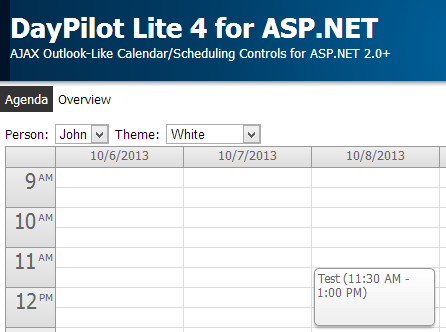
DayPilot Lite 4 (Apache Software License 2.0) brings a new feature: full CSS styling and themes support.
Let's explore it using a simple scheduling project.
Project features:
- Weekly agenda scheduling using an event calendar view
- Scheduling events for multiple resources (people)
- Summary scheduler view with all resources
- Event creating using a modal dialog
- Event editing using a modal dialog
You can download this project in a Visual Studio 2012 solution.
HTML5/JavaScript Event Calendar
New (May 2015): DayPilot Lite for JavaScript (open-source) is now available for download. It includes event calendar for HTML5/JavaScript/jQuery with drag and drop support. AngularJS event calendar plugin is included.

SQL Server Database

We will create a simple SQL Server database with the following schema:
CREATE TABLE [dbo].[Event]
(
[EventId] INT NOT NULL PRIMARY KEY IDENTITY,
[EventName] NVARCHAR(100) NULL,
[EventStart] DATETIME NULL,
[EventEnd] DATETIME NULL,
[ResourceId] INT NOT NULL
);
CREATE TABLE [dbo].[Resource]
(
[ResourceId] INT NOT NULL PRIMARY KEY IDENTITY,
[ResourceName] NVARCHAR(100) NULL
);
There are two tables in the database:
- [Event] table stores events (reservations)
- [Resource] table stores resources (people)
LINQ to SQL

We will access the database (DayPilot.mdf) using LINQ to SQL.
First, we will create the required helper classes by dragging the tables from the Server Explorer to a new DayPilot.dbml file.
Event Calendar (Agenda)

The Default.aspx file will display an event calendar with an agenda for the selected person. It uses DayPilot Calendar ASP.NET control.
Default.aspx
<DayPilot:DayPilotCalendar
runat="server"
ID="DayPilotCalendar1"
CssOnly="true"
CssClassPrefix="calendar_white"
>
</DayPilot:DayPilotCalendar>
Default.aspx.cs
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
InitCalendar();
}
LoadEvents();
}
private void InitCalendar()
{
DayPilotCalendar1.TimeRangeSelectedHandling = DayPilot.Web.Ui.Enums.TimeRangeSelectedHandling.JavaScript;
DayPilotCalendar1.TimeRangeSelectedJavaScript = "create('{0}')";
DayPilotCalendar1.EventClickHandling = DayPilot.Web.Ui.Enums.EventClickHandlingEnum.JavaScript;
DayPilotCalendar1.EventClickJavaScript = "edit('{0}')";
DayPilotCalendar1.StartDate = DayPilot.Utils.Week.FirstDayOfWeek();
DayPilotCalendar1.Days = 7;
}
private void LoadEvents()
{
int resource = Convert.ToInt32(DropDownListResources.SelectedValue);
DayPilotCalendar1.DataSource = from e in db.Events where e.ResourceId == resource select e;
DayPilotCalendar1.DataTextField = "EventName";
DayPilotCalendar1.DataValueField = "EventId";
DayPilotCalendar1.DataStartField = "EventStart";
DayPilotCalendar1.DataEndField = "EventEnd";
DayPilotCalendar1.DataBind();
}
Scheduler

The Overview.aspx page will display a scheduler view with all people displayed at once. It uses DayPilot Scheduler ASP.NET control.
Overview.aspx
<DayPilot:DayPilotScheduler
runat="server"
ID="DayPilotScheduler1"
CssOnly="true"
CssClassPrefix="scheduler_white"
>
</DayPilot:DayPilotScheduler>
Overview.aspx.cs
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
LoadResources();
InitCalendar();
}
LoadEvents();
}
private void InitCalendar()
{
DayPilotScheduler1.TimeRangeSelectedHandling = DayPilot.Web.Ui.Enums.TimeRangeSelectedHandling.JavaScript;
DayPilotScheduler1.TimeRangeSelectedJavaScript = "create('{0}', '{1}')";
DayPilotScheduler1.EventClickHandling = DayPilot.Web.Ui.Enums.EventClickHandlingEnum.JavaScript;
DayPilotScheduler1.EventClickJavaScript = "edit('{0}')";
DayPilotScheduler1.CellWidth = 40;
DayPilotScheduler1.EventHeight = 25;
}
private void LoadEvents()
{
DayPilotScheduler1.DataSource = from e in db.Events select e;
DayPilotScheduler1.DataTextField = "EventName";
DayPilotScheduler1.DataValueField = "EventId";
DayPilotScheduler1.DataStartField = "EventStart";
DayPilotScheduler1.DataEndField = "EventEnd";
DayPilotScheduler1.DataResourceField = "ResourceId";
DayPilotScheduler1.DataBind();
}
private void LoadResources()
{
var res = from r in db.Resources select r;
foreach (Resource r in res)
{
DayPilotScheduler1.Resources.Add(r.ResourceName, r.ResourceId.ToString());
}
}
Event Calendar CSS Themes
This project includes three event calendar CSS themes. You can create your own theme in the online DayPilot Theme Designer.
White CSS Theme

Green CSS Theme

Transparent CSS Theme

Scheduler CSS Themes
This project includes three scheduler CSS themes. You can create your own theme in the online DayPilot Theme Designer.
White CSS Theme

Green CSS Theme

Transparent CSS Theme

History
See Also