Introduction
The ASP.NET Routing Framework is at the core of every ASP.NET MVC request and it is simply a pattern-matching system. At the application start-up, it registers one or more patterns with route table of the framework to tell it what to do with the pattern matching requests. For routing, the main purpose is to map URLs to a particular action in a specific controller.
When the routing engine receives any request, it first matches the request URL with the patterns already registered with it. If it finds any matching pattern in route table, it forwards the request to the appropriate handler for the request. If the request URL does not match with any of the registered route patterns, the routing engine returns a 404 HTTP status code.
Where to Configure
How to Configure
ASP.NET MVC routes are responsible for determining which controller methods need to be executed for the requested URL. The properties for this to be configured are:
- Unique Name: A name unique to a specific route
- URL Pattern: A simple URL pattern syntax
- Defaults: An optional set of default values for each segment defined in URL pattern
- Constraints: A set of constraints to more narrowing the URL pattern to match more exactly
The RegisterRoute
method in RouteConfig.cs file is as follows:
public class RouteConfig
{
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.MapRoute(
name: "Default",
url: "{controller}/{action}/{id}",
defaults: new { controller = "Login", action = "Index",
id = UrlParameter.Optional }
);
}
}
Then in Global.asax.cs file, call this on application start event handler so that this will be registered on application start itself:
protected void Application_Start()
{
RouteConfig.RegisterRoutes(RouteTable.Routes);
}
How Routing Engine Works
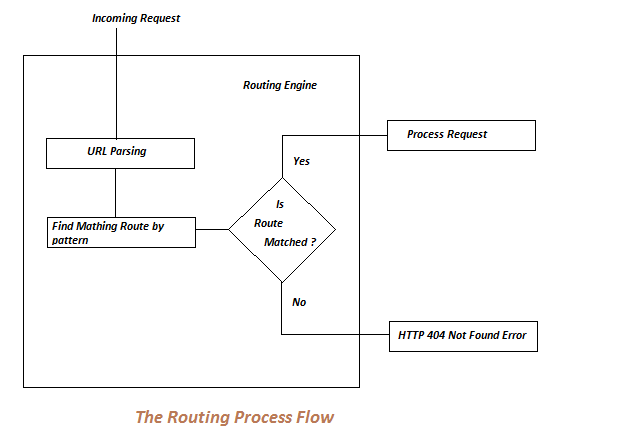
Route Matching

Hope this will give a clear understanding on what is routing and how routing engine works...
CodeProject