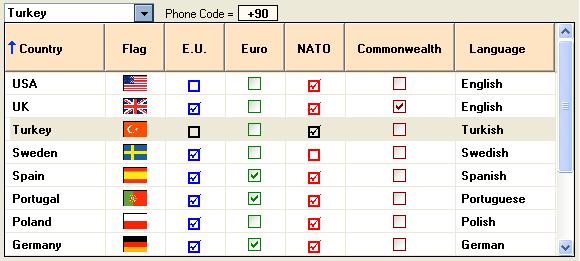
Introduction
This component, written in VB.NET, was first produced in 2008. But recently, I decided to introduce some new features, especially in the ListView
part. Some reasons are listed below:
- The need to present
Images
and good 'CheckBox
es', not just those resulting from the use of characters from the Wingdings font. - The need to automatically adjust the height of the subcomponents, given the use of different fonts and font sizes.
- The need to 'correctly' sort
Text
, Numeric
and Date(Time)
Columns. - A fact which irritated everyone: with Visual Studio 2008 on Windows XP, increasing the font size did not change the height of the
ListView
header. Annoying... So, I also decided to impose the Header Height.
Thus, the control became, not only 'one more ComboBox
', but an enhanced, flexible, powerful and easy-to-use component.
- It works with various types of data:
Text
, Number
s, Date(Time)
, Image
s and Boolean
s Image
s can be sent as (real) images or by path reference Boolean
s are shown as 'checkbox
es', but in 2 ways: by picture (better aspect, scalable), or by characters from Wingdings font (higher performance) - Has a built-in Row Counter
- Rows can be filtered, in order to reduce the list, by typing characters in the
TextBox
- The user can control the List Header Height and Colors
- The user can control the List Rows Height
- The user can control each Column's Fore & Back Colors
- The user can control the
Size
and SizeMode
of Images and 'picture checkboxes' - Columns can be
Resized
, Reordered
and Sorted
(ascending/descending, with Arrow indicator) Number
s and Date
s are 'effectively' ordered, regardless of the Format in which they are displayed

Of course, the more images are added, the worse the performance...
Programming
Also, the code is as simple as possible, and programmers can find some useful code snippets, related to:
- Expandable properties
- Fading effects
- Color change
- Embedded controls handling
HeaderControl
subclassing (NativeWindow
) HDM_LAYOUT
capture - Header Height control
- Header Sort Arrows
- Columns
Text
, Date
& Numeric
effective sorting
See Documentation and Examples
First of all, download the .ZIP file.
The .ZIP file includes ExCB_Documentation.PDF, which fully describes the component.
The demo forms present several examples, techniques and tips to manipulate data.
Last Updates
- Addendum to ExCB_Documentation.PDF:
Filtering - To reset filtering, selecting all Rows, just drop-down the List clicking the mouse right-button. - When starting the provided solution, if you receive the message 'A project with an Output Type of Class Library cannot be started directly', then:
Go to the Solution Explorer, select ExCB_Test
Project, right-click mouse button and click Set as StartUp Project. - Addendum to ExCB_Documentation.PDF: Take note of another Event:
Event
DropDownChanged - Occurs when the List is Opened/Closed.
Syntax:
DropDownChanged(IsDroppedDown as Boolean)
Remarks:
- IsDroppedDown
= True
- The List was Opened
- IsDroppedDown
= False
- The List was Closed - To avoid an error, in a very specific circumstance, please,
in Class ExCB.vb
, Property SelectedIndex()
, replace the line:
MyLV1.Items(value).Selected = True
with:
Try
MyLV1.Items(value).Selected = True
Catch
End Try
- In response to questions posed by L.Botello:
- ...it seems that, in certain circumstances, when font size is increased, the list is slightly truncated (bottom). Isn't?
Yes, it's a bug. To fix it, please:
in ExCB.vb
, Region "TextBox & Button code
", Sub Button1_MouseDown
, replace the line:
Dim LX As Integer = Sizes_.ListHeader_Height + 1 + MX * (_ListRowHeight + 1)
with:
Dim LX As Integer = Math.Max(Sizes.ListHeader_Height, _
TextRenderer.MeasureText(" ", Me.Font).Height + 4) + _
MX * (_ListRowHeight + 1)
- Did you ever have considered the possibility of growing/shrink the list (regardless of
MaxRowsDisp
property value) when the form is resized?
Thank you for the suggestion, that will improve control's features.
It will be an option. Please, do the following:
in Class ExCB.vb
, Region "Public Methods
", insert the following Sub:
Public Sub AdjustListHeight()
Try
Dim Parent_csH As Integer = Me.ParentForm.ClientSize.Height
Dim HeaderH = Math.Max(Sizes.ListHeader_Height, TextRenderer.MeasureText(" ", Me.Font).Height + 4)
Dim RestH As Integer = Parent_csH - Me.Top - MyLV1.Top - HeaderH
_MaxDsp = RestH \ (_ListRowHeight + 1)
Dim MinRows As Integer = Math.Min(_MaxDsp, MyLV1.Items.Count + 1)
If MyLV1.Visible Then
MyLV1.Height = HeaderH + MinRows * (_ListRowHeight + 1)
Me.Height = _LblBoxH + MyLV1.Height
Else
_HideLV()
End If
Catch
End Try
End Sub
Then, in your Form, insert this code and invoke the Method just for the Control instance(s) you want the feature active:
Private Sub Me_Sizes(sender As Object, e As EventArgs) Handles Me.Resize, Me.SizeChanged
ExCB1.AdjustListHeight()
End Sub
Anyway, define MaxRowsDisp
value for the first display (when it occurs before the first resize...).
- Made available for download a new project (source and demo), for VS2012, which is the 'original' plus all the above updates (including Method
AdjustListHeight
), plus a new Property AutoComplete
, which gives the Control AutoComplete-like capabilities: whenever a key is pressed, the List drops down, showing only the rows that matches the typed string. The match applies to any subitem (Column). Setting the Property AutoComplete
to True
implies Property Filterable
to be also set to True
. Before running ExCB_Test
project, don't forget to Set
it as StartUp Project
.
Using the Code
The component is provided in 2 versions: Visual Studio 2008 and Visual Studio 2012.
All features are available in both versions, but version 2012 is less limited and easier to use.
The component DLL is located at ...\ExCB\VS20xx\ExCB\bin\Release\ExCB.dll.
- Add it to your Toolbox, right-clicking the Toolbox area -> Choose Items… -> Browse -> select the DLL
- In the Solution Explorer, select your Project, open My Project -> References -> Add… -> Browse -> select the DLL
- Drag the component from the Toolbox to your Form.
Brief Description of the Component's Own Properties, Event and Methods
Browsable Properties
BorderStyle
The BorderStyle
which applies to the TextBox
subcomponent Colors
(Expandable Property)
HeadBackColor
- BackColor
of the ListView
Header HeadForeColor
- ForeColor
of the ListView
Header ListBackColor
- BackColor
of the ListView
Items ListForeColor
- ForeColor
of the ListView
Items TBoxBackColor
- BackColor
of TextBox
element TBoxForeColor
- ForeColor
of TextBox
element
CounterLoc
- The position and alignment of the built-in Row Counter
, relative to the TextBox
DisplayColumn
- The Index
of the SubItem
(Column
) to display in the TextBox
, when selecting a Row
in the List
Filterable
- Indicates if the ListView
Rows can/cannot be filtered Font
- Defines the Font Name, Size and Styles for ALL the subcomponents (ListView
, TextBox
and Counter
) GridLines
- Displays grid lines around Items
and SubItems
, in the ListView
MaxRowsDisp
- Maximum number of Rows to display, when the List
is dropped-down MinimunWidth
- Minimum Width of the component. Changes when the component is resized Read_Only
- When True
, the component cannot be dropped-down / changed, but shows the current selection Reordable
- Indicates if the ListView
Columns can/cannot be reordered Resizable
- Indicates if the ListView
Columns can/cannot be resized Sizes
- (Expandable Property)
Image_Height
- Height of the Images sent to the List
Image_Width
- Width of the Images sent to the List
ListHeader_Height
- Height of the ListView
Header ListRow_Height
- Height of the ListView
Rows YesNo_Height
- Height (and Width) of the "checkboxes"
Sortable
Indicates if the ListView
Columns can/cannot be sorted
Non-browsable Properties
Count
- Get
s the number of Rows in the List
SelectedIndex
- Get
s / Set
s the Index
of the Row
currently selected Text
- Get
s / Set
s the Text
from/to the TextBox
part of the component
Events
ItemSelected
- Occurs when a Row is Selected / programmatically Unselected DropDownChanged
- Occurs when the List is Opened/Closed
Methods
AddColumn
- Creates a new Column (with 1 required and 13 optional parameters)
Syntax:
AddColumn(HeaderText As String,
[Width As Integer = 80],
[Align As System.Windows.Forms.HorizontalAlignment = HorizontalAlignment.Left],
[DataType As ExCB.ExCB.DataType = ExCB.ExCB.DataType._Text],
[Format As String = Nothing],
[ForeColor As System.Drawing.Color = Nothing],
[BackColor As System.Drawing.Color = Nothing],
[ImgWidth As Integer = 0],
[ImgHeight As Integer = 0],
[SizeMode As ExCB.ExCB.SizeMode = ExCB.ExCB.SizeMode._Stretch],
[Resizable As Boolean = True],
[Reordable As Boolean = True],
[Sortable As Boolean = True],
[ArrowOnRight As Boolean = False])
AddRow
- Adds a Row (Item
) to the ListView
(sends a String
or Object
Array) ARGB
- Converts a Color
to its Integer
value Clear
- Deletes all Row
s of the List
(including Embedded Controls), optionally all Columns RowSelect
- Selects a Row
, giving a Value
and a Column Index
, optionally returning another subitem GetImage
- Retrieves the Image
at the specified Index
within the Component
's array
History
03.Jun.2014 - First posted
05.Jun.2014 - Added Last Updates 1. and 2. to See Documentation and Examples
11.Jun.2014 - Added Last Updates 3. and 4. to See Documentation and Examples
05.Dec.2014 - Added Last Updates 5. to See Documentation and Examples
21.Mar.2016 - Added Last Updates 6. to See Documentation and Examples