Canvas
will be added inside the canvas
, Ellipse
, Lines
, TextBlock
will be added accordingly. Now let us see how the clock is made in 3 easy steps.
Step 1: Adding Clock Like Appearance
First, create a Canvas
with Height = "200"
and Width ="200"
and inside the Canvas
, draw an ellipse, then fill ellipse with Radial Gradient Brush. Note: Grid
background is set as "Black
". Then lines are added for every 5 minutes and these lines are transformed accordingly as shown below (for the "twelve", we do not RenderTransform
as it is straight down).
<Line Name="twelve"
X1="100" Y1="10"
X2="100" Y2="2"
Stroke="White"
StrokeThickness="2"/>
<Line Name="One"
X1="100" Y1="5"
X2="100" Y2="2"
Stroke="White"
StrokeThickness="2">
<Line.RenderTransform>
<RotateTransform Angle="30" CenterX="100" CenterY="100"/>
</Line.RenderTransform>
</Line>
<Line Name="Two"
X1="100" Y1="5"
X2="100" Y2="2"
Stroke="White"
StrokeThickness="2">
<Line.RenderTransform>
<RotateTransform Angle="60" CenterX="100" CenterY="100"/>
</Line.RenderTransform>
</Line>
After adding twelve lines with transformation, now we will add four text blocks to display numbers 3, 6, 9 and 12 in the clock.
Step 2: Adding Hands to Clock
Now it is time for adding hour, minute, second and ellipse at the center. By now, the designer window will show a clock appearance. For all the Hours and Minute hand:
<Line Name="mins"
StrokeThickness="5"
Stroke="Navy" StrokeEndLineCap="Triangle"
StrokeStartLineCap="Round"
X2="100" Y2="30"
X1="100" Y1="100"/>
<Line Name="hrs"
StrokeThickness="6"
Stroke="Navy"
StrokeStartLineCap="Round"
StrokeEndLineCap="Triangle"
X2="100" Y2="45"
X1="100" Y1="100"/>
<Line Name="secs"
StrokeThickness="3"
Stroke="Blue" StrokeEndLineCap="Round"
StrokeStartLineCap="Round"
X2="100" Y2="15"
X1="100" Y1="115"/>
<Ellipse Height="10" Width="10"
Fill="Blue"
StrokeThickness="1" Margin="95,95,0,0"
Stroke="White"/>
C# Part
Step 3: Adding Code to Timer Event
Now it's time to make the seconds, minute and hour hand to transform with the help of System.DateTime
. Timer is added and initiated. Then for each second, it executes the method below. The RotateTransform
helps to rotate the line with the given axis.
RotateTransform rt1 = new RotateTransform(_seconds, 100, 100);
_seconds
is nothing but the angle then followed by CenterX
and CenterY
. As we have canvas with height 200 and width 200, the center point is 100,100. So now, the transformation is done with respect to center.
Likewise for the minute and hour hands.
void timers_Tick(object sender, EventArgs e)
{
int nowSecond = DateTime.Now.Second * 6;
secs.RenderTransform = new RotateTransform(nowSecond, 100, 100);
int nowMinute = DateTime.Now.Minute * 6;
mins.RenderTransform = new RotateTransform(nowMinute, 100, 100);
int nowHour = DateTime.Now.Hour;
if (nowHour > 12)
{
nowHour -= 12;
}
nowHour *= 30;
nowHour += nowMinute / 12;
hrs.RenderTransform = new RotateTransform(nowHour, 100, 100);
}
Points of Interest
It helps how the RotateTransform
can be used to rotate lines both in XAML and C#. In my next post, I will try to add more content so that we could make some cool stuff similar to Windows 7 desktop clock gadget with themes in it.
History
- Source files were added correctly
- Repeated XAML codes were removed
- Hour calculation was wrong and has now been corrected (26th March, 2013)
- Default Window removed, added right click option to set color and Drag enabled